Introducing the Screen Orientation API
Everyone uses the web in a different way. There are people who use it to chat, others use it to watch movies, and others use it to play games. For those of you who access the internet to play games on their mobile device, I have a question: have you ever played a game that asks you to rotate the screen to start? I bet the answer is yes.
The reason you have to rotate the screen is that the UI has been developed for a specific mode, portrait or landscape. If your web application has the same need, or you are developing one of these games, I have a JavaScript API for you: the Screen Orientation API. In this article I’ll discuss what it is and what it is good for.
Key Takeaways
- The Screen Orientation API enables developers to read the screen orientation state, be informed when this state changes, and lock the screen orientation to a specific state. This can help improve user experience by eliminating the need for instructions on screen rotation.
- The API extends the screen object of the window with a property, orientation, and two methods, lockOrientation() and unlockOrientation(). The orientation property returns a string representing the screen orientation while the two methods allow locking and unlocking of the screen orientation.
- The API also provides an ‘onorientationchange’ event that fires every time the screen orientation changes, allowing developers to detect changes of the orientation and update the UI or business logic of the website accordingly.
- Browser support for the Screen Orientation API is good, with Firefox 18+, Internet Explorer 11+, Chrome 38+, and Opera 25+ supporting it. However, Safari does not currently support this API. Developers should always check if the API is implemented in the user’s browser before using it.
What is the Screen Orientation API?
The Screen Orientation API provides the ability to read the screen orientation state, to be informed when this state changes, and to be able to lock the screen orientation to a specific state.
In other words, you are now able to detect the orientation of a user’s device (in terms of portrait and landscape) and lock in the mode you need. Doing so, you don’t need to show your users weird animations and labels to specify the orientation needed. In fact, you can now set the device’s screen in the orientation needed so that the user will understand how to rotate the device.
The Screen Orientation API is at a very early stage, as it’s a W3C Working Draft. The current specifications may be superseded in a few months by a newer version that is currently in progress. The new version is slightly different from the old one because it introduces a new property and a different return type for one of the methods.
It’s also worth noting that to lock the screen, the web page must be in full-screen mode (you can achieve this using the Fullscreen API).
Now that you know what the Screen Orientation API is, let’s discover more about its properties and methods.
Properties and Events
The Screen Orientation API extends the screen
object of window
with a property, orientation
, and two methods, lockOrientation()
and unlockOrientation()
.
The orientation
property returns a string representing the orientation of the screen. Its value can be one of the followings:
portrait-primary
: The orientation is in the primary portrait mode. For a smartphone this values means that it’s in a vertical position with the buttons at the bottom.portrait-secondary
: The orientation is in the secondary portrait mode. For a smartphone this value means that it’s in a vertical position with the buttons at the top (the device is down under)landscape-primary
: The orientation is in the primary landscape mode. For a smartphone this value means that it’s in a horizontal position with the buttons at the right.landscape-secondary
: The orientation is in the secondary landscape mode. For a smartphone this value means that it’s in a horizontal position with the buttons at the left.
The lockOrientation()
and unlockOrientation()
methods are complementary in what they do. The first method locks the device’s screen as if the device was physically rotated in a certain position, as shown in the figure below. It returns a Boolean which is true
in case of success of the operation and false
otherwise.
