This article will outline what you need to know about WordPress and its filtering mechanism available to you via your posts administration screen.
We will detail exactly what the WordPress filters are and the purpose they serve in displaying your post listings. From there we will learn how you can extend your post administration area – by creating new customized WordPress administration filters.
By the end you will be able to take what you have learned and apply it to your own projects, allowing you to provide additional information and functionality for your end users.
Filtering Posts
WordPress will output its default filter mechanisms to the posts administration screen. These filters allow you to display only certain posts that match set criteria. For example, you may want to display posts published within a set date period (as shown below).

WordPress comes pre-configured with several filters that you can use on your posts administration screen. Additional filters may be added by certain themes and plugins and provide additional filters for you to use.
Overall these filters serve the same purpose of allowing you to narrow down your listing of posts based on a set of criteria, viewing only the posts that are relevant given your set criteria.
Adding Your Own Filters
While WordPress comes with its own great set of filters, often you may want to add your own to help your users (or yourself) in narrowing down posts on your administration screen.
You can add additional filters to your website via the use of two hooks
restrict_manage_posts
pre_get_posts
These two hooks, when combined will allow you to filter your posts by your chosen criteria and return only posts / items that match that criteria.
Before we go through and explore these hooks, lets talk about a real world example (so that when we start building our filters you can see how they may be useful).
Imagine that we are building a self managed website where each post
will be manually assigned a post format
and automatically assigned a post author
When we are on our administration listing page for our posts it will show the user all posts, regardless of what format the post is in or the author who wrote it. This screen can be messy and hard to read through (and often will require flipping through pages of records to find what you need)
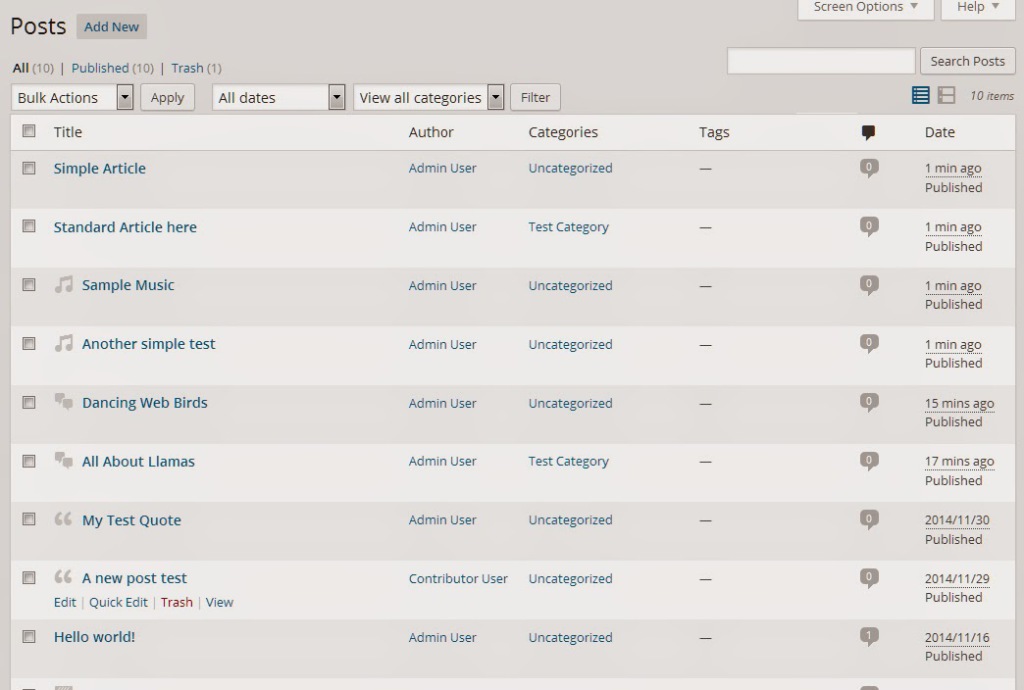
Even though a post will have link with the name of the author, it is possible the user will simply overlook this (along with the small icon displayed that represents the post format type of the post).
