Key Takeaways
- jQuery can be effectively used to capture the vertical scroll percentage of a window, triggering events at specified scroll percentages. However, it has been observed that Firefox 8 never reaches 100% scroll, unlike Chrome 12.
- A script is provided to lock the footer on scroll, showing it when the window scrolls down beyond a certain point and hiding it when scrolling up beyond a different point. This can be useful for maintaining consistent navigation or displaying key information.
- The author answers several FAQs about using jQuery to capture vertical scroll percentage, including how to display this percentage in the title bar, use it in a progress bar, and capture it in specific elements or without using jQuery. Practical code snippets are provided for each scenario.
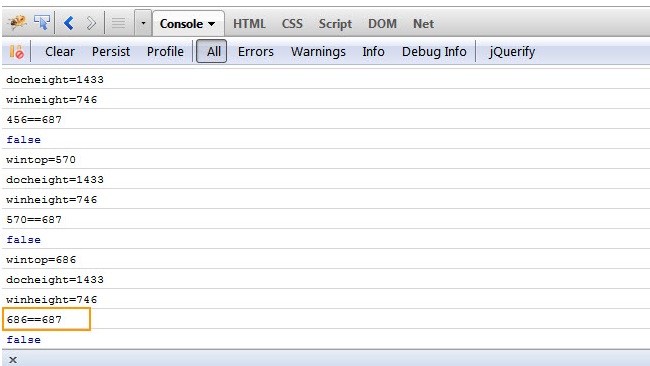
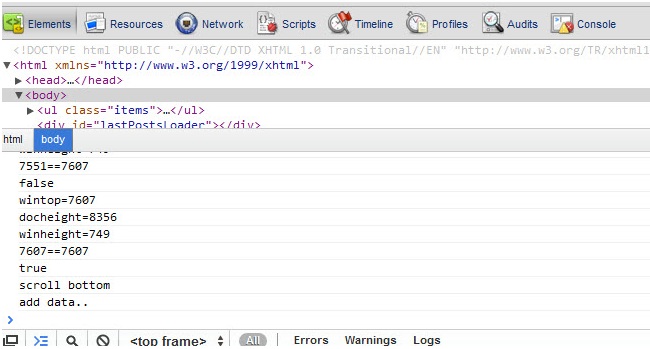
//never reaches bottom
$(window).scroll(function(){
var wintop = $(window).scrollTop(), docheight = $(document).height(), winheight = $(window).height();
console.log('wintop='+wintop);
console.log('docheight='+docheight);
console.log('winheight='+winheight);
console.log(wintop+'=='+(docheight-winheight));
console.log(wintop==(docheight-winheight));
if ($(window).scrollTop() == $(document).height() - $(window).height()) {
console.log('scroll bottom');
}
});
Detect % of vertical scroll using jQuery
This little script will fire off code at any given percentage, currently set to 95% of the window view.//capture scroll any percentage
$(window).scroll(function(){
var wintop = $(window).scrollTop(), docheight = $(document).height(), winheight = $(window).height();
var scrolltrigger = 0.95;
console.log('wintop='+wintop);
console.log('docheight='+docheight);
console.log('winheight='+winheight);
console.log(wintop+'=='+(docheight-winheight));
console.log(wintop==(docheight-winheight));
console.log('%scrolled='+(wintop/(docheight-winheight))*100);
if ((wintop/(docheight-winheight)) > scrolltrigger) {
console.log('scroll bottom');
lastAddedLiveFunc();
}
});
Now we can see, Firefox 8 fires triggers the event.
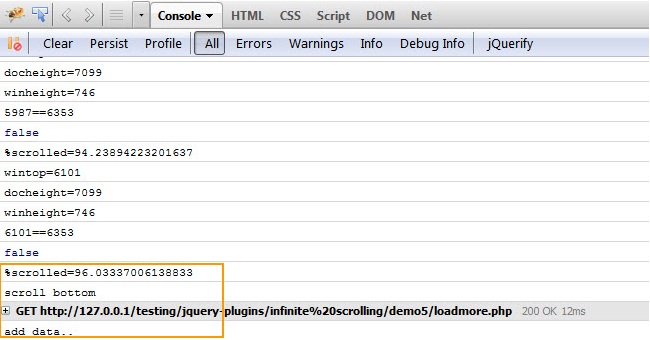
Lock Footer on scroll example
JS Code.//hide/show footer locked when page scrolled up/down
$(window).bind('scroll', function(e)
{
var wintop = $(window).scrollTop(), docheight = $(document).height(), winheight = $(window).height(),
showTrigger = '700', //700 down it will show
hideTrigger = '200'; //200 up it will hide
//show on scroll down
//hide on scroll up
if (wintop > showTrigger)
{
$('#footer').addClass('fixed').fadeIn();
}
else if (wintop < hideTrigger)
{
$('#footer').fadeOut().removeClass('fixed');
}
});
CSS code.
