JavaScript Operators, Conditionals & Functions
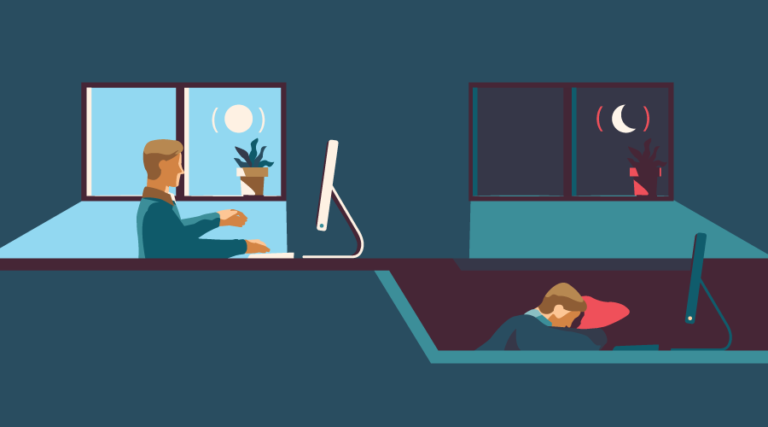
Key Takeaways
- JavaScript operators are essential tools that allow manipulation of data, including assignment, arithmetic, comparison, and logical operators.
- Conditional statements like if, else, and switch control the flow of execution based on different conditions, enabling decision-making in code.
- Functions in JavaScript are blocks of reusable code that perform specific tasks, can accept inputs, and return outputs, enhancing modularity and reusability.
- Understanding operator precedence is crucial for predicting the outcomes of operations, as it dictates the order in which operations are processed.
- Logical operators (&&, ||, !) are used to combine multiple conditions or invert boolean values, playing a critical role in complex decision-making.
- The use of functions not only simplifies code by avoiding repetition but also allows for dynamic interactions within a program by passing different arguments.
It’s essential to have a firm grasp of the fundamentals before delving into creating programs with JavaScript. In this article, we will go over some of the most important basic concepts of JavaScript that will allow you to start writing your own programs: operators, conditional statements, and functions.
These concepts are important building blocks in JavaScript and each enable essential functions for developers:
- Arithmetic Operators: Perform operations and manipulate data. For example, the addition operator (+) can be used to add two numbers together.
- Comparison Operators: Compare two or more values and return a boolean value (true or false) For example, using the greater than (>) operator to check if an integer variable is larger than another.
- Logical Operators: Used to connect two or more comparison operators and return a boolean value. For example, using the AND operator (&&) to see if a variable is between two values, i.e. that it is larger than one number and smaller the other.
- Conditional statements: Control the flow of a program based on certain conditions. For example, an if statement can be used to execute a block of code only if a certain condition is met.
- Functions: Encapsulate a set of statements to be used multiple times, making code more organized and reusable. Functions can also accept inputs and return outputs, allowing for more dynamic and flexible code.
Together, these elements provide the foundation for creating complex logic, algorithms, and systems. They are essential for developers to write programs that can make decisions, perform actions, and manipulate data.
Table of Contents
Before we begin, you should have an understanding of basic JavaScript syntax, comments, data types, and assigning values to variables. You can learn or review all that information in A Beginner’s Guide to JavaScript Variables and Data Types.
Disclaimer: This guide is intended for complete beginners to JavaScript and programming. As such, many concepts will be presented in a simplified manner.
Ready? Let’s get started!
JavaScript Operators
JavaScript operators are symbols that are used to perform different operations on data. There are several types of operators in JavaScript, and in this lesson we’ll learn about the most common ones: assignment operators, arithmetic operators, comparison operators, and logical operators.
Assignment Operators
Assignment operators, in their most basic form, apply data to a variable. In this example, I’ll assign the string "Europe"
to the variable continent
.
To create a ‘constant’ variable, one that cannot be reassigned or changed, use the const
keyword. This is useful for values in your application that would cause issues if changed unintentionally:
const continent = "Europe";
To create a variable that you intend to update, use let
:
let continent = "Europe";
...
continent = "Australia";
Before 2015, the var
keyword was used primarily to define both of the above types. It can still be used but is not best practice. Read about its use cases and history here.
Assignment is represented by the equals sign (=
). Although there are other types of assignment operators, which you can view here, this is by far the most common.
You can test all the examples throughout this article by using the console.log()
function, or by using the Console.
Arithmetic Operators
JavaScript, like all programming languages, has the built-in ability to do math, just like a calculator. Arithmetic operators perform mathematic calculations on numbers, or variables that represent numbers. You already know the most common of these — addition, subtraction, multiplication, and division.
