There’s an inevitable delay whenever your web application interacts with the server. That could be for an Ajax request, uploading a file, or using newer HTML5 APIs such as web sockets or server-sent events. Ideally, you should give the user some feedback to indicate their action is being processed. You’ll often see small animated GIFs with rotating patterns. There are several sites which will create them for you, such as preloaders.net and ajaxload.info. Images are the best cross-browser option but they have a number of issues:
- GIFs do not support alpha-transparency. You need to be careful when placing the image on a colored background.
- Bitmap images won’t scale nicely. If you change the dimensions, you need to create a new image.
- If you’re not careful, animated graphics can have a large file size.
- Images incur an additional HTTP request. While the image will be cached, the initial download may take longer than the background process it represents! You can code around this issue by pre-loading the image or using embedded data URLs but it’s more effort.
- A single HTML element, e.g.
<div id="ajaxloader"></div>
. - A few CSS backgrounds, border and shading effects to create a graphical icon.
- CSS3 transformations and animations to rotate or move the element.
Browser Compatibility
CSS3 transformations and animations are experimental properties which require vendor prefixes — and you know what trouble they cause. The example code implements the final property as well as prefixes for -webkit (Chrome and Safari), -moz (Firefox), -ms (IE), and -o (Opera), but there’s no guarantee they’ll work consistently if at all. At the time of writing, recent versions of Chrome, Safari and Firefox offer CSS3 animations. IE9/8/7/6 and Opera show a static image, although IE10 and Opera 12 may support the properties. Just to complicate matters further, Firefox allows you to animate pseudo elements separately. You can therefore add a couple of elements using :before and :after and rotate or move them in different directions to create more complex animations. While I’d initially hoped to do that, it doesn’t work in the webkit browsers. Chrome and Safari only allow real elements to be animated. It appears to be a bug or oversight, but it’s not been fixed in the current or beta releases.Creating the Icon
Our HTMLdiv
can be placed anywhere in the document although it might be best to append it as the last child of the body
. It will then appear above other elements and can be positioned in relation to the page.
The icon CSS simply sets wide white rounded border. The right border color is then set to transparent and a little shading is applied:
#ajaxloader
{
position: absolute;
width: 30px;
height: 30px;
border: 8px solid #fff;
border-right-color: transparent;
border-radius: 50%;
box-shadow: 0 0 25px 2px #eee;
}
The result:
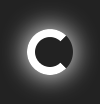
border-right: 0 none;
produces:
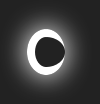
Animating the Icon
To make the icon spin and pulsate, we apply rotation transformations and opacity changes within a CSS3 animation. The animation name, duration, easing type and repeat are applied to the element:
#ajaxloader
{
animation: spin 1s linear infinite;
}
followed by the animation keyframes:
@keyframes spin
{
from { transform: rotate(0deg); opacity: 0.2; }
50% { transform: rotate(180deg); opacity: 1.0; }
to { transform: rotate(360deg); opacity: 0.2; }
}
None of the browsers support animation without vendor prefixes so you’ll see -webkit, -moz, -ms and -o alternatives within the source code when you view the demonstration page.
The icon can now be displayed using a little JavaScript whenever an Ajax request is initiated. It’s a great effect which can be customized easily and works on 55% of current browsers.
Unfortunately, 45% of web users won’t see the animation. That number will fall when IE10 is released and users switch to recent versions of other browsers, but it remains a large percentage. You could fall back to an image but, if you’re doing that, you might as well use the image for all browsers.
I therefore suggest you look at your own statistics. If your visitors are are predominately using Chrome, Safari and Firefox you could adopt the technique today. If not, stick with images for now and wait a little longer for more consistent browser support.
Frequently Asked Questions (FAQs) about CSS3 AJAX Loading Icons
How can I create a CSS3 AJAX loading icon?
Creating a CSS3 AJAX loading icon involves a combination of HTML, CSS, and JavaScript. First, you need to create a div element in your HTML file. This div will serve as the container for your loading icon. Next, you need to style this div using CSS. You can use CSS3 animations to create a spinning effect. Finally, you can use AJAX to show or hide the loading icon based on the state of your web page. AJAX allows you to update parts of your web page without reloading the whole page.
Can I customize the appearance of my CSS3 AJAX loading icon?
Yes, you can customize the appearance of your CSS3 AJAX loading icon. You can change the color, size, and animation speed of the icon using CSS. You can also use different shapes for your icon, such as circles, squares, or custom shapes defined using SVG.
How can I use a CSS3 AJAX loading icon in my web application?
To use a CSS3 AJAX loading icon in your web application, you need to include the HTML, CSS, and JavaScript code for the icon in your web page. You can then use AJAX to show or hide the icon based on the state of your web application. For example, you can show the icon when an AJAX request is in progress and hide it when the request is complete.
Can I use a CSS3 AJAX loading icon in a responsive design?
Yes, you can use a CSS3 AJAX loading icon in a responsive design. You can use CSS media queries to adjust the size and position of the icon based on the screen size. This ensures that the icon looks good on all devices, from smartphones to desktop computers.
How can I make my CSS3 AJAX loading icon accessible?
To make your CSS3 AJAX loading icon accessible, you can use ARIA attributes. ARIA stands for Accessible Rich Internet Applications and it helps to make web content and web applications more accessible to people with disabilities. For example, you can use the aria-busy attribute to indicate that an element is being updated and the aria-live attribute to announce updates to screen readers.
Can I use a CSS3 AJAX loading icon with jQuery?
Yes, you can use a CSS3 AJAX loading icon with jQuery. jQuery is a popular JavaScript library that simplifies HTML document traversal, event handling, and AJAX interactions. You can use jQuery’s AJAX methods to show or hide the loading icon based on the state of your AJAX requests.
How can I test my CSS3 AJAX loading icon?
You can test your CSS3 AJAX loading icon by simulating AJAX requests in your web page. You can use tools like Postman or the network tab in your browser’s developer tools to simulate AJAX requests. You can also use automated testing tools like Selenium or Jest to test the behavior of your loading icon.
Can I use a CSS3 AJAX loading icon in a single-page application (SPA)?
Yes, you can use a CSS3 AJAX loading icon in a single-page application (SPA). SPAs are web applications that load a single HTML page and dynamically update that page as the user interacts with the app. You can use AJAX to update parts of the page and show or hide the loading icon based on the state of these updates.
How can I optimize the performance of my CSS3 AJAX loading icon?
You can optimize the performance of your CSS3 AJAX loading icon by minimizing the amount of CSS and JavaScript code used for the icon. You can also use CSS3 animations instead of JavaScript animations, as they are generally more performant. Additionally, you can use tools like Google Lighthouse to measure the performance of your loading icon and identify areas for improvement.
Can I use a CSS3 AJAX loading icon in a progressive web app (PWA)?
Yes, you can use a CSS3 AJAX loading icon in a progressive web app (PWA). PWAs are web applications that use modern web capabilities to deliver an app-like experience to users. You can use AJAX to update parts of the app and show or hide the loading icon based on the state of these updates.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.