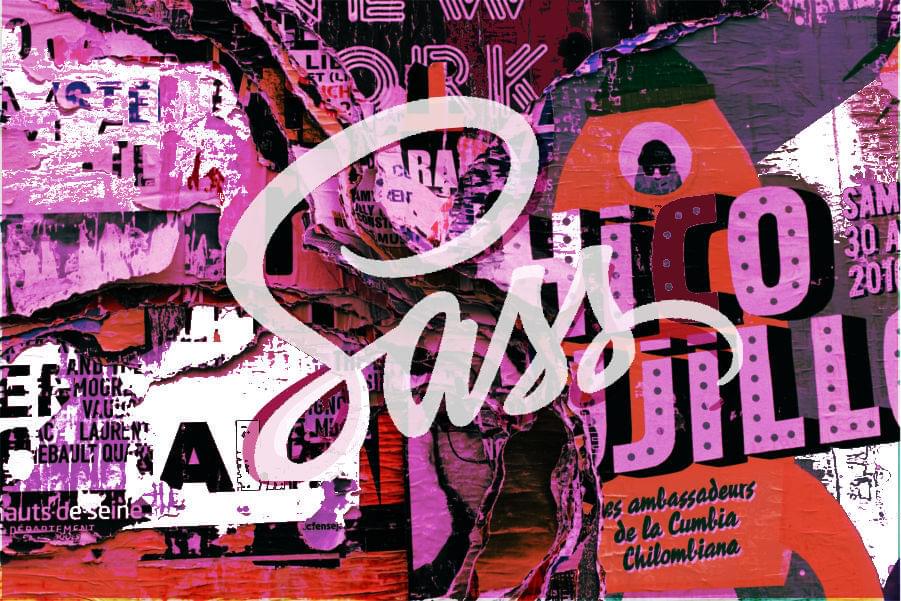
This is the updated version of an article first published on March 5, 2015.
Long ago, in the dense mists of the Internet’s past, intrepid adventurers tackled danger in much the same way: sling a fixed 960px layout, fight in a set grid and fire their typography in pixels to ward off evil.
Responsive web design has since changed all that by bringing us out of that dense mist and into an age of enlightenment. And yet, using typography on the web is still a pain at times. With the push from pixels or points to percentages and ems, I find myself continuously having to Google “pixel to percentange conversion chart” or something similar at the beginning of every project, and often even throughout.
In frustration, I finally turned to the power of Sass to forge a new, all-encompassing function in my quest to vanquish these problems, and today you’re going to build that function with me.
It’ll allow you to convert freely between pixels, em, and percentage values without having to consult a chart every time and, hopefully, alleviate a lot of your headaches in the process.
Setting Things Up for Typographic Units Conversion in Sass
Firstly, it’s extremely important to have a default font-size
defined in your CSS. Most browsers will default to 16px
, but if your project requires something different, make sure that your CSS knows about it. Also, most boilerplates come with 16px
defined as their default value, therefore I’m going to assume this as the default for this tutorial, too.
You then need to decide which units you’re going to support. Since this is likely to be helpful in a print to web environment, or even just a project which starts in Photoshop and ends up in the browser, you’ll be looking at pixels, points, ems and percentage.
You also want to give yourself the option of converting freely between them, so you can already say that your function needs at least three arguments:
@function convert($value, $currentUnit, $convertUnit) {}
The first argument is the font-size
number whose unit you wish to convert (for example 16), the second is the unit you’re planning to convert (for example pixels), and the third one is the desired unit you’re aiming for (like percentage). So, as an example, if you want to convert 16 pixels into a percentage value, you would do this:
.foo{
font-size: convert(16, px, percent);
}
Which will give you:
.foo{
font-size: 100%;
}
Let’s Beef It
Now, it’s time to tackle the bit that goes in between the braces.
Firstly, you want to be able to tackle pixels, ems, points and percentages, so you’ll need four statements to take care of them all.