Addition
The addition operator, represented by a plus sign (+
), will add two values and return the sum.
const x = 2 + 2; // x returns 4
Subtraction
The subtraction operator, represented by a minus sign (-
), will subtract two values and return the difference.
const x = 10 - 7; // x returns 3
Multiplication
The multiplication operator, represented by an asterisk (*
), will multiply two values and return the product.
const x = 4 * 5; // x returns 20
Division
The division operator, represented by a forward slash (/
), will divide two values and return the quotient.
const x = 20 / 2; // x returns 10
Modulus
Slightly less familiar is the modulus operator, which returns the remainder after division, and is represented by the percentage sign (%
).
const x = 10 % 3; // returns 1
3
goes into 10
three times, with 1
remainder.
Increment
A number will be incremented by one with the increment operator, represented by a double plus sign (++
).
let x = 10;
x++; // x returns 11
This happens post assignment. It is also possible to write ++x;
which happens prior to assignment. Compare:
let x = 10;
let y = x++;
// y is 10, x is 11
And:
let x = 10;
let y = ++x;
// y is 11, x is 11
Decrement
A number will be decremented by one with the decrement operator, represented by a double minus sign (--
).
let x = 10;
x--; // x returns 9
As above, it is also possible to write --x;
.
Comparison Operators
Comparison operators will evaluate the equality or difference of two values and return true
or false
. They’re usually used in logical statements.
Equal
Two equals signs (==
) means equal in JavaScript. It’s easy to be confused between single, double, and triple equals signs when you’re first learning, but remember that a single equals sign applies a value to a variable, and never evaluates equality.
const x = 8;
const y = 8;
x == y; // true
This is a loose type of equality, and will return true
even if a string is used instead of a number.
const x = 8;
const y = "8";
x == y; // true
Strict Equal
Three equals signs (===
) means strict equal in JavaScript.
const x = 8;
const y = 8;
x === y; // true
This is a more frequently used and more accurate form of determining equality than the regular equal (==
), since it requires both the type and value to be the same to return true
.
const x = 8;
const y = "8";
x === y; // false
Not Equal
An exclamation point followed by an equals sign (!=
) means not equal in JavaScript. This is the exact opposite of ==
, and will only test value, not type.
const x = 50;
const y = 50;
x != y; // false
It will treat this string and number as equal.
const x = 50;
const y = "50";
x != y; // false
Strict Not Equal
An exclamation point followed by two equals signs (!==
) means strict not equal in JavaScript. This is the exact opposite of ===
, and will test both value and type.
const x = 50;
const y = 50;
x !== y; // false
It will treat this string and number as unequal.
const x = 50;
const y = "50";
x !== y; // true
Less Than
Another familiar symbol, less than (<
) will test if the value on the left is less than the value on the right.
const x = 99;
const y = 100;
x < y; // true
Less Than or Equal To
Less than or equal to (<=
) is the same as above, but equal will also evaluate to true
.
const x = 100;
const y = 100;
x <= y; // true
Greater Than
Greater than (>
) will test if the value on the left is greater than the value on the right.
const x = 99;
const y = 100;
x > y; // false
Greater Than or Equal To
Greater than or equal to (>=
) is the same as above, but equal will also evaluate to true
.
const x = 100;
const y = 100;
x >= y; // true
Logical Operators
A logical statement will often use the comparison operators we just learned, to determine a true
or false
value. There are three additional operators that can be used in these statements to test for true
or false
.
It’s important to understand these operators before moving on to conditional statements.
And
And is represented by two ampersands (&&
). If both the statements to the left and right of &&
evaluate to true
, the whole statement returns true
.
const x = 5;
x > 1 && x < 10; // true
In the above example, x
is equal to 5
. With my logical statement, I’m testing if x
is greater than 1
and less than 10
, which it is.
const x = 5;
x > 1 && x < 4; // false
The above example returns false
because even though x
is greater than 1
, x
is not less than 4
.
Or
Or is represented by two pipes (||
). If either one of the statements to the left and right of the ||
is evaluates to true
, the statement will return true
.
const x = 5;
x > 1 || x < 4; // true
x
is not less 4
, but it is greater than 1
, so the statement returns true
.
Not
The last logical operator is not, represented by an exclamation point (!
), which returns false
if the statement is true
, and true
if the statement is false
. It also returns false
if a value exists (that does not evaluate to false
). Take a second to digest that …
const x = 99;
!x // false
Since x
exists and has a value, !x
will return false
. We can also test a Boolean value – if the value is false
, we can test it using the !
operator, it will return true
.
const x = false;
!x // true
This operator might seem confusing now, but it will make sense as we move into the next section — conditional statements.
Operator Precedence
When you learned math in school, you may have learned the PEMDAS (Please Excuse My Dear Aunt Sally) acronym to learn the Order of Operations. This stands for “Parentheses, Exponents, Multiplication, Division, Addition, Subtraction” – the order in which mathematical operations must be executed.