It is more intuitive to add drop downs to the top of each administration listing screen that implicitly show that your posts will be filtered. This is what we will be doing in this tutorial.
Creating the Select Drop Down Using the ‘restrict_manage_posts’ Filter
The first thing that needs to be done is the creation of the select drop down for the two additional filters we will be making.
You will need to navigate over to your child themes functions.php
file (or another applicable file) and add code which will add these two new filters to your posts administration screen.
Filtering by Author
//defining the filter that will be used to select posts by 'post formats'
function add_post_formats_filter_to_post_administration(){
//execute only on the 'post' content type
global $post_type;
if($post_type == 'post'){
$post_formats_args = array(
'show_option_all' => 'All Post formats',
'orderby' => 'NAME',
'order' => 'ASC',
'name' => 'post_format_admin_filter',
'taxonomy' => 'post_format'
);
//if we have a post format already selected, ensure that its value is set to be selected
if(isset($_GET['post_format_admin_filter'])){
$post_formats_args['selected'] = sanitize_text_field($_GET['post_format_admin_filter']);
}
wp_dropdown_categories($post_formats_args);
}
}
add_action('restrict_manage_posts','add_post_formats_filter_to_post_administration');
Lets step though this code so that you can get an understanding of exactly what we are doing.
- We create a function and attach it to the
restrict_manage_posts
action. This gives us access to the filter zone shown at the top of the posts administration page. - We get the current post type listing being displayed via the global
post_type
. We use this to determine if we are on the correct post type (we only want to execute this for posts, not pages or other content types). - We intent to call the
wp_dropdown_categories
WordPress function which will generate a drop down list of all post formats based on a set of criteria. Post formats are actually just one of WordPress’s custom taxonomies (much like categories or tags) and as such we can use this function by supplying a list of arguments.show_option_all
– This determines the name that will be shown when we do not want to filter anything (we use this so that you can show ‘all post formats’ and will see all of your post formats).orderby
– This is how this will be ordered, I have chosen to order this by its name.order
– This determines which way the list will be sorted, I have chosen ascending.name
– This is the name of the drop down list itself. You need to give this a unique name so that you can fetch its chosen value later on.taxonomy
– (This is important) This value determines which elements will be pulled into the list. Since post formats are just a taxonomy we can specify its taxonomy name ofpost_format
and it will collect the post format terms.
- After we declare our arguments for the
wp_dropdown_categories
function, we search the global$_GET
variable to see if we have actually already chosen a post format type to filter by. If a value exists we need to add a new argument onto our argument array calledselected
which basically tells the drop down list which value should be chosen by default.
Filtering by Author
//defining the filter that will be used so we can select posts by 'author'
function add_author_filter_to_posts_administration(){
//execute only on the 'post' content type
global $post_type;
if($post_type == 'post'){
//get a listing of all users that are 'author' or above
$user_args = array(
'show_option_all' => 'All Users',
'orderby' => 'display_name',
'order' => 'ASC',
'name' => 'aurthor_admin_filter',
'who' => 'authors',
'include_selected' => true
);
//determine if we have selected a user to be filtered by already
if(isset($_GET['aurthor_admin_filter'])){
//set the selected value to the value of the author
$user_args['selected'] = (int)sanitize_text_field($_GET['aurthor_admin_filter']);
}
//display the users as a drop down
wp_dropdown_users($user_args);
}
}
add_action('restrict_manage_posts','add_author_filter_to_posts_administration');
Lets step through this code so you can get an understanding of exactly what we are doing
- We create a function and attach it to the
restrict_manage_posts
action. This will give us access to the filters at the top of the posts administration screen. - We use the global
post_type
variable to check to see if we are currently on the correct posts administration screen. We only want to execute onposts
. We want to use the
wp_dropdown_users
function to generate a listing of users on the website. We need to supply the following arguments to it.show_option_all
– This determines the default option for the select list. In our case we want it to be ‘All Users’ which would mean we don’t want to filter anything at all.orderby
– Determines how the list will be ordered. I have chosen to order just by the name of the author.order
– Determines in what order the list will be ordered, I have chosen ascending order.name
– The name of the drop down, which will be used later on when we need to fetch the chosen value so we can filter the posts.who
– Determines who will be chosen. The only value we can set isauthors
which will fetch all users who can create posts.include_selected
– I have chosen to enable this for simplicities sake.