If you were using a full-fledged programming language, you might use a switch statement. Since this is Sass, you’ll stick with if statements:
@function convert($value, $currentUnit, $convertUnit){
@if $currentUnit == px{
// stuff for pixels
}@else if $currentUnit == ems{
// stuff for ems
}@else if $currentUnit == percent{
// stuff for percentage
}@else if $currentUnit == pts{
// stuff for points
}
}
You now have an if statement for each possible input unit (whether you want pixels, ems, points or percentages to start with). So this is about 50% of the way there. All you have to do now is throw some awesome stuff into those if statements!
Throwing in the Maths for the Sass Typographic Units Conversion
Okay, so things get pretty mathematical at this point. Assuming you’re working with 16px as the default font-size
, you’ll have to convert it into ems and percentage like so:
@if $currentUnit == px{
@if $convertUnit == ems{
@return $value / 16 + 0em;
}
@else if $convertUnit == percent{
@return percentage($value / 16);
}
}
Again, you’re using one if statement per conversion (so one for ems, one for percentage) and then doing a little math to get the desired output. I’m not going to do a case for point values, since these only work with print CSS anyway.
With ems (and a default size of 16px), you just divide by 16 and add the “em” unit (+ 0em
).
Percentages with Sass are a little trickier. You can’t just throw a “%” at the end of the statement like you did with ems, as Sass will throw an error right back (something to the effect of “what are you doing putting that there!”). So here you need to incorporate Sass’s percentage function in order to return a valid percentage unit.
And with that, you have a function that converts pixels into ems or percentages! This is usually enough for a lot of developers, but let’s see how you can extend this function to cover ems to pixel conversion and percentage to pixel conversion:
@else if $currentUnit == ems{
@if $convertUnit == px{
@return $value * 16 + 0px;
}
@else if $convertUnit == percent{
@return percentage($value);
}
}
The math needs to change here for each statement, but that will sort out ems.
Next, here’s how you can convert percentages into pixels and into ems:
@else if $currentUnit == percent{
@if $convertUnit == px{
@return $value * 16 / 100 + 0px;
}
@else if $convertUnit == ems{
@return $value / 100 + 0em;
}
}
Finally it’s the turn of points to pixels, points to ems, and points to percentage conversions:
@else if $currentUnit == pts{
@if $convertUnit == px{
@return $value * 1.3333 + 0px;
}
@else if $convertUnit == ems{
@return $value / 12 + 0em;
}
@else if $convertUnit == percent{
@return percentage($value / 12)
}
}
And you’re done! You’ve created a function that lets you freely convert any value between any unit you want.
To Sum Up
The final function for typographic units conversion in Sass looks like this:
@function convert($value, $currentUnit, $convertUnit){
@if $currentUnit == px{
@if $convertUnit == ems{
@return $value / 16 + 0em;
}
@else if $convertUnit == percent{
@return percentage($value / 16);
}
}@else if $currentUnit == ems{
@if $convertUnit == px{
@return $value * 16 + 0px;
}
@else if $convertUnit == percent{
@return percentage($value);
}
}@else if $currentUnit == percent{
@if $convertUnit == px{
@return $value * 16 / 100 + 0px;
}
@else if $convertUnit == ems{
@return $value / 100 + 0em;
}
}@else if $currentUnit == pts{
@if $convertUnit == px{
@return $value * 1.3333 +0px;
}
@else if $convertUnit == ems{
@return $value / 12 + 0em;
}
@else if $convertUnit == percent{
@return percentage($value / 12)
}
}
}
It looks a little daunting, but all it really does is take the initial size, then convert it from the first unit into the second unit, and return the result. The only tough part is keeping track of what calculations to make.
If you want to play around with this function, you can do so in this Sassmeister demo.
As always, feel free to steal, mangle, rearrange and otherwise use this in whatever helps you the most when working with typography on the web.
