Simple jQuery code snippet to replace all the text on the place with any given string. Could be useful for changing company names based on dynamic variables which may change. Important: don’t forget that the replace() function returns a value and does not do a live update on the page. In order to do this you simply assign the page element with the result of the .replace().
//ie to replace all dots on page with hyphens
var replace_str = $('body').html().replace(/./g,'-');
$('body').html(replace_str);
//if that doesn't work try this
var strNewString = $('body').html().replace(/./g,'---');
$('body').html(strNewString);
Frequently Asked Questions (FAQs) about jQuery Replace Strings
How can I use jQuery to replace a string in HTML content?
jQuery provides a simple and efficient way to replace a string in HTML content. You can use the .html() method combined with the JavaScript replace() function. First, you need to select the HTML element using a jQuery selector. Then, use the .html() method to get the HTML content. Apply the replace() function to this content, specifying the string to be replaced and the new string. Finally, use the .html() method again to update the HTML content with the new string. Here’s an example:$("p").html(function(index, oldHtml){
return oldHtml.replace("old string", "new string");
});
In this example, “old string” is the string you want to replace and “new string” is the string you want to replace it with.
Can I replace multiple instances of a string using jQuery?
Yes, you can replace multiple instances of a string using jQuery. However, the JavaScript replace() function only replaces the first instance of the string. To replace all instances, you need to use a regular expression with the ‘g’ flag (global match). Here’s an example:$("p").html(function(index, oldHtml){
var regex = new RegExp("old string", "g");
return oldHtml.replace(regex, "new string");
});
In this example, all instances of “old string” in the paragraph will be replaced with “new string”.
How can I replace a string in a specific part of my HTML document?
You can replace a string in a specific part of your HTML document by using a more specific jQuery selector. For example, if you want to replace a string in a paragraph with a certain class, you can use a class selector like $(“.myClass”). Here’s an example:$(".myClass").html(function(index, oldHtml){
var regex = new RegExp("old string", "g");
return oldHtml.replace(regex, "new string");
});
In this example, all instances of “old string” in paragraphs with the class “myClass” will be replaced with “new string”.
Can I use jQuery to replace a string in an attribute value?
Yes, you can use jQuery to replace a string in an attribute value. You can use the .attr() method to get and set attribute values. Here’s an example:$("img").attr("src", function(index, oldSrc){
var regex = new RegExp("old.jpg", "g");
return oldSrc.replace(regex, "new.jpg");
});
In this example, all instances of “old.jpg” in the “src” attribute of image tags will be replaced with “new.jpg”.
Can I replace a string with HTML content using jQuery?
Yes, you can replace a string with HTML content using jQuery. When you use the .html() method to set HTML content, any string can be replaced with HTML. Here’s an example:$("p").html(function(index, oldHtml){
var regex = new RegExp("old string", "g");
return oldHtml.replace(regex, "<span>new string</span>");
});
In this example, all instances of “old string” in the paragraph will be replaced with the HTML content “new string“.
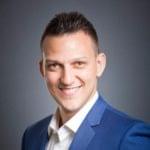
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.