#footer.fixed {
position: fixed;
bottom: 0;
width: 100%;
}
Frequently Asked Questions (FAQs) about jQuery Capture Vertical Scroll Percentage
How can I use jQuery to capture the vertical scroll percentage in different browsers?
jQuery is a cross-platform JavaScript library that simplifies the client-side scripting of HTML. It is designed to make it easier to navigate a document, create animations, handle events, and develop Ajax applications. To capture the vertical scroll percentage in different browsers using jQuery, you need to calculate the scroll position relative to the document’s height. This can be achieved by dividing the scroll top position by the document’s height minus the window’s height. The result is then multiplied by 100 to get the percentage. Here is a simple code snippet:$(window).scroll(function() {
var s = $(window).scrollTop(),
d = $(document).height(),
c = $(window).height();
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
});
How can I display the scroll percentage in the browser’s title bar?
Displaying the scroll percentage in the browser’s title bar can be achieved by setting the document’s title to the calculated scroll percentage. This can be done within the scroll event handler. Here is a code snippet that demonstrates this:$(window).scroll(function() {
var s = $(window).scrollTop(),
d = $(document).height(),
c = $(window).height();
var scrollPercent = (s / (d - c)) * 100;
document.title = "Scroll: " + Math.round(scrollPercent) + "%";
});
How can I get the current percentage of scroll in an element, not the document?
To get the current percentage of scroll in a specific element, you need to calculate the scroll position relative to the element’s height. This can be achieved by dividing the scroll top position by the element’s scroll height minus the element’s height. The result is then multiplied by 100 to get the percentage. Here is a simple code snippet:$('#element').scroll(function() {
var s = $(this).scrollTop(),
d = $(this)[0].scrollHeight,
c = $(this).height();
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
});
How can I use the scroll percentage in a progress bar?
You can use the scroll percentage to update a progress bar by setting the progress bar’s value or width to the calculated scroll percentage. Here is a code snippet that demonstrates this:$(window).scroll(function() {
var s = $(window).scrollTop(),
d = $(document).height(),
c = $(window).height();
var scrollPercent = (s / (d - c)) * 100;
$('#progress-bar').width(scrollPercent + '%');
});
How can I capture the vertical scroll percentage without jQuery?
If you prefer not to use jQuery, you can capture the vertical scroll percentage using pure JavaScript. This can be achieved by using the window
object’s scrollY
, innerHeight
, and documentElement.scrollHeight
properties. Here is a simple code snippet:window.onscroll = function() {
var s = window.scrollY,
d = document.documentElement.scrollHeight,
c = window.innerHeight;
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
};
How can I throttle the scroll event to improve performance?
Throttling the scroll event can help to improve performance by limiting the number of times the scroll event handler is called. This can be achieved by using a throttle function that only allows the scroll event handler to be called once every specified number of milliseconds. Here is a simple throttle function and how to use it with the scroll event:function throttle(func, limit) {
var lastFunc, lastRan;
return function() {
var context = this, args = arguments;
if (!lastRan) {
func.apply(context, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(function() {
if ((Date.now() - lastRan) >= limit) {
func.apply(context, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
};
}
$(window).scroll(throttle(function() {
var s = $(window).scrollTop(),
d = $(document).height(),
c = $(window).height();
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
}, 250));
How can I capture the horizontal scroll percentage?
Capturing the horizontal scroll percentage is similar to capturing the vertical scroll percentage. The main difference is that you need to use the scroll left position and the document’s width instead of the scroll top position and the document’s height. Here is a simple code snippet:$(window).scroll(function() {
var s = $(window).scrollLeft(),
d = $(document).width(),
c = $(window).width();
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
});
How can I animate the scroll to a specific percentage?
Animating the scroll to a specific percentage can be achieved by using jQuery’s animate
function. You need to calculate the scroll top position that corresponds to the desired scroll percentage and then animate the scroll to that position. Here is a code snippet that demonstrates this:var scrollPercent = 50; // desired scroll percentage
var scrollTop = ($(document).height() - $(window).height()) * (scrollPercent / 100);
$('html, body').animate({ scrollTop: scrollTop }, 1000);
How can I capture the scroll percentage of an iframe?
Capturing the scroll percentage of an iframe can be a bit tricky due to the same-origin policy. However, if the iframe’s content comes from the same origin as the parent document, you can access the iframe’s content and calculate the scroll percentage in a similar way as for the document. Here is a simple code snippet:$('#iframe').load(function() {
$(this).contents().scroll(function() {
var s = $(this).scrollTop(),
d = $(this)[0].scrollHeight,
c = $(this).height();
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
});
});
How can I capture the scroll percentage of a div with overflow?
Capturing the scroll percentage of a div with overflow is similar to capturing the scroll percentage of an element. The main difference is that you need to use the div’s scroll top position and the div’s scroll height instead of the document’s scroll top position and document’s height. Here is a simple code snippet:$('#div').scroll(function() {
var s = $(this).scrollTop(),
d = $(this)[0].scrollHeight,
c = $(this).height();
var scrollPercent = (s / (d - c)) * 100;
console.log(scrollPercent);
});
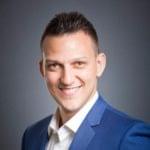
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.