jquery validation for dob to specific format ie dd-mm-yy
$.validator.addMethod("dateFormat",
function(value, element) {
return value.match(/^dd?-dd?-dd$/);
},
"Please enter a date in the format dd-mm-yyyy.");
And then on your form add:
$('#myForm')
.validate({
rules : {
myDate : {
dateFormat: true
}
}
});
Frequently Asked Questions (FAQs) on jQuery Validate Date of Birth Format
How Can I Validate a Date in the Format DD-MM-YYYY Using jQuery Validate?
To validate a date in the format DD-MM-YYYY using jQuery Validate, you need to add a custom method to the jQuery Validate plugin. This method will check if the input date is in the correct format. Here is a simple code snippet that demonstrates this:$.validator.addMethod("dateITA", function(value, element) {
var check = false;
var re = /^\d{1,2}-\d{1,2}-\d{4}$/;
if( re.test(value)){
var adata = value.split('-');
var gg = parseInt(adata[0],10);
var mm = parseInt(adata[1],10);
var aaaa = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Use jQuery Validate to Check if the Date of Birth Makes the User at Least 18 Years Old?
To use jQuery Validate to check if the date of birth makes the user at least 18 years old, you can add a custom method that calculates the age based on the input date. Here is a code snippet that demonstrates this:$.validator.addMethod("minAge", function(value, element, min) {
var today = new Date();
var birthDate = new Date(value);
var age = today.getFullYear() - birthDate.getFullYear();
if (age < min+1) {
return false;
}
return true;
}, "You are not old enough!");
How Can I Validate a Date of Birth in the Format MM-DD-YYYY Using jQuery Validate?
To validate a date of birth in the format MM-DD-YYYY using jQuery Validate, you can use a similar approach as for the DD-MM-YYYY format, but adjust the order of the date parts in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateMDY", function(value, element) {
var check = false;
var re = /^\d{1,2}-\d{1,2}-\d{4}$/;
if( re.test(value)){
var adata = value.split('-');
var mm = parseInt(adata[0],10);
var gg = parseInt(adata[1],10);
var aaaa = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format YYYY-MM-DD Using jQuery Validate?
To validate a date of birth in the format YYYY-MM-DD using jQuery Validate, you can use a similar approach as for the other formats, but adjust the order of the date parts in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateYMD", function(value, element) {
var check = false;
var re = /^\d{4}-\d{1,2}-\d{1,2}$/;
if( re.test(value)){
var adata = value.split('-');
var aaaa = parseInt(adata[0],10);
var mm = parseInt(adata[1],10);
var gg = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format DD/MM/YYYY Using jQuery Validate?
To validate a date of birth in the format DD/MM/YYYY using jQuery Validate, you can use a similar approach as for the other formats, but adjust the regular expression and the separator in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateDMY", function(value, element) {
var check = false;
var re = /^\d{1,2}\/\d{1,2}\/\d{4}$/;
if( re.test(value)){
var adata = value.split('/');
var gg = parseInt(adata[0],10);
var mm = parseInt(adata[1],10);
var aaaa = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format MM/DD/YYYY Using jQuery Validate?
To validate a date of birth in the format MM/DD/YYYY using jQuery Validate, you can use a similar approach as for the other formats, but adjust the regular expression and the separator in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateMDY", function(value, element) {
var check = false;
var re = /^\d{1,2}\/\d{1,2}\/\d{4}$/;
if( re.test(value)){
var adata = value.split('/');
var mm = parseInt(adata[0],10);
var gg = parseInt(adata[1],10);
var aaaa = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format YYYY/MM/DD Using jQuery Validate?
To validate a date of birth in the format YYYY/MM/DD using jQuery Validate, you can use a similar approach as for the other formats, but adjust the regular expression and the separator in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateYMD", function(value, element) {
var check = false;
var re = /^\d{4}\/\d{1,2}\/\d{1,2}$/;
if( re.test(value)){
var adata = value.split('/');
var aaaa = parseInt(adata[0],10);
var mm = parseInt(adata[1],10);
var gg = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format DD.MM.YYYY Using jQuery Validate?
To validate a date of birth in the format DD.MM.YYYY using jQuery Validate, you can use a similar approach as for the other formats, but adjust the regular expression and the separator in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateDMY", function(value, element) {
var check = false;
var re = /^\d{1,2}\.\d{1,2}\.\d{4}$/;
if( re.test(value)){
var adata = value.split('.');
var gg = parseInt(adata[0],10);
var mm = parseInt(adata[1],10);
var aaaa = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format MM.DD.YYYY Using jQuery Validate?
To validate a date of birth in the format MM.DD.YYYY using jQuery Validate, you can use a similar approach as for the other formats, but adjust the regular expression and the separator in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateMDY", function(value, element) {
var check = false;
var re = /^\d{1,2}\.\d{1,2}\.\d{4}$/;
if( re.test(value)){
var adata = value.split('.');
var mm = parseInt(adata[0],10);
var gg = parseInt(adata[1],10);
var aaaa = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
How Can I Validate a Date of Birth in the Format YYYY.MM.DD Using jQuery Validate?
To validate a date of birth in the format YYYY.MM.DD using jQuery Validate, you can use a similar approach as for the other formats, but adjust the regular expression and the separator in the code. Here is a code snippet that demonstrates this:$.validator.addMethod("dateYMD", function(value, element) {
var check = false;
var re = /^\d{4}\.\d{1,2}\.\d{1,2}$/;
if( re.test(value)){
var adata = value.split('.');
var aaaa = parseInt(adata[0],10);
var mm = parseInt(adata[1],10);
var gg = parseInt(adata[2],10);
var xdata = new Date(aaaa,mm-1,gg);
if ( ( xdata.getFullYear() == aaaa ) && ( xdata.getMonth () == mm - 1 ) && ( xdata.getDate() == gg ) )
check = true;
else
check = false;
} else
check = false;
return this.optional(element) || check;
}, "Please enter a correct date");
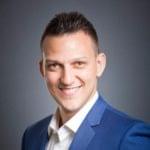
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.