How to keep div on screen (at the bottom or top) using jQuery. This can be achieved with basic CSS but if this fails there is some jQuery/JavaScript you can use to potentially fix the problem of keeping a div on the bottom of the screen even when scrolling.
.bottom { position:fixed; position:absolute; right:0; bottom:0; padding:0; margin:0; }
/*IE FIX*/
.fixie {
left: expression( ( - jsrp_related.offsetWidth + ( document.documentElement.clientWidth ? document.documentElement.clientWidth : document.body.clientWidth ) + ( ignoreMe2 = document.documentElement.scrollLeft ? document.documentElement.scrollLeft : document.body.scrollLeft ) ) + 'px' );
top: expression( ( - jsrp_related.offsetHeight + ( document.documentElement.clientHeight ? document.documentElement.clientHeight : document.body.clientHeight ) + ( ignoreMe = document.documentElement.scrollTop ? document.documentElement.scrollTop : document.body.scrollTop ) ) + 'px' );
}
//note you MUST set a height property for it to work correctly
if ( $.browser.msie ) {
div.css({position: "absolute", width: jslide_width, right: 0, height: 100});
div.addClass('fixie');
} else {
div.css({position: "fixed", width: jslide_width, right: 0, height: 100});
}
Further Techniques
There is also a jQuery plugin which can keep any element on the sidebar in view.Frequently Asked Questions (FAQs) about jQuery and Div Bottom Screen
How can I keep a div at the bottom of the screen when scrolling using jQuery?
To keep a div at the bottom of the screen when scrolling, you can use the CSS property ‘position: fixed’. This property allows the div to stay in a specific position even when the page is scrolled. Here’s a simple example:$("#yourDiv").css("position", "fixed");
$("#yourDiv").css("bottom", "0");
In this code, ‘#yourDiv’ is the id of your div. This will keep your div at the bottom of the screen when scrolling.
How can I find the position of the bottom of a div with jQuery?
To find the position of the bottom of a div, you can use the jQuery ‘offset()’ method combined with the ‘height()’ method. Here’s how you can do it:var bottom = $("#yourDiv").offset().top + $("#yourDiv").height();
In this code, ‘#yourDiv’ is the id of your div. The ‘offset().top’ method returns the top position (in pixels) relative to the document, and the ‘height()’ method returns the height of the div. Adding these two values gives the position of the bottom of the div.
Why doesn’t ‘position: absolute; bottom: 0;’ update on scroll?
The CSS property ‘position: absolute’ positions an element relative to the nearest positioned ancestor. However, if an absolute positioned element has no positioned ancestors, it uses the document body, and moves along with page scrolling. So, ‘bottom: 0’ will not update on scroll. If you want the element to stay at the bottom of the screen when scrolling, use ‘position: fixed’ instead.
How can I get the bottom position of a div with jQuery in relation to the viewport?
To get the bottom position of a div in relation to the viewport, you can subtract the div’s offset from the window’s scroll position. Here’s how you can do it:var bottom = $(window).scrollTop() + $(window).height() - $("#yourDiv").offset().top;
In this code, ‘#yourDiv’ is the id of your div. The ‘scrollTop()’ method returns the vertical scrollbar position for the window, and the ‘height()’ method returns the height of the window. Subtracting the div’s top offset from this value gives the bottom position of the div in relation to the viewport.
How can I make a div stick to the bottom of another div using jQuery?
To make a div stick to the bottom of another div, you can use the jQuery ‘append()’ method. This method inserts specified content at the end of the selected elements. Here’s how you can do it:$("#parentDiv").append($("#childDiv"));
In this code, ‘#parentDiv’ is the id of the parent div and ‘#childDiv’ is the id of the child div. This will make the child div stick to the bottom of the parent div.
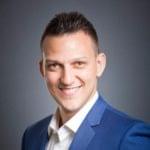
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.