Sometimes to improve our website performance and usability we may want to add some keyboard shortcuts which can used to perform common site tasks faster than a speeding bullet! In this article I will explain in easy to understand n00b language how to create keyboard events using jQuery.
This is how you do it.// this is a generic setup to capture keyup events in jquery will console log for firebug
if(typeof console == 'undefined'){
console = {};
console.log = function(arg){return false;};
}
$(document).keyup(function(e){
//find out which key was pressed
switch(e.keyCode){
case 65 : console.log('a'); break; // a
case 66 : console.log('b'); break; // b
case 67 : console.log('c'); break; // c
case 68 : console.log('d'); break; // d
case 69 : console.log('e'); break; // e
case 70 : console.log('f'); break; // f
case 71 : console.log('g'); break; // g
case 72 : console.log('h'); break; // h
case 73 : console.log('i'); break; // i
case 74 : console.log('j'); break; // j
case 75 : console.log('k'); break; // k
case 76 : console.log('l'); break; // l
case 77 : console.log('m'); break; // m
case 78 : console.log('n'); break; // n
case 79 : console.log('o'); break; // o
case 80 : console.log('p'); break; // p
case 81 : console.log('q'); break; // q
case 82 : console.log('r'); break; // r
case 83 : console.log('s'); break; // s
case 84 : console.log('t'); break; // t
case 85 : console.log('u'); break; // u
case 86 : console.log('v'); break; // v
case 87 : console.log('w'); break; // w
case 88 : console.log('x'); break; // x
case 89 : console.log('y'); break; // y
case 90 : console.log('z'); break; // z
}
});
Frequently Asked Questions about Capturing Single Key Press
How can I capture a single key press using jQuery?
To capture a single key press using jQuery, you can use the keypress()
method. This method triggers the keypress event, or attaches a function to run when a keypress event occurs. Here’s a simple example:$(document).keypress(function(event){
alert('Key pressed: ' + String.fromCharCode(event.which));
});
In this code, $(document).keypress()
is the jQuery method that captures the key press event. The function(event)
is the function that runs when the keypress event occurs. The alert()
function is used to display an alert box with a specified message and an OK button.
What is the difference between keydown, keypress, and keyup events?
The keydown, keypress, and keyup events are all related to keyboard interactions, but they are triggered at different times. The keydown event is triggered the moment the key is pressed. However, it does not consider whether the key leads to a character being typed. The keypress event is triggered when a key that produces a character value is pressed. Unlike keydown, it does not trigger for keys like Shift, Esc, and others that do not produce a character. The keyup event is triggered when a key is released.
How can I prevent the default action of a keypress event?
To prevent the default action of a keypress event, you can use the event.preventDefault()
method in your event handler function. This method stops the default action of an element from happening. For example, if you want to prevent a form from submitting when the enter key is pressed, you can do something like this:$('form').keypress(function(event){
if(event.which == 13){
event.preventDefault();
alert('Form submission prevented!');
}
});
In this code, event.which == 13
checks if the key pressed is the enter key (the enter key has a key code of 13). If it is, event.preventDefault()
is called to prevent the form from submitting.
How can I detect which key was pressed?
You can detect which key was pressed by using the event.which
property in your event handler function. This property returns the Unicode value of the key that triggered the keypress event. For example:$(document).keypress(function(event){
alert('Key pressed: ' + String.fromCharCode(event.which));
});
In this code, event.which
returns the Unicode value of the key pressed, and String.fromCharCode(event.which)
converts that Unicode value into a string representing the corresponding character.
Can I use the keypress event to detect non-character keys?
No, the keypress event is only triggered when a key that produces a character value is pressed. This means it does not trigger for keys like Shift, Esc, and others that do not produce a character. If you need to detect non-character keys, you should use the keydown or keyup events instead.
How can I attach multiple event handlers to the keypress event?
You can attach multiple event handlers to the keypress event by calling the keypress()
method multiple times with different functions. For example:$(document).keypress(function(){
console.log('Handler 1');
});
$(document).keypress(function(){
console.log('Handler 2');
});
In this code, two event handlers are attached to the keypress event. When a keypress event occurs, both handlers will be executed in the order they were attached.
Can I trigger the keypress event manually?
Yes, you can trigger the keypress event manually by calling the keypress()
method without passing a function. For example:$(document).keypress();
This code will trigger the keypress event on the document object.
How can I remove an event handler from the keypress event?
You can remove an event handler from the keypress event by using the off()
method. This method removes event handlers that were attached with the on()
method. For example:$(document).off('keypress');
This code will remove all keypress event handlers from the document object.
Can I use the keypress event with form elements?
Yes, you can use the keypress event with form elements. In fact, it is commonly used with form elements to validate user input, prevent certain characters from being entered, and so on. For example:$('input').keypress(function(event){
if(event.which == 13){
event.preventDefault();
alert('Enter key pressed in input field!');
}
});
This code prevents the form from being submitted when the enter key is pressed in an input field.
Can I use the keypress event with other events?
Yes, you can use the keypress event with other events. For example, you might want to trigger a click event when a certain key is pressed. You can do this by calling the click()
method inside your keypress event handler function. For example:$(document).keypress(function(event){
if(event.which == 13){
$('#myButton').click();
}
});
This code triggers a click event on a button with the id “myButton” when the enter key is pressed.
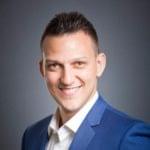
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.