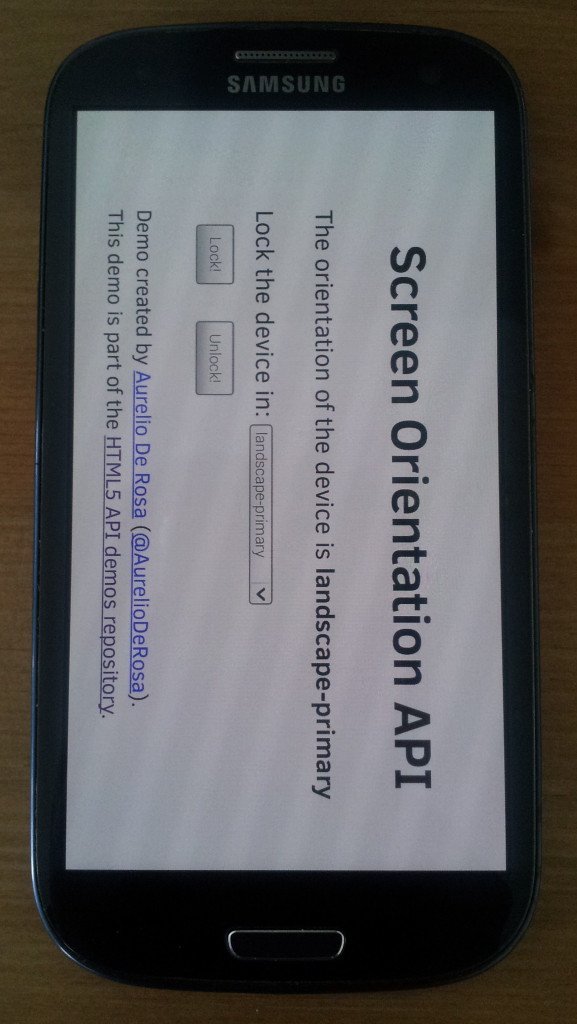
lockOrientation()
accepts one or more strings to specify the orientations we want to lock the screen at. The strings must be one of the previously mentioned values of the orientation
property, portrait
to specify the orientation can be either portrait-primary
and portrait-secondary
, or landscape
to indicate the orientation can be either landscape-primary
and landscape-secondary
.
An example of code that uses this method and passes one string is shown below:
window.screen.lockOrientation('portrait');
Here is an example that passes more than one string:
window.screen.lockOrientation('portrait-primary', 'landscape-primary');
The unlockOrientation()
method is used to release a previously set lock and allows the screen to rotate again in every position.
The API also provides an event named onorientationchange
that is fired every time the screen orientation changes. We can use this event to detect changes of the orientation and update the UI or the business logic of our website accordingly.
Browser Compatibility
The support for the Screen Orientation API is very good, although some browsers still use the prefixed version. The API is supported by Firefox 18+ using its vendor prefix (moz
), Internet Explorer 11+ using its vendor prefix (ms
), Chrome 38+ (currently in beta), and Opera 25+ (currently in beta). Unfortunately, just like many other really interesting and useful APIs, Safari doesn’t have any support.
As you can see, with the next release of Chrome and Opera, you’ll have almost every major browser supporting this API. So, you can really use it in your next project. Even without support, we’ve learned to develop our projects based on feature detection. To test if the API is implemented your browser, you can write:
if ('orientation' in screen) {
// API supported, yeah!
} else {
// API not supported :(
}
So far, we’ve covered the properties and the events exposed by the API as well as the API’s use cases. In the next section, we’re going to create a simple web page to see the Screen Orientation API in action.
Demo
The demo we’re going to develop consists of an HTML page that displays text indicating the current orientation of the screen. Then, we have a select box to specify the orientation we want to lock the screen at. Finally, we have two buttons: one to lock the screen and the other to unlock it.
Inside the JavaScript code we detect if the browser supports this API or not, and what prefix it uses, if any. If the API isn’t implemented in the browser we display the message “API not supported” and the buttons will be disabled. If the API is supported we attach a handler to the correct event (the name varies because of the prefixes) where we update the text of the paragraph to show the current orientation.
Finally, we create other two handlers. In the first we set the page in full-screen mode and lock the screen in the orientation specified by the select box. In the second we remove the lock and exit full-screen mode.
Important note: While developing and testing this demo I’ve found a couple of bugs in Firefox. The browser crashes with any value passed to lockOrientation()
(actually mozLockOrientation()
) but portrait-primary
and portrait-secondary
. In addition, when portrait-secondary
is given, Firefox acts as if the string was landscape-primary
. Based on my tweets, two bugs have been filed (https://bugzil.la/1061372 and https://bugzil.la/1061373) and hopefully they will be fixed soon.
