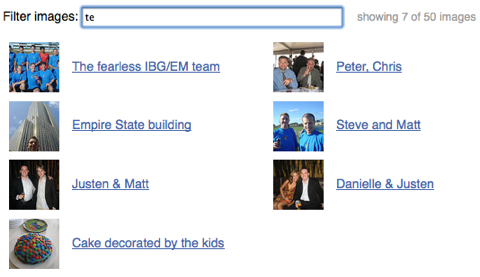
jQuery Code for Live Image Search
$("#filter").keyup(function () {
var filter = $(this).val(), count = 0;
$(".filtered:first li").each(function () {
if ($(this).text().search(new RegExp(filter, "i")) < 0) {
$(this).addClass("hidden");
} else {
$(this).removeClass("hidden");
count++;
}
});
$("#filter-count").text(count);
});
Demo
Source
It uses the Live Search with QuickSilver Style jQuery plugin based upon the QuickSilver string ranking algorithm in JavaScript.
QuickSilver Search Demo
Quicksilver Live Search Plugin
(function($) {
var self = null;
$.fn.liveUpdate = function(list) {
return this.each(function() {
new $.liveUpdate(this, list);
});
};
$.liveUpdate = function (e, list) {
this.field = $(e);
this.list = $('#' + list);
if (this.list.length > 0) {
this.init();
}
};
$.liveUpdate.prototype = {
init: function() {
var self = this;
this.setupCache();
this.field.parents('form').submit(function() { return false; });
this.field.keyup(function() { self.filter(); });
self.filter();
},
filter: function() {
if ($.trim(this.field.val()) == '') { this.list.children('li').show(); return; }
this.displayResults(this.getScores(this.field.val().toLowerCase()));
},
setupCache: function() {
var self = this;
this.cache = [];
this.rows = [];
this.list.children('li').each(function() {
self.cache.push(this.innerHTML.toLowerCase());
self.rows.push($(this));
});
this.cache_length = this.cache.length;
},
displayResults: function(scores) {
var self = this;
this.list.children('li').hide();
$.each(scores, function(i, score) { self.rows[score[1]].show(); });
},
getScores: function(term) {
var scores = [];
for (var i=0; i < this.cache_length; i++) {
var score = this.cache[i].score(term);
if (score > 0) { scores.push([score, i]); }
}
return scores.sort(function(a, b) { return b[0] - a[0]; });
}
}
})(jQuery);
Full Code for the Image Search
/*
* jQuery Filter Demo
* Matt Ryall
* http://www.mattryall.net/blog/2008/07/jquery-filter-demo
*
* Licensed under Creative Commons Attribution 3.0.
* http://creativecommons.org/licenses/by/3.0/
*/
jQuery(function ($) {
var thumbnailUrl = "http://farm{farm-id}.static.flickr.com/{server-id}/{id}_{secret}_s.jpg";
var linkUrl = "http://www.flickr.com/photos/mjryall/{id}/";
$.getJSON("/flickr-photos.cgi?count=50", function (data) {
var photos = data.photos.photo;
var list = $("").attr("src", url)
.attr("title", photo.title).attr("alt", "A photo on Flickr");
var href = linkUrl.replace("{id}", photo.id);
var link = $("").attr("href", href).append(img);
var caption = $("").attr("href", href)
.text(photo.title).addClass("caption");
var div = $("").append(link).append(caption);
$(list).append($("
").append(div));
});
$("#flickr-photos .loading").remove();
$("#flickr-photos").append(list);
})
$("#filter").keyup(function () {
var filter = $(this).val(), count = 0;
$(".filtered:first li").each(function () {
if ($(this).text().search(new RegExp(filter, "i")) < 0) {
$(this).addClass("hidden");
} else {
$(this).removeClass("hidden");
count++;
}
});
$("#filter-count").text(count);
});
});
Frequently Asked Questions (FAQs) about jQuery Image Filtering
How can I use jQuery to filter images based on their attributes?
jQuery provides a powerful method called .filter()
that can be used to filter images based on their attributes. For instance, if you want to select all images with a specific alt
attribute, you can use the following code:$('img').filter(function() {
return $(this).attr('alt') == 'your desired alt attribute';
});
This code will return all images that have the specified alt
attribute. You can replace 'your desired alt attribute'
with the actual attribute you want to filter by.
Can I use jQuery to filter images based on their dimensions?
Yes, you can use jQuery to filter images based on their dimensions. The .filter()
method can be used in combination with the .width()
and .height()
methods to achieve this. Here’s an example:$('img').filter(function() {
return $(this).width() > 500 && $(this).height() > 500;
});
This code will select all images that have a width and height greater than 500 pixels.
How can I use jQuery to filter images based on their visibility?
jQuery provides several selectors that can be used to filter elements based on their visibility. For instance, the :visible
selector can be used to select all visible elements, while the :hidden
selector can be used to select all hidden elements. Here’s how you can use these selectors to filter images:$('img:visible'); // Selects all visible images
$('img:hidden'); // Selects all hidden images
Can I use jQuery to filter images based on their source URL?
Yes, you can use jQuery to filter images based on their source URL. The .filter()
method can be used in combination with the .attr()
method to achieve this. Here’s an example:$('img').filter(function() {
return $(this).attr('src') == 'your desired source URL';
});
This code will select all images that have the specified source URL. You can replace 'your desired source URL'
with the actual URL you want to filter by.
How can I use jQuery to filter images based on their position in the DOM?
jQuery provides several methods that can be used to filter elements based on their position in the DOM. For instance, the :first
selector can be used to select the first element, while the :last
selector can be used to select the last element. Here’s how you can use these selectors to filter images:$('img:first'); // Selects the first image
$('img:last'); // Selects the last image
Can I use jQuery to filter images based on their CSS properties?
Yes, you can use jQuery to filter images based on their CSS properties. The .filter()
method can be used in combination with the .css()
method to achieve this. Here’s an example:$('img').filter(function() {
return $(this).css('border') == '1px solid black';
});
This code will select all images that have a 1px solid black border.
How can I use jQuery to filter images based on their data attributes?
jQuery provides a method called .data()
that can be used to access data attributes. You can use this method in combination with the .filter()
method to filter images based on their data attributes. Here’s an example:$('img').filter(function() {
return $(this).data('your desired data attribute') == 'your desired value';
});
This code will select all images that have the specified data attribute with the specified value.
Can I use jQuery to filter images based on their parent element?
Yes, you can use jQuery to filter images based on their parent element. The .parent()
method can be used in combination with the .filter()
method to achieve this. Here’s an example:$('img').filter(function() {
return $(this).parent().is('your desired parent element');
});
This code will select all images that are children of the specified parent element.
How can I use jQuery to filter images based on their index?
jQuery provides a method called .eq()
that can be used to select an element based on its index. You can use this method to filter images based on their index. Here’s an example:$('img').eq(your desired index);
This code will select the image at the specified index.
Can I use jQuery to filter images based on their alt text?
Yes, you can use jQuery to filter images based on their alt text. The .filter()
method can be used in combination with the .attr()
method to achieve this. Here’s an example:$('img').filter(function() {
return $(this).attr('alt') == 'your desired alt text';
});
This code will select all images that have the specified alt text.
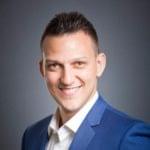
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.