jQuery break out of a foreach loop
Simple jQuery code snippet to break out of a foreach loop using jQuery’s .each() function when you’ve found the value your looking for you might not need to loop through the rest of the results.
var selected = 0;
// Iterate through item in the list. If we find the selected item, return false to break out of the loop
$('ul#mylist li').each(function(index){
if ( $(this).hasClass(‘selected’) )
{
selected = index;
return false;
}
});
console.debug('Selected position is: '+ selected);
Frequently Asked Questions (FAQs) about jQuery Break Foreach Loop
What is the purpose of the jQuery .each() function?
The jQuery .each() function is a powerful tool that allows you to iterate over a jQuery object. This function is used to loop through elements of an array or properties of an object. It is particularly useful when you want to perform the same operation on all elements in a collection or array.
How can I break out of a jQuery .each() loop?
To break out of a jQuery .each() loop, you can use the ‘return false’ statement. This will immediately terminate the loop and prevent it from iterating over the remaining elements. However, it’s important to note that this will not stop the execution of the function that contains the loop.
Can I use the ‘break’ statement to exit a jQuery .each() loop?
No, you cannot use the ‘break’ statement to exit a jQuery .each() loop. The ‘break’ statement is used to exit traditional loops like ‘for’ or ‘while’. In the context of a jQuery .each() loop, using ‘return false’ is the correct way to exit the loop.
How can I continue to the next iteration in a jQuery .each() loop?
To continue to the next iteration in a jQuery .each() loop, you can use the ‘return true’ statement. This will skip the current iteration and move on to the next one.
Can I break out of a jQuery .each() loop at a certain index value?
Yes, you can break out of a jQuery .each() loop at a certain index value. You can do this by checking the index value inside the loop and using ‘return false’ when the index matches the value at which you want to exit the loop.
Is it possible to use a ‘continue’ statement in a jQuery .each() loop?
No, you cannot use a ‘continue’ statement in a jQuery .each() loop. The ‘continue’ statement is used in traditional loops like ‘for’ or ‘while’. In the context of a jQuery .each() loop, using ‘return true’ is the equivalent of a ‘continue’ statement.
How can I access the current element in a jQuery .each() loop?
Inside a jQuery .each() loop, you can access the current element using the ‘this’ keyword. This keyword refers to the current element in the loop.
Can I use a jQuery .each() loop with an object?
Yes, you can use a jQuery .each() loop with an object. The loop will iterate over the properties of the object, and you can access the current property name and value inside the loop.
How can I use a jQuery .each() loop with an array?
To use a jQuery .each() loop with an array, you simply pass the array as the first argument to the .each() function. Inside the loop, you can access the current index and value.
Can I nest jQuery .each() loops?
Yes, you can nest jQuery .each() loops. This can be useful when you want to iterate over multi-dimensional arrays or objects. However, be aware that nested loops can lead to complex code that is harder to understand and maintain.
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
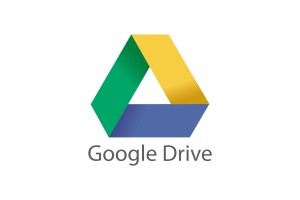
Published in
·APIs·Authentication·CMS & Frameworks·Frameworks·Laravel·Libraries·PHP·Web Services·July 22, 2016