After we declare our arguments for the
wp_dropdown_users
function, we check the global$_GET
to see if we have the drop down value set. If this is set it means that we are already filtering by a user and we need to update the arguments. We set the value ofselected
to the ID of the author which ensures that the author is selected by default when the page loads.
After adding both of these filters your posts administration screen should have an additional set of drop down lists. One for your post formats and another for your authors as seen below
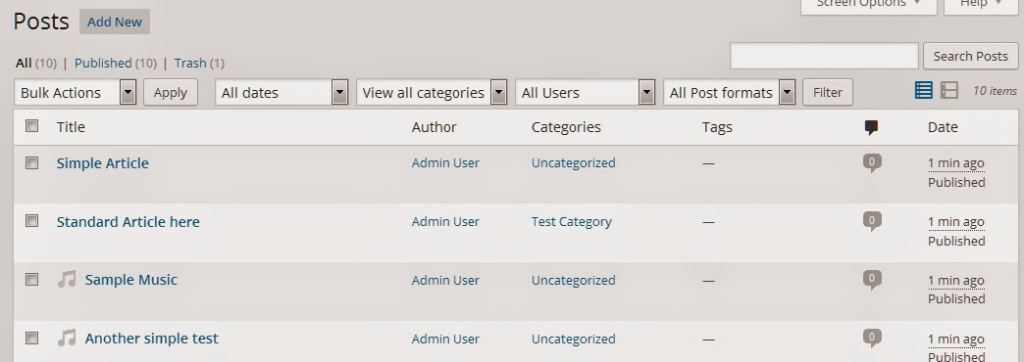
Filtering the Posts Using the ‘pre_get_posts’ Filter
Now that we have an interface in which the user can select their post format and / or authors, we need to filter the listing of posts based on these drop downs.
We will do this by hooking into the pre_get_posts
filter.
The pre_get_posts
filter has access to the current query
before it is executed by WordPress and will let us change the posts that will be fetched from the database.
The overall idea is to modify the query
in such as way as to restrict which posts will be brought into the administration listing. To do this for both post formats and authors is slightly different so I have broken down each method below
Filtering for Post Formats
//restrict the posts by the chosen post format
function add_post_format_filter_to_posts($query){
global $post_type, $pagenow;
//if we are currently on the edit screen of the post type listings
if($pagenow == 'edit.php' && $post_type == 'post'){
if(isset($_GET['post_format_admin_filter'])){
//get the desired post format
$post_format = sanitize_text_field($_GET['post_format_admin_filter']);
//if the post format is not 0 (which means all)
if($post_format != 0){
$query->query_vars['tax_query'] = array(
array(
'taxonomy' => 'post_format',
'field' => 'ID',
'terms' => array($post_format)
)
);
}
}
}
}
add_action('pre_get_posts','add_post_format_filter_to_posts');
Let us break this code down and run through exactly what it is we are doing:
- We create a function called
add_post_format_filter_to_posts
and hook it onto thepre_get_posts
filter. This filter has access to the globalquery
variable so we also pass that into our function so we can manipulate it. This variable is passed by reference so we don’t need to return or echo any values, any changes to the query will stay. - We get the global
post_type
andpage_now
variables and check to seepage_now
is equal toedit.php
andpost_type
is equal topost
. Essentially we are checking to make sure we are on the post administration screen. - We determine if a post format has been selected by checking the
$_GET
variable. If it has been set, we collect its value. - We compare the chosen value to ensure it is not our default value of 0 (default meaning it wants to show all post formats, which is the exact same as not applying a post format filter). As long as we have chosen that we want to filter down to a specific post format, we create modify the
query
object so that we can tell it only to select only posts matching our chosen post format. certain posts. - We modify the
query
and change itstax_query
element so that it filters by post formats. We set thetaxonomy
topost_format
, thefields
toID
and then theincludes
to an array of$post_format
(which contains our value we want to filter by).
