jQuery Code Snippet to get the alt tags of each image and set the title tag so it is the same as the alt tag. Could be useful to improve SEO for the web page and added support for browsers such as Firefox that utilise image title tags.
jQuery(document).ready(function($) {
$("img:not([title])").each(function() {
if($(this).attr("alt") != '') $(this).parent().attr("title", $(this).attr("alt"))
else $(this).parent().attr("title", $(this).attr("src"));
});
});
Frequently Asked Questions about jQuery, Image Title and Alt Tag
How can I set an image title and alt tag using jQuery?
To set an image title and alt tag using jQuery, you need to use the .attr() method. This method is used to get the attribute value for only the first element in the matched set. It returns undefined for values of undefined elements. Here is a simple example of how you can use it:$('img').attr({
'alt': 'Alt Tag',
'title': 'Title Tag'
});
In this example, ‘img’ is the selector, ‘alt’ and ‘title’ are the attributes, and ‘Alt Tag’ and ‘Title Tag’ are the values you want to set for these attributes.
What is the importance of alt tags and title tags for SEO?
Alt tags and title tags play a crucial role in SEO. The alt tag, also known as “alt attribute” and “alt description,” is used within an HTML code to describe the appearance and function of an image on a page. It helps search engines understand what an image is about, which can help it rank in Google Images. It’s also used for web accessibility.
On the other hand, the title tag is displayed on mouse hover in most browsers and used by search engines when displaying search results. It gives users and search engines an accurate and concise description of your page’s content.
How can I get the alt attribute from an image that resides inside an ‘a’ tag using jQuery?
To get the alt attribute from an image that resides inside an ‘a’ tag, you can use the .find() method in combination with the .attr() method. Here’s an example:$('a').find('img').attr('alt');
In this example, ‘a’ is the selector for the anchor tag, .find(‘img’) is used to find the image inside the ‘a’ tag, and .attr(‘alt’) is used to get the alt attribute of the image.
Can I use jQuery to replace the title attribute with the alt attribute for an image?
Yes, you can use jQuery to replace the title attribute with the alt attribute for an image. Here’s how you can do it:$('img').attr('title', function() {
return $(this).attr('alt');
});
In this example, the function returns the alt attribute of the image, which is then set as the new value for the title attribute.
How can I add alt text to an image for better SEO?
To add alt text to an image for better SEO, you can use the .attr() method in jQuery. Here’s an example:$('img').attr('alt', 'Your Alt Text Here');
In this example, ‘img’ is the selector for the image, ‘alt’ is the attribute you want to set, and ‘Your Alt Text Here’ is the alt text you want to add to the image. Remember, the alt text should accurately describe the image content for the best SEO results.
How can I remove the alt attribute from an image using jQuery?
To remove the alt attribute from an image using jQuery, you can use the .removeAttr() method. Here’s an example:$('img').removeAttr('alt');
In this example, ‘img’ is the selector for the image, and ‘alt’ is the attribute you want to remove.
Can I use jQuery to add a title attribute to an ‘a’ tag?
Yes, you can use jQuery to add a title attribute to an ‘a’ tag. Here’s how you can do it:$('a').attr('title', 'Your Title Here');
In this example, ‘a’ is the selector for the anchor tag, ‘title’ is the attribute you want to set, and ‘Your Title Here’ is the title you want to add to the ‘a’ tag.
How can I use jQuery to change the alt attribute of an image?
To change the alt attribute of an image using jQuery, you can use the .attr() method. Here’s an example:$('img').attr('alt', 'New Alt Text Here');
In this example, ‘img’ is the selector for the image, ‘alt’ is the attribute you want to change, and ‘New Alt Text Here’ is the new alt text you want to set.
Can I use jQuery to get the title attribute of an ‘a’ tag?
Yes, you can use jQuery to get the title attribute of an ‘a’ tag. Here’s how you can do it:$('a').attr('title');
In this example, ‘a’ is the selector for the anchor tag, and .attr(‘title’) is used to get the title attribute of the ‘a’ tag.
How can I use jQuery to remove the title attribute from an ‘a’ tag?
To remove the title attribute from an ‘a’ tag using jQuery, you can use the .removeAttr() method. Here’s an example:$('a').removeAttr('title');
In this example, ‘a’ is the selector for the anchor tag, and ‘title’ is the attribute you want to remove.
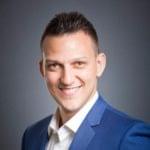
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.