Sass Functions to Kick-Start Your Style Sheets
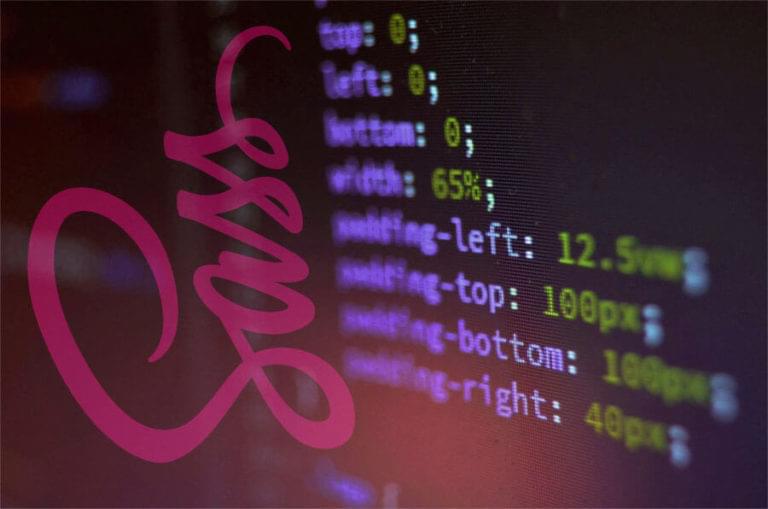
This is the updated version of an article originally published on November 11, 2014.
Sass has a number of built-in functions to help you set up the styles for your project a lot quicker and easier.
Let’s dive into a few of them!
Key Takeaways
- Sass has built-in functions that can significantly speed up the process of setting up styles for a project. Functions such as darken and lighten can adjust a color by a certain percentage, while opacify and transparentize can manipulate the opacity of colors. This helps maintain a consistent color palette throughout a project, even if the main brand color is changed midway through development.
- The complement Sass function is useful for creating visual contrast, as it returns the complementary color of any color input. The percentage function converts any number into a percentage, which can be useful for typographic or responsive value conversion.
- Sass also provides an inline if function, similar to the ternary operator in other programming languages. This allows for the creation of variable-dependent conditions in style sheets, and is particularly useful in responsive projects where properties may need to change based on variables that vary at different screen sizes or displays.
The Darken and Lighten Sass Functions
Possibly two of the best-known functions in this list, I’m going to count these as one because they do the same thing, but in different directions.
As the names suggest, darken
and lighten
will darken and lighten a color by a certain percentage respectively. You could use them on a button’s hover state or throughout a site to create hierarchy. Here’s how:
$main-color: #6dcff6;
$darker-color: darken($main-color, 20%);
$lighter-color: lighten($main-color, 20%);
The second argument in these two functions takes a percentage value by which to darken/lighten a color. This way you don’t have to look up the hex for a slightly lighter color every time you want an easy interaction state. For example, you could do this:
.brand-button {
background: $main-color;
}
.brand-button:hover {
background: $lighter-color;
}
.brand-button:visited {
background: $darker-color;
}
Which compiles into this:
.brand-button {
background: #6dcff6;
}
.brand-button:hover {
background: #cdeffc;
}
.brand-button:visited {
background: #0fafee;
}
Using these functions means that you could create an effective color palette that can remain consistent throughout your project. If, for instance, you have highlight and inactive state colors based off of a main brand color and your client decides to change their main color midway through development (it happens more than I care to admit…), you only have to change one value and see it cascade throughout the rest of a site.
The Opacify and Transparentize Sass Functions
Still sticking with colors, opacify
and transparentize
make colors more or less opaque respectively.
Personally, I’ve found these helpful when working with modals and other pop-ups where you might want the background content to fade out.
$main-color: rgba(0, 0, 0, 0.5);
$opaque-color: opacify($main-color, 0.5);
$transparent-color: transparentize($main-color, 0.3);
Unlike darken
and lighten
, the second argument in these two functions needs to be a decimal between 0 and 1 instead of a percentage. These functions are helpful for colors based off of a single, main brand color that permeates throughout a project. And again, by including these functions, you’re able to make a change to the main color alone and it will propagate accordingly.
You could use the values obtained with opacify
and transparentize
as follows:
.modal.focus {
background: $main-color;
}
.modal.blur {
background: $transparent-color;
}
.main-content {
background: $opaque-color;
}
Which will compile into this:
.modal.focus {
background: rgba(0, 0, 0, 0.5);
}
.modal.blur {
background: rgba(0, 0, 0, 0.2);
}
.main-content {
background: black;
}
You can also use fade-in
and fade-out
to create the same effect as these, as they’re aliases for opacify
and transparentize
.