Once you have this code you will be able to filter your posts based on their post format. For example you may want to filter your posts to only show quotes
or chats
as shown below:
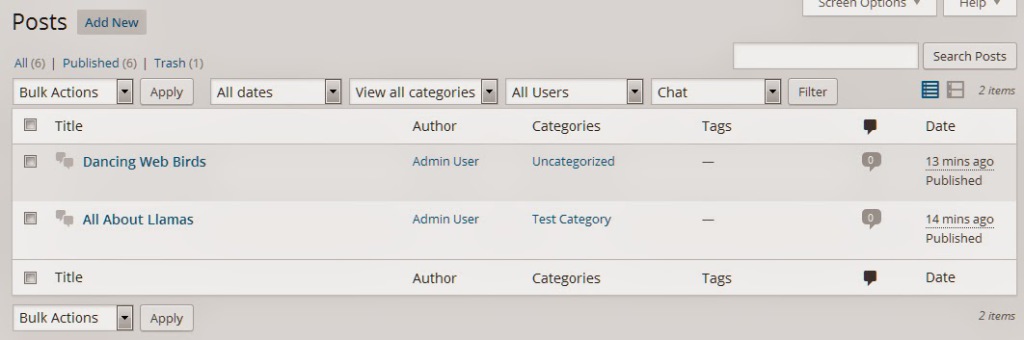
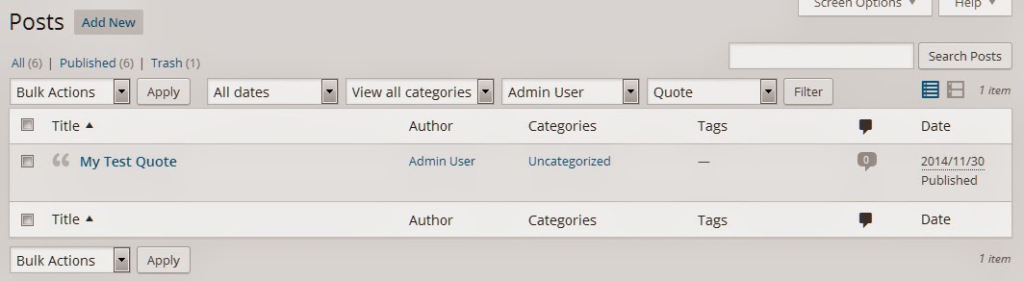
Filtering by Author
//restrict the posts by an additional author filter
function add_author_filter_to_posts_query($query){
global $post_type, $pagenow;
//if we are currently on the edit screen of the post type listings
if($pagenow == 'edit.php' && $post_type == 'post'){
if(isset($_GET['aurthor_admin_filter'])){
//set the query variable for 'author' to the desired value
$author_id = sanitize_text_field($_GET['aurthor_admin_filter']);
//if the author is not 0 (meaning all)
if($author_id != 0){
$query->query_vars['author'] = $author_id;
}
}
}
}
add_action('pre_get_posts','add_author_filter_to_posts_query');
Let us break this code down and run through exactly what it is we are doing
- We create a function called
add_author_filter_to_posts_query
and hook it onto thepre_get_posts
filter. This filter has access to the globalquery
variable so we also pass that into our function so we can manipulate it. This variable is passed by reference so we don’t need to return or echo any values, any changes to the query will stay. - We get the global
post_type
andpage_now
variables and check to seepage_now
is equal toedit.php
andpost_type
is equal topost
. Essentially we are checking to make sure we are on the post administration screen. - We determine if a post format has been selected by checking the
$_GET
variable. If it has been set, we collect its value. - We check to make sure that the entered value is not
0
as that is our default value. A value of0
represents the drop down filter option of ‘All Authors’ which would essentially mean that our filter does nothing. If our value is not0
we access thequery_vas
inside thequery
and set the value ofauthor
to our ID. Once this is set it will pull posts belonging only to that author id.
Once you have this code up and running you will be able to filter your posts by their author.
