Say we are storing item ids inside the element container id attributes and we simply want to extract the numbers from the id attribute string. You can get the number from any elements id tag by using a simple JavaScript regular expression replace statement.
$(this).attr('id').replace(/[^d]/g, '');
Before: container1
After: 1
Say the id tag contains both letters and numbers and we are interested in getting only the number from the div container id. This could be used for something like when you are trying to grab an id from a parent container element:
//elements...
//a button...with a bound click event
//elements...
//a button...with a bound click event
//elements...
//a button...with a bound click event
So when we click any element we can grab it’s container id to use.
//gets the container id number only from element
function getIdNum(elem)
{
if (elem.attr('id'))
{
return elem.attr('id').replace(/[^d]/g, '');
}
else
{
return elem.parents('.widget').attr('id').replace(/[^d]/g, '');
}
}
//example call
var containerId = getIdNum($('some button'));
Frequently Asked Questions (FAQs) about jQuery Numbers and Element ID
How can I select an element by ID using jQuery?
To select an element by ID using jQuery, you use the “#” symbol followed by the ID of the element. For example, if you have an element with the ID “myElement”, you would select it in jQuery like this: $(“#myElement”). This will return a jQuery object that you can then use to manipulate the element.
Can I use numbers in an element’s ID?
Yes, you can use numbers in an element’s ID. However, the ID must not start with a number. It should start with a letter for compatibility with CSS and JavaScript. For example, “id1” is a valid ID, but “1id” is not.
How can I get the value of an element’s ID with jQuery?
You can get the value of an element’s ID using the attr() method in jQuery. For example, if you have an element with the ID “myElement”, you can get its ID like this: $(“#myElement”).attr(“id”). This will return the string “myElement”.
What is the difference between ID and class in jQuery?
In jQuery, an ID is a unique identifier for an element, while a class can be used to identify multiple elements. You can select an element by its ID using the “#” symbol, and by its class using the “.” symbol. For example, $(“#myElement”) selects the element with the ID “myElement”, while $(“.myClass”) selects all elements with the class “myClass”.
Can I use special characters in an element’s ID?
Yes, you can use special characters in an element’s ID, but they must be escaped with two backslashes in jQuery. For example, if you have an element with the ID “my:Element”, you would select it in jQuery like this: $(“#my\:Element”).
How can I change the ID of an element with jQuery?
You can change the ID of an element using the attr() method in jQuery. For example, if you want to change the ID of an element from “oldID” to “newID”, you would do it like this: $(“#oldID”).attr(“id”, “newID”).
How can I select multiple elements with the same ID in jQuery?
In HTML, an ID should be unique within a page. Therefore, you should not have multiple elements with the same ID. If you do, jQuery will only select the first one. If you want to select multiple elements, you should use a class instead.
Can I use jQuery to select elements by their attributes?
Yes, you can use jQuery to select elements by their attributes. For example, to select all elements with a certain data attribute, you would do it like this: $(“[data-myAttribute]”). This will select all elements that have the “data-myAttribute” attribute.
How can I select an element by its ID in pure JavaScript?
In pure JavaScript, you can select an element by its ID using the document.getElementById() method. For example, if you have an element with the ID “myElement”, you would select it like this: document.getElementById(“myElement”).
Can I use jQuery to select elements by their tag name?
Yes, you can use jQuery to select elements by their tag name. For example, to select all div elements, you would do it like this: $(“div”). This will select all div elements on the page.
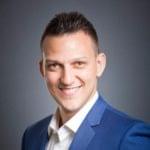
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.