Hints for Making a Cross-platform HTML5 Game
You already know that HTML5 is great for game development, right? Perhaps best of all, it provides a way for you to develop cross-platform applications, effectively reducing the time needed to spend on browser testing. Even so, developing a HTML5 game is not always an easy task. In this post, I’m going to share some tips for developing a quality, cross-platform HTML5 game.
Key Takeaways
- Prioritize the mobile game experience in HTML5 game development, given the significant number of mobile players and the need for optimized performance on these devices.
- Begin thinking about cross-platform contingencies early in the development phase to ensure the game is suitable for all platforms, including mobile and desktop.
- Gain a strong understanding of CSS as it forms a significant part of cross-platform HTML5 game development, and consider using the DOM (Document Object Model) for faster and more responsive games.
First Priority: Mobile
The mobile-first policy is something I ignored in my first HTML5 game project. From now on, I’ll never underestimate the amount of mobile players out there. I’m a game player. I love playing Angry Birds on the desktop, and I appreciate the fact I can play it on my Android device when not at home.
If I’d figured out this earlier, I would have spent more time and energy optimizing the mobile game experience instead of concentrating on the desktop browser experience with my first HTML5 game. Although I took it into consideration, mobile was only treated as a secondary game environment. Make mobile game experience optimization a priority.
Think Cross-platform Early
Take Zynga’s CityVille as an example. I’ve played CityVille before, as well as CityVille Hometown (the mobile version) on my Android device. Frankly, the game experiences are quite different. CityVille Hometown revolves around a a totally different gaming experience.
From a game developer’s perspective, CityVille is not suitable for a mobile platform — it would need to change significantly. Its menu is too large and complex for an iPhone’s screen; that’s why Zynga published individual versions of CityVille Hometown for iOS and Android. It pays to think of your cross-platform contingencies early on in the development phase.
Multi-platform Detection
Once you start to develop an HTML5 game, you may want to show different styles and forms of content (such as different user interfaces) for players on different platforms. This can become quite complicated, because whatever language you choose to specialize in, different devices always have differing levels of support for JavaScript, CSS3 and so on.
Use of JavaScript
JavaScript is pretty popular with most game developers. In the following code snippet, the JavaScript property navigator.userAgent
returns a string object and enables you to detect the name and version of any web browser.
<script type="text/javascript">// <![CDATA[ var mobile = (/iphone|ipad|ipod|android|blackberry|mini|windowssce|palm/i.test(navigator.userAgent.toLowerCase())); if (mobile) { document.location = "http://www.yoursite.com/mobile.html"; } // ]]></script>
The problem is that many mobile devices don’t (currently) support JavaScript, and using one JavaScript script to detect all browser types could create a page performance nightmare for your users.
Use of CSS @media queries
Media queries are a good way of displaying CSS styles for mobile devices. You simply create one page and two style sheets — the first for a desktop screen, and the second for mobile devices. You just need to maintain one game page, and the style sheet defines how it looks.
Let’s suppose the screen size is 800px wide. We can use media queries to hide or move UI elements that aren’t critical for display on mobile devices:
@media screen and (max-width: 800px) {
/* Whatever CSS you want here */
}
Use of PHP, JSP, ASP, etc
Another method uses a server-side language (PHP, JSP, ASP, etc) to detect the browser type. It doesn’t rely on a scripting language or CSS, which a mobile device doesn’t use. A simple PHP code for detection might look like this:
<? if ( stristr($ua, "Windows CE") or stristr($ua, "AvantGo") or stristr($ua,"Mazingo") or stristr($ua, "Mobile") or stristr($ua, "T68") or stristr($ua,"Syncalot") or stristr($ua, "Blazer") ) { $DEVICE_TYPE="MOBILE"; } if (isset($DEVICE_TYPE) and $DEVICE_TYPE=="MOBILE") { $location='mobile/index.php'; header ('Location: '.$location); exit; } ?>
Bone Up on Your CSS Knowledge
Study and improve your CSS. Developing a game that runs on desktop, iOS and Android requires a large amount of CSS knowledge. Now, CSS3 is so powerful that you can make games entirely with it. In my team, there is a guy responsible for all CSS-related work, and he creates dynamically changing menu sizes depending on the mobile device. In cross-platform HTML5 game development, a good 50% of your work could relate to CSS.
Test with the DOM
When I began HTML5 game development, I thought it was a nice idea to render game using Canvas, because it’s quite useful on desktop computers. But on a platform like the iPhone 3GS, it turns out to be a bit of a headache. The game performance sucked; images were rendering at less than 5fps. I had to create rendering engines, one after another.
Currently, I rely on the DOM (Document Object Model). It dictates the way in which my applications are constructed of HTML elements and CSS3 selectors, rather than how they’re rendered to a Canvas. The advantage of using the DOM is that the game can be much faster and more responsive, due to hardware acceleration. It’s important to test your game again and again, and I personally think using the DOM is a better choice than Canvas.
Deal with Audio Issues
Embedding sounds within a game is vital. Unfortunately, the biggest issue in developing a cross-platform HTML5 game is sound. Within HTML5 game development, Canvas has good support, Video is constantly improving, but Audio is still pretty patchy. Games can’t play sound and music simultaneously. Taken from this aspect, HTML5 is not necessarily an ideal Flash alternative!