In my example site, I have two users admin user
and contributor user
. I can now choose to see posts only from a selected author as seen below:
In Conclusion
Now that you have a better understanding of WordPress administrative filters, you can use this tutorial and apply it to your own projects.
There are a wide range of already provided post attributes that you can filter by. In this tutorial we looked at filtering by the value of author
and the taxonomy post_format
, but you could easily filter by other values such as the ones listed on the WordPress Query class reference page.
Frequently Asked Questions (FAQs) about Customized WordPress Administration Filters
What are the benefits of using customized WordPress administration filters?
Customized WordPress administration filters offer several benefits. They allow you to modify the default behavior of WordPress, giving you more control over your website’s functionality. For instance, you can use filters to change the way your content is displayed, add or remove features, or even create new functionalities. This flexibility can greatly enhance your website’s user experience and functionality. Additionally, using filters can help you keep your code clean and organized, as you can make changes without directly editing the core WordPress files.
How do I add a new filter in WordPress?
Adding a new filter in WordPress involves using the add_filter() function. This function allows you to hook a custom function to a specific filter action. The add_filter() function requires two parameters: the name of the filter you want to hook the function to, and the name of the function you want to be called. Here’s a basic example:function my_custom_function($content) {
// Your code here
}
add_filter('the_content', 'my_custom_function');
In this example, ‘the_content’ is the name of the filter, and ‘my_custom_function’ is the name of the function that will be called.
Can I remove a filter in WordPress?
Yes, you can remove a filter in WordPress using the remove_filter() function. This function allows you to unhook a function from a filter hook. Like the add_filter() function, remove_filter() requires two parameters: the name of the filter hook and the name of the function to be removed. Here’s an example:remove_filter('the_content', 'my_custom_function');
In this example, ‘the_content’ is the name of the filter hook, and ‘my_custom_function’ is the name of the function to be removed.
How can I add custom filters to WordPress admin tables?
Adding custom filters to WordPress admin tables involves using the ‘restrict_manage_posts’ action hook. This hook allows you to add extra filtering options to the admin posts, pages, or custom post types list. Here’s a basic example:function my_custom_admin_filter() {
// Your code here
}
add_action('restrict_manage_posts', 'my_custom_admin_filter');
In this example, ‘restrict_manage_posts’ is the name of the action hook, and ‘my_custom_admin_filter’ is the name of the function that will be called.
What is the difference between actions and filters in WordPress?
Actions and filters are both types of hooks in WordPress, but they work in different ways. Actions are used to add or modify functionality, while filters are used to modify data. In other words, actions allow you to insert custom code at specific points in the WordPress execution process, while filters allow you to change data before it is sent to the browser or the database.
Can I use filters to modify the WordPress loop?
Yes, you can use filters to modify the WordPress loop. The loop is the main process in WordPress that displays your posts. By using filters, you can change the way your posts are displayed, the number of posts that are shown, the order in which they appear, and more. This can be a powerful way to customize your website’s appearance and functionality.
How can I test if a filter is working correctly?
Testing a filter involves checking if the expected changes are being applied. This can be done by viewing the affected part of your website and verifying that the changes you’ve made are visible. If the changes are not visible, there may be an issue with the filter’s code, or it may be conflicting with another filter or plugin.
Can I use filters to modify WordPress queries?
Yes, you can use filters to modify WordPress queries. The ‘posts_clauses’ filter, for example, allows you to modify the SQL clauses that are used to retrieve posts. This can be a powerful way to customize the way your content is retrieved and displayed.
Can I add multiple functions to a single filter hook?
Yes, you can add multiple functions to a single filter hook. Each function will be called in the order they were added. This can be useful if you want to apply multiple modifications to the same data.
Can I use filters to modify the WordPress admin interface?
Yes, you can use filters to modify the WordPress admin interface. For example, you can use the ‘admin_footer_text’ filter to change the footer text in the admin area, or the ‘login_redirect’ filter to change the page users are redirected to after logging in. This can be a great way to customize the admin area to better suit your needs.

Full stack developer and overall web enthusiast. I love everything to do with web / design and my passion revolves around creating awesome websites. Focusing primarily on WordPress, I create themes, plugins and bespoke solutions.