The same concept applies to JavaScript, except it includes more types of operators. For a full table of of operator precedence, view the reference on MDN.
Of the operators we learned, here is the correct order of operations, from highest to lowest precedence.
- Grouping (
()
) - Not (
!
) - Multiplication (
*
) - Division (
/
) - Modulus (
%
) - Addition (
+
) - Subtraction (
-
) - Less than (
<
) - Less than or equal (
<=
) - Greater than (
>
) - Greater than or equal (
>=
) - Equal (
=
) - Not equal (
!=
) - Strict equal (
===
) - Strict not equal (
!==
) - And (
&&
) - Or (
||
) - Assignment (
=
)
By way of an example, what do you expect the value of x
to be in the following snippet?
var x = 15 - 5 * 10;
Well done if you said -35
. The reason for this result is that the multiplication operator takes precedence over the subtraction operator and the JavaScript engine first evaluates 5 * 10
before subtracting the result from 15
.
To alter operator precedence you can use parentheses.
var x = (15 - 5) * 10;
// x is 100
Conditional Statements
If you’ve ever encountered a block of JavaScript code, you’ve most likely noticed the familiar English words if
and else
. These are conditional statements, or blocks of code that execute based on whether a condition is true
or false
.
All the comparison and logical operators we just learned will come in handy when evaluating these statements.
Conditional statements can be thought of as flow charts that will produce different outcome based on different results.
If / Else
If
An if statement will always be written with the keyword if
, followed by a condition in parentheses (()
), and the code to be executed in curly braces ({}
). This would be written as if () {}
. Since if
statements usually contain a larger amount of code, they’re written on multiple lines with indentation.
if () {
}
In an if
statement, the condition will only run if the statement in parentheses is true
. If it is false
, the entire block of code will be ignored.
if (condition) {
// execute code
}
First, it can be used to test for the existence of a variable.
const age = 21;
if (age) {
console.log("Your age is " + age + ".");
}
In the above example, an age
variable exists, therefore the code will print to the console. if (age)
is shorthand for if (age === true)
, since the if
statement evaluates to true
by default.
We can use the comparison operators we learned earlier to make this condition more powerful. If you’ve ever seen the website for an alcoholic product, they usually have an age limit you must enter to view the site. In America, the age is 21. They might use an if
statement to test if the user’s age is greater than or equal to 21.
const age = 21;
if (age >= 21) {
console.log("Congratulations, you can view this site.");
}
Else
If you wanted to display a different message for users who don’t meet the condition, you would use an else statement. If the first condition isn’t true, the first code block will be ignored and the else
code block will be executed.
if (condition) {
// execute code
} else {
// execute other code
}
Here is an example with a younger user. Since the user does not meet the condition, the second code block will run.
const age = 18;
if (age >= 21) {
console.log("Congratulations, you can view this site.");
} else {
console.log("You must be 21 to view this site.");
}
Else If
If there are more than two options, you can use an else if statement to execute code based on multiple conditions.
const country = "Spain";
if (country === "England") {
console.log("Hello");
} else if (country === "France") {
console.log("Bonjour");
} else if (country === "Spain") {
console.log("Buenos días");
} else {
console.log("Please enter your country.");
}
In the above example, the output will be "Buenos Días"
since the value of country
is set to "Spain"
.
Switch
There is another type of conditional statement, known as a switch statement. It is very similar to an if
statement, and performs the same function, but is written differently.
A switch
statement is useful when evaluating many possible outcomes, and is usually preferable to using many else if
statements.
A switch statement is written as switch () {}
.
switch (expression) {
case x:
// execute code
break;
case y:
// execute code
break;
default:
// execute code
}
Within the statement, you’ll see the case
, break
, and default
keywords. We’ll use the same example as we did for else if
with a switch statement to understand better.
const country = "Spain";
switch (country) {
case "England":
console.log("Hello");
break;
case "France":
console.log("Bonjour");
break;
case "Spain":
console.log("Buenos días");
break;
default:
console.log("Please enter your country.");
}
In this example, we’re evaluating the variable for a certain string, and a block of code will execute based on each case
. The break
keyword will prevent further code from running once a match is found. If no match is found, the default
code block will execute, similar to an else
statement.
Functions
A JavaScript function is a contained block of code. It can perform a task or calculation and accept arguments. One of the main reasons to use a function is to write reusable code that can produce different results each time it is run (depending on the values passed to it).
Declaration
Before a function can be used, it must be declared (or defined). A function is declared with the function
keyword, and follows the same rules for naming as variables.
A function is written as function() {}
. Here is a simple “Hello, World!” in a function.
function greeting() {
return "Hello, World!";
}
Invocation
In order to invoke (use) the function, type the name followed by parentheses.
greeting(); // returns "Hello, World!"
Parameters and Arguments
A function can also accept arguments and perform calculations. An argument is a value passed into a function. A parameter is a local variable that the function accepts and executes.