Other audio issues include:
- Different browsers support different sound formats (mp3, wav, ogg etc)
- iPhones and iPads allow only one mp3 sound to be loaded and played at any time
- Android has limited support of the <audio> tag and the JavaScript audio API
There are a few ways around these issues:
- For the format issue, I duplicate all game sounds in two formats (OGG and MP3), which covers most browsers.
- For the iOS platform, I keep sound and music in the desktop version of the game, and only music in the mobile version.
- As for Android, because it supports Flash audio play, so I use jPlayer to handle automatic HTML5/Flash audio transition.
However, all these should be temporary measures. The real solution for the issues surrounding HTML5 audio involves the W3C working with app and browser developers to create a good standard that everyone can follow.
Conclusion
I hope these tips are helpful in guiding you in the development of a cross-platform HTML5 game. Although it is not an easy task, and there are still problems such as audio integration, there is no reason not to develop a game that can run across multiple platforms and bring your game experience to a wide variety of users.
Frequently Asked Questions (FAQs) about Cross-Platform HTML5 Game Development
What are the key considerations when developing a cross-platform HTML5 game?
When developing a cross-platform HTML5 game, it’s crucial to consider the game’s performance across different platforms. This includes ensuring the game runs smoothly and maintains a consistent user experience on various devices and operating systems. It’s also important to consider the game’s scalability, as it should be able to handle a large number of users without compromising performance. Additionally, the game should be designed with a responsive layout to accommodate different screen sizes and resolutions.
How can I optimize my HTML5 game for mobile devices?
Optimizing your HTML5 game for mobile devices involves several steps. First, ensure your game is responsive and can adapt to different screen sizes. Second, optimize your game’s performance by minimizing the use of heavy graphics and animations that can slow down the game. Third, consider touch controls and design your game’s interface to be user-friendly on smaller screens. Lastly, test your game on various mobile devices to ensure it runs smoothly and provides a consistent user experience.
What tools can I use for cross-platform HTML5 game development?
There are several tools available for cross-platform HTML5 game development. These include game engines like Phaser, Construct 2, and Unity, which provide a robust framework for developing games. Additionally, tools like Adobe Animate and Tumult Hype can be used for creating animations and interactive content. For coding, you can use text editors like Sublime Text or Visual Studio Code.
How can I ensure my HTML5 game is accessible to all users?
Ensuring your HTML5 game is accessible involves considering various factors. This includes providing alternative text for images, ensuring the game can be navigated using keyboard controls, and providing captions for audio content. Additionally, consider color contrast and font size to ensure the game is visually accessible.
How can I monetize my HTML5 game?
There are several ways to monetize your HTML5 game. This includes in-game advertising, in-app purchases, and selling the game outright. Additionally, you can offer premium features or content for a fee. It’s important to choose a monetization strategy that aligns with your game’s design and target audience.
How can I test the performance of my HTML5 game?
Testing the performance of your HTML5 game involves using tools like Google’s Lighthouse or WebPageTest. These tools can provide insights into your game’s load time, responsiveness, and overall performance. Additionally, it’s important to test your game on various devices and browsers to ensure it runs smoothly and provides a consistent user experience.
What are some common challenges in cross-platform HTML5 game development?
Some common challenges in cross-platform HTML5 game development include ensuring consistent performance across different platforms, optimizing the game for mobile devices, and dealing with browser compatibility issues. Additionally, designing a game that is accessible and user-friendly on various devices can be challenging.
How can I improve the user experience of my HTML5 game?
Improving the user experience of your HTML5 game involves several steps. This includes ensuring the game loads quickly, runs smoothly, and is responsive on various devices. Additionally, the game’s interface should be intuitive and easy to navigate. Providing clear instructions and feedback can also enhance the user experience.
How can I handle browser compatibility issues in HTML5 game development?
Handling browser compatibility issues in HTML5 game development involves using tools like Modernizr, which can detect features supported by the user’s browser. Additionally, consider using polyfills to provide fallbacks for unsupported features. It’s also important to test your game on various browsers to identify and fix compatibility issues.
How can I keep my HTML5 game secure?
Keeping your HTML5 game secure involves several steps. This includes using secure protocols like HTTPS, sanitizing user input to prevent cross-site scripting attacks, and regularly updating your game to fix any security vulnerabilities. Additionally, consider using tools like Content Security Policy (CSP) to prevent unauthorized access to your game’s data.
Benny is an HTML5 game developer and host of the 7plusDezine blog. He loves to share his game development experiences, creative thoughts and valuable design resources with the whole online community. Here is my link to 7plusDezine:
Published in
·Animation·Canvas & SVG·Design·Design & UX·Entrepreneur·HTML & CSS·Illustration·Review·Software·September 22, 2014
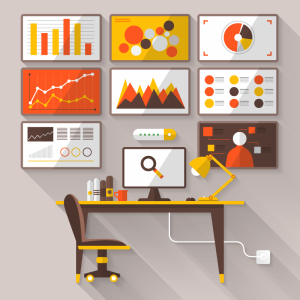
Published in
·automation·Debugging & Deployment·Development Environment·Miscellaneous·PHP·November 30, 2016