You can find the complete code for the demo below. You can also play with it online if you like.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
<meta name="author" content="Aurelio De Rosa">
<title>Screen Orientation API Demo by Aurelio De Rosa</title>
<style>
*
{
-webkit-box-sizing: border-box;
-moz-box-sizing: border-box;
box-sizing: border-box;
}
body
{
max-width: 500px;
margin: 2em auto;
padding: 0 0.5em;
font-size: 20px;
}
h1
{
text-align: center;
}
.api-support
{
display: block;
}
.hidden
{
display: none;
}
.value
{
font-weight: bold;
}
.button-demo
{
padding: 0.5em;
margin: 1em;
}
.author
{
display: block;
margin-top: 1em;
}
</style>
</head>
<body>
<h1>Screen Orientation API</h1>
<span id="so-unsupported" class="api-support hidden">API not supported</span>
<div id="so-results">
<p>
The orientation of the device is <span id="orientation" class="value">unavailable</span>
</p>
<form id="form-orientation">
<label for="orientation">Lock the device in:</label>
<select id="orientation-type">
<option value="portrait">portrait</option>
<option value="landscape">landscape</option>
<option value="portrait-primary">portrait-primary</option>
<option value="portrait-secondary">portrait-secondary</option>
<option value="landscape-primary">landscape-primary</option>
<option value="landscape-secondary">landscape-secondary</option>
</select>
<br />
<input class="button-demo" id="lock-button" type="submit" value="Lock!" />
<button class="button-demo" id="unlock-button">Unlock!</button>
</form>
</div>
<small class="author">
Demo created by <a href="http://www.audero.it">Aurelio De Rosa</a>
(<a href="https://twitter.com/AurelioDeRosa">@AurelioDeRosa</a>).<br />
This demo is part of the <a href="https://github.com/AurelioDeRosa/HTML5-API-demos">HTML5 API demos repository</a>.
</small>
<script>
var prefix = 'orientation' in screen ? '' :
'mozOrientation' in screen ? 'moz' :
'msOrientation' in screen ? 'ms' :
null;
if (prefix === null) {
document.getElementById('so-unsupported').classList.remove('hidden');
['lock-button', 'unlock-button'].forEach(function(elementId) {
document.getElementById(elementId).setAttribute('disabled', 'disabled');
});
} else {
var form = document.getElementById('form-orientation');
var select = document.getElementById('orientation-type');
// Function needed to see lock in action
function launchFullscreen(element) {
if(element.requestFullscreen) {
element.requestFullscreen();
} else if(element.mozRequestFullScreen) {
element.mozRequestFullScreen();
} else if(element.webkitRequestFullscreen) {
element.webkitRequestFullscreen();
} else if(element.msRequestFullscreen) {
element.msRequestFullscreen();
}
}
function exitFullscreen() {
if(document.exitFullscreen) {
document.exitFullscreen();
} else if(document.mozCancelFullScreen) {
document.mozCancelFullScreen();
} else if(document.webkitExitFullscreen) {
document.webkitExitFullscreen();
} else if (document.msExitFullscreen) {
document.msExitFullscreen();
}
}
function orientationHandler() {
var orientationProperty = prefix + (prefix === '' ? 'o' : 'O') + 'rientation';
document.getElementById('orientation').textContent = screen[orientationProperty];
}
screen.addEventListener(prefix + 'orientationchange', orientationHandler);
document.getElementById('lock-button').addEventListener('click', function(event) {
event.preventDefault();
launchFullscreen(document.documentElement);
setTimeout(function() {
screen[prefix + (prefix === '' ? 'l' : 'L') + 'ockOrientation'](select.value);
}, 1);
});
document.getElementById('unlock-button').addEventListener('click', function() {
exitFullscreen();
screen[prefix + (prefix === '' ? 'u' : 'U') + 'nlockOrientation']();
});
orientationHandler();
}
</script>
</body>
</html>
Conclusion
In this article we’ve discussed the Screen Orientation API. This API exposes one property that specifies the orientation of the screen and two methods. The first method allows you to lock the screen in a given orientation, while the second releases the lock. Finally, it provides an event to know when a change of orientation happens.