You could easily expand on the existing function, maybe including something like rem unit conversion, error handling for inputs that won’t work, or setting default units that you use all the time.
If you have any other awesome ideas for this Sass units conversion function, let us know in the comments section below.
Frequently Asked Questions (FAQs) on Converting Typographic Units with SASS
What is the difference between CSS and SASS?
CSS (Cascading Style Sheets) is a style sheet language used for describing the look and formatting of a document written in HTML or XML. SASS (Syntactically Awesome Style Sheets), on the other hand, is a preprocessor scripting language that is interpreted or compiled into CSS. SASS provides features like variables, nesting, and mixins, which make the CSS more readable and easier to maintain. It also supports mathematical operations, functions, and other high-level programming constructs.
How do I convert CSS to SASS?
Converting CSS to SASS is a straightforward process. You can use online tools like CSS to SASS converter, which allows you to paste your CSS code and get the equivalent SASS code. Alternatively, you can manually convert your CSS code to SASS by following the SASS syntax. For instance, you can replace the CSS selectors with nested selectors in SASS, use variables instead of repeating the same values, and use mixins for reusable styles.
Can I use SASS in my existing CSS project?
Yes, you can use SASS in your existing CSS project. SASS is fully compatible with all versions of CSS. So, you can gradually migrate your CSS code to SASS. You can start by renaming your .css files to .scss, which is the file extension for SASS files. Then, you can refactor your CSS code to take advantage of the features provided by SASS.
How do I compile SASS to CSS?
To compile SASS to CSS, you need to install a SASS compiler. There are many SASS compilers available, such as Dart Sass, Node Sass, and Ruby Sass. Once you have installed a SASS compiler, you can run a command in your terminal or command prompt to compile your SASS files to CSS. The command usually looks like this: sass input.scss output.css
.
What are the benefits of using SASS over CSS?
SASS provides several benefits over CSS. It allows you to use variables, which can help you avoid repeating the same values in your stylesheets. It also supports nesting, which can make your code more readable and easier to maintain. Moreover, SASS provides features like mixins and functions, which can help you write reusable and modular code. Lastly, SASS allows you to split your code into multiple files, which can make it easier to organize and manage your stylesheets.
What are typographic units in SASS?
Typographic units in SASS are units of measurement used for typography. They include units like pixels (px), points (pt), ems (em), and rems (rem). These units allow you to specify the size, spacing, and other typographic properties of your text.
How do I convert typographic units in SASS?
To convert typographic units in SASS, you can use the built-in functions provided by SASS. For instance, you can use the px-to-em
function to convert pixels to ems, or the pt-to-rem
function to convert points to rems. You can also create your own functions to convert between other typographic units.
Can I use CSS functions in SASS?
Yes, you can use CSS functions in SASS. In fact, SASS supports all CSS functions and adds several additional functions. These functions allow you to perform calculations, manipulate colors, and do other operations in your stylesheets.
How do I use variables in SASS?
To use variables in SASS, you need to declare a variable with a = sign followed by the variable name. Then, you can assign a value to the variable. Once you have declared a variable, you can use it in your stylesheets by referencing the variable name. For instance, you can declare a variable for a color like this:
$primary-color: #ff0000;, and then use it in your styles like this:
color: $primary-color;`.
What are mixins in SASS and how do I use them?
Mixins in SASS are reusable blocks of styles that you can include in your stylesheets. To create a mixin, you use the @mixin
directive followed by the mixin name and a block of styles. Then, you can include the mixin in your styles using the @include
directive followed by the mixin name. For instance, you can create a mixin for a button style like this: @mixin button-style { background-color: #ff0000; color: #ffffff; }
, and then include it in your styles like this: .button { @include button-style; }
.
Byron Houwens is a designer and developer who enjoys focusing on front end technologies and UX. He currently works as a front end developer in the burgeoning edtech environment, and lives in sunny Cape Town, South Africa.