The Complement Sass Function
As the name suggests, complement
will return the complement of any color that gets fed into it. This is especially useful if you’re trying to create a call-to-action on your page or view and need a bit of visual contrast. Using this built-in function alleviates the need to go and look the color up on a color wheel.
$main-color: #6dcff6;
$call-to-action: complement($main-color); //=> this will return #f6946d
The Percentage Sass Function
Sass’s numeric functions help you create your own functions and loops. The percentage
function, for instance, converts any number into a percentage, like so:
width: percentage(0.16) //=> this will return 16%
width: percentage(100px/50px); //=> this will return 200%
The percentage
function doesn’t even care if you shove units like px onto the values. This makes it a candidate for easy typographic or responsive value conversion, where percentages are king. What a nice guy!
The If Sass Function
Sass comes with the ability to write normal if statements using @if
. It does a great job in replicating what you’d find in a traditional programming language to create some fantastic conditional logic.
Sass also provides an inline if
function, which works much like the ternary operator in a number of other programming languages:
.foo {
width: if( 1 > 2, 400px, 500px); //=> this will return 500px
}
This function accepts three arguments, the first being the condition to check, the second being the output if the condition is true, and the third being the output if the condition is false.
You could use this ternary function if you wanted to create variable-dependent conditions (if the width of an article element is bigger than an aside element, for instance) in your style sheets, though the possibilities with this one are a little more open-ended.
Personally, I find if
more useful in responsive projects. You could use this function to change properties based on otherwise unrelated variables (like the font-size
of an element depending on the width
of its container), or even have properties rely on variables that change at different screen sizes or displays (like changing the background image of an element for retina screens).
Conclusion
Sass has a number of useful functions in its built-in arsenal, and these don’t even include the ones that Compass makes available to you, should you decide to use it. In the end, it’s about what kind of workflow makes sense to you, depending on your project, team and deadlines.
Are there any Sass or Compass functions you’ve come across that you think should be added to the list or that you simply find useful in your projects? Let us know in the comments below.
Frequently Asked Questions about Sass Functions
What are the different types of Sass functions?
Sass functions are powerful tools that allow you to perform computations and operations within your stylesheets. There are two types of Sass functions: built-in functions and user-defined functions. Built-in functions are pre-defined in Sass and include functions for manipulating colors, strings, numbers, lists, and maps. User-defined functions, on the other hand, are custom functions that you can create to perform specific tasks. They are defined using the @function rule and can accept any number of arguments.
How do I create a user-defined function in Sass?
Creating a user-defined function in Sass involves using the @function rule followed by the function name and a list of arguments in parentheses. The function body is then defined within curly braces. Here’s an example:@function calculate-rem($size) {
@return $size / 16px * 1rem;
}
This function takes a pixel value as an argument and returns its equivalent rem value.
How do I use Sass functions in my stylesheets?
To use a Sass function, you simply call it by its name followed by a list of arguments in parentheses. For example, if you have a function named calculate-rem, you can use it like this:font-size: calculate-rem(32px);
This will set the font size to the equivalent rem value of 32px.
Can I use Sass functions with CSS properties?
Yes, you can use Sass functions with any CSS property. The function will compute a value that can be used as the property value. This allows you to create dynamic styles that can adapt based on certain conditions or variables.
What are some common uses of Sass functions?
Sass functions are commonly used for tasks such as color manipulation, unit conversion, and value computation. For example, you can use the darken() function to create a darker version of a color, or the percentage() function to convert a decimal number to a percentage.
Can I use Sass functions in media queries?
Yes, Sass functions can be used in media queries. This can be particularly useful when you want to create responsive designs that adapt based on the viewport size. For example, you can create a function that calculates the appropriate font size based on the viewport width.
How do I debug a Sass function?
Sass provides a few built-in functions for debugging, such as inspect() and type-of(). These functions can help you understand what’s happening inside your functions and identify any issues.
Can I use Sass functions in other functions?
Yes, you can call a Sass function from within another function. This allows you to create complex computations and operations by combining multiple functions.
How do I handle errors in Sass functions?
Sass provides the @error directive that you can use to throw an error if something goes wrong in your function. The @error directive will stop the compilation and display an error message.
Can I use Sass functions in mixins?
Yes, you can use Sass functions in mixins. This allows you to create reusable chunks of code that can perform complex operations using functions.
Byron Houwens is a designer and developer who enjoys focusing on front end technologies and UX. He currently works as a front end developer in the burgeoning edtech environment, and lives in sunny Cape Town, South Africa.
Published in
·Content management·Design·Design & UX·Performance·Photography & Imagery·Resources·Technology·UX·October 13, 2015