As you’ve seen, using this API is quite simple and it should not be too difficult for you to employ it in a future project. Finally, the support among browsers is very good, so this is really something you can plan to adopt.
Frequently Asked Questions (FAQs) about Screen Orientation API
What is the Screen Orientation API and how does it work?
The Screen Orientation API is a web-based interface that allows web applications to access and control the screen orientation of a device. It works by providing methods and properties that enable the web application to read the current screen orientation and lock or unlock the screen orientation. This API is particularly useful for web applications that need to adapt their layout or functionality based on the screen orientation, such as games, video players, or responsive web designs.
How can I use the Screen Orientation API in my web application?
To use the Screen Orientation API in your web application, you first need to check if the API is available in the user’s browser. You can do this by checking if the ‘orientation’ property exists in the ‘screen’ object. If it does, you can then use the ‘lock’ method to lock the screen orientation and the ‘unlock’ method to unlock it. You can also use the ‘onchange’ event to react to changes in the screen orientation.
What are the different screen orientations that the Screen Orientation API can detect?
The Screen Orientation API can detect four different screen orientations: ‘portrait-primary’, ‘portrait-secondary’, ‘landscape-primary’, and ‘landscape-secondary’. The ‘portrait-primary’ orientation is the default portrait mode, while the ‘portrait-secondary’ orientation is the upside-down portrait mode. The ‘landscape-primary’ orientation is the default landscape mode, while the ‘landscape-secondary’ orientation is the upside-down landscape mode.
Can I lock the screen orientation to a specific mode using the Screen Orientation API?
Yes, you can lock the screen orientation to a specific mode using the Screen Orientation API. You can do this by calling the ‘lock’ method with the desired screen orientation as the argument. For example, if you want to lock the screen orientation to the landscape mode, you can call ‘screen.orientation.lock(‘landscape’)’.
What happens if I try to use the Screen Orientation API in a browser that does not support it?
If you try to use the Screen Orientation API in a browser that does not support it, the ‘orientation’ property in the ‘screen’ object will be undefined. Therefore, it is important to always check if this property exists before using the API. If it does not exist, you should provide a fallback solution for handling the screen orientation.
Can I use the Screen Orientation API in mobile web applications?
Yes, you can use the Screen Orientation API in mobile web applications. In fact, this API is particularly useful for mobile web applications that need to adapt their layout or functionality based on the screen orientation, such as games, video players, or responsive web designs.
How can I detect changes in the screen orientation using the Screen Orientation API?
You can detect changes in the screen orientation using the Screen Orientation API by listening to the ‘onchange’ event. This event is fired whenever the screen orientation changes. You can add an event listener for this event and define a function that will be executed when the event is fired.
Can I unlock the screen orientation using the Screen Orientation API?
Yes, you can unlock the screen orientation using the Screen Orientation API. You can do this by calling the ‘unlock’ method. This method removes any screen orientation lock that has been set, allowing the screen orientation to change according to the user’s device orientation.
What are some use cases for the Screen Orientation API?
Some use cases for the Screen Orientation API include games, video players, and responsive web designs. For example, a game might want to lock the screen orientation to the landscape mode for a better gaming experience. A video player might want to switch to the full-screen mode when the screen orientation changes to the landscape mode. A responsive web design might want to adapt its layout based on the screen orientation.
Is the Screen Orientation API supported in all browsers?
The Screen Orientation API is not supported in all browsers. As of now, it is supported in most modern browsers, including Chrome, Firefox, and Opera. However, it is not supported in Internet Explorer and Safari. Therefore, it is important to always check if the ‘orientation’ property exists in the ‘screen’ object before using the API.
I'm a (full-stack) web and app developer with more than 5 years' experience programming for the web using HTML, CSS, Sass, JavaScript, and PHP. I'm an expert of JavaScript and HTML5 APIs but my interests include web security, accessibility, performance, and SEO. I'm also a regular writer for several networks, speaker, and author of the books jQuery in Action, third edition and Instant jQuery Selectors.
Published in
·Cloud·CMS·Databases·Debugging & Deployment·Development Environment·PHP·Programming·Web·March 12, 2014