Bascially in a nutshell you can use Live and Toggle together BUT for coding best practices you shouldn’t. Both jQuery .Live() and jQuery .Toggle() automatically create thier own bind events to the element. This causes problems when they are used together as you would need to click the button twice to get the Toggle to work. A way around this is to use a Live(‘click’) event with inside Toggle event you can add the .Trigger(‘click’) command to the end of the function call like this:
// Add sort functions on dynamic elements inserted into DOM
$('.sort').live('click',JQUERY4U.sortClickListener);
[code lang="js"]
//function inside JQUERY4U namespace
sortClickListener: function(){
// Find second class name
var button = $(this).attr('class').split(' ');
// Sort table
$(this).toggle(function() {
$('.item').tsort('.'+button[1],{order:'desc'});
}, function() {
$('.item').tsort('.'+button[1]);
}).trigger('click'); /*force the button to work with 1 click*/
},
Another way would be to use .Data method but this would be overkill and a long winded way of solving the puzzle.
$(".reply").live('click', function () {
var toggled = $(this).data('toggled');
$(this).data('toggled', !toggled);
if (!toggled) {
x1();
}
else {
x2();
}
});
Frequently Asked Questions (FAQs) about jQuery Toggle
What is the difference between jQuery toggle() and toggleClass()?
The jQuery toggle() function is used to alternate between hide() and show() for the selected elements. This means that if the element is initially displayed, it will be hidden and vice versa. On the other hand, the toggleClass() function is used to add or remove one or more classes from the selected elements. This means that if the specified class(es) exist, it will be removed and if it does not exist, it will be added.
How can I use jQuery toggle() to show or hide multiple elements?
You can use the jQuery toggle() function to show or hide multiple elements by selecting them using a common class or attribute. For example, if you have multiple div elements with the class ‘myDiv’, you can toggle their visibility using $(‘.myDiv’).toggle().
Can I use jQuery toggle() with animations?
Yes, you can use the jQuery toggle() function with animations. The toggle() function can take two parameters: duration and callback. The duration parameter specifies the duration of the animation, and the callback parameter is a function to be executed after the toggle() function completes.
Why is my jQuery toggle() function not working?
There could be several reasons why your jQuery toggle() function is not working. Some common issues include: the jQuery library is not properly included in your project, the element you are trying to select does not exist or the CSS display property of the element is set to ‘none’.
How can I use jQuery toggle() to change the text of a button?
You can use the jQuery toggle() function in combination with the text() or html() function to change the text of a button. For example, you can create a function that changes the text of a button when it is clicked using the toggle() function.
Can I use jQuery toggle() with hover?
Yes, you can use the jQuery toggle() function with hover. However, since the hover event is not a standard JavaScript event, you will need to use the mouseenter and mouseleave events instead. You can use the toggle() function in the callback function of these events to show or hide an element when the mouse pointer enters or leaves it.
How can I use jQuery toggle() with checkboxes?
You can use the jQuery toggle() function with checkboxes to show or hide an element based on the checked state of the checkbox. You can use the change event of the checkbox to call the toggle() function whenever the checked state changes.
Can I use jQuery toggle() with radio buttons?
Yes, you can use the jQuery toggle() function with radio buttons. Similar to checkboxes, you can use the change event of the radio button to call the toggle() function whenever the selected radio button changes.
How can I use jQuery toggle() with dropdown menus?
You can use the jQuery toggle() function with dropdown menus to show or hide the dropdown menu when the dropdown button is clicked. You can use the click event of the dropdown button to call the toggle() function.
Can I use jQuery toggle() with forms?
Yes, you can use the jQuery toggle() function with forms to show or hide form elements based on user input. For example, you can show or hide a text input field based on the selected option of a dropdown menu using the toggle() function.
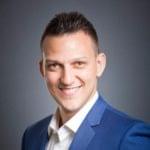
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.