A local variable is a variable that will only work inside a specific code block.
In the example, we’re creating a function called addTwoNumbers
that, well, adds two numbers together (seriously, good naming is important). We will send the numbers 7
and 3
through as arguments, which will be accepted by the function as the parameters x
and y
.
function addTwoNumbers(x, y) {
return x + y;
}
addTwoNumbers(7, 3); // returns 10
Since 7
+ 3
= 10
, the function will return 10
. Below, you will see how functions are reusable, as we’ll pass different arguments to the exact same function to produce a different output.
function addTwoNumbers(x, y) {
return x + y;
}
addTwoNumbers(100, 5); // returns 105
There are a couple of other ways of declaring functions in JavaScript. You can read more about those in this article: Quick Tip: Function Expressions vs Function Declarations.
Conclusion
In this article, we learned three very important fundamental concepts of JavaScript: operators, conditional statements, and functions. Operators are symbols that perform operations on data, and we learned about assignment, arithmetic, comparison, and logical operators. Conditional statements are blocks of code that execute based on a true or false result, and functions are contained blocks of reusable code that perform a task.
With this knowledge, you’re ready to move on to more intermediate concepts of JavaScript. If you have any questions or comments about the material presented, I’d be happy to hear them in the comments below (all the more so if you’re just getting your feet wet with JavaScript).
This article was peer reviewed by James Kolce and Tom Greco. Thanks to all of SitePoint’s peer reviewers for making SitePoint content the best it can be!
Frequently Asked Questions (FAQs) about JavaScript Operators, Conditionals, and Functions
What is the significance of operator precedence in JavaScript?
Operator precedence in JavaScript determines the way operators are parsed concerning each other. Operators with higher precedence become the operands of operators with lower precedence. Understanding operator precedence is crucial as it helps to predict the outcome of an operation. For instance, multiplication (*) and division (/) have higher precedence than addition (+) and subtraction (-). Therefore, in an expression like 2 + 3 * 4, the multiplication happens first, resulting in 2 + 12 = 14, not 20.
How can I change the order of operations in JavaScript?
You can change the order of operations in JavaScript by using parentheses. The expressions inside parentheses are evaluated first. For example, in the expression (2 + 3) * 4, the addition operation is performed first because it’s inside the parentheses, resulting in 5 * 4 = 20.
What are conditional statements in JavaScript?
Conditional statements in JavaScript are used to perform different actions based on different conditions. The most common conditional statements are if, else if, and else. These statements allow the program to make decisions and execute the appropriate code block based on the condition’s truthiness.
How do JavaScript functions work?
Functions in JavaScript are blocks of code designed to perform a particular task. A JavaScript function is executed when something invokes it (calls it). Functions can be defined using the function keyword, followed by a name, and parentheses (). The code to be executed by the function is placed inside curly brackets {}.
What are the different types of operators in JavaScript?
JavaScript includes various types of operators such as arithmetic operators (+, -, *, /, %, ++, –), assignment operators (=, +=, -=, *=, /=, %=), comparison operators (==, ===, !=, !==, >, <, >=, <=), logical operators (&&, ||, !), and bitwise operators (&, |, ^, ~, <<, >>, >>>).
How does the ternary operator work in JavaScript?
The ternary operator is a shorthand way of writing an if-else statement. It’s called the ternary operator because it takes three operands – a condition, a result for true, and a result for false. For example, the code let result = (a > b) ? ‘a is greater’ : ‘b is greater’; will assign the string ‘a is greater’ to the result if a is greater than b, and ‘b is greater’ if a is not greater than b.
What is the difference between == and === operators in JavaScript?
The == operator checks for equality in value but not type. It performs type coercion if the variables being compared are not of the same type. On the other hand, the === operator, also known as the strict equality operator, checks for both value and type. It does not perform type coercion, so if the variables being compared are not of the same type, it will return false.
How can I define a function in JavaScript?
A function in JavaScript can be defined using the function keyword, followed by a unique function name, a list of parameters (that might be empty), and a statement block surrounded by curly braces. For example, function myFunction(parameter1, parameter2) { // code to be executed }.
What are JavaScript logical operators?
JavaScript logical operators are used to determine the logic between variables or values. The three logical operators are AND (&&), OR (||), and NOT (!). The AND operator returns true if both operands are true, the OR operator returns true if at least one operand is true, and the NOT operator returns the inverse of the given boolean value.
How does the switch statement work in JavaScript?
The switch statement in JavaScript is used to perform different actions based on different conditions. It’s a more efficient way to code when dealing with many conditions. The switch expression is evaluated once, and its value is compared with the values of each case. If there’s a match, the associated block of code is executed.
A web developer from Chicago who blogs about code and design, and enjoys losing at Age of Empires 2 in her spare time.