Expanding on my previous post about detecting and removing broken images I have delved further into the realms of using jQuery to replace broken images using AJAX. In most browsers the ALT tag is shown if the image is not found. This could be a problem if the image is small and the ALT tag is long as it seems the output width of the element is not forced by the length of the alt tag. So it makes sense to replace broken images with a default image.
Get information about the current images on page
$("img").each( function () {
console.log($(this).attr('src')+ ' ' + $(this).attr('alt') + ' ' + $(this).width());
});
Using AJAX to test if the image exists
$("img").each( function ()
{
$.ajax({
url:$(this).attr('src'),
type:'HEAD',
error:
function(){
//image doesn't exist
console.log('ERROR');
},
success:
function(){
//image exists
console.log('success');
}
});
});
/*
Output:
success
success
ERROR
success
success
*/
Refresh image
d = new Date();
$("#myimg").attr("src", "/myimg.jpg?"+d.getTime()); //cache flush
Fix broken images using ajax
Note the additions of _this and e.status.$(".productBoxImage img").each( function ()
{
var _this = $(this);
$.ajax({
url:$(this).attr('src'),
type:'HEAD',
async: false,
error:
function(e)
{
if (e.status == '404') {
$(_this).attr('src',[replaceImageUrl]);
}
}
});
});
Non ajax function version
/**
* Returns true if image is broken, false otherwise
* @param {jQuery} image A single image element
* @return {Boolean}
*/
isImageBroken: function(image)
{
$image = $(image);
if($image.attr('complete') == false || $image.attr('naturalWidth') == 0 || $image.attr('readyState') == 'uninitialized' || this.trim($image.attr('src')) == '')
{
return true;
}
return false;
},
Hope that all made sense, if not post a comment and i’ll answer your question! :)
Frequently Asked Questions (FAQs) about Fixing Broken Images Automatically in jQuery
What is the basic concept behind fixing broken images in jQuery?
The basic concept behind fixing broken images in jQuery is to use the error event. This event is triggered when an error occurs while loading an external file, such as an image. In the context of images, we can use this event to replace the source of the image (src attribute) with a default or placeholder image whenever the original image fails to load.
How can I use the error event in jQuery to fix broken images?
To use the error event in jQuery, you need to select the images and then bind the error event to them. Inside the event handler function, you can change the source of the image to a default image. Here’s a basic example:$('img').error(function(){
$(this).attr('src', 'default.jpg');
});
Can I use a specific image as a replacement for each broken image?
Yes, you can use a specific image as a replacement for each broken image. You just need to modify the src attribute inside the error event handler function. For example, if you want to replace a broken image with an image named ‘specific.jpg’, you can do it like this:$('img').error(function(){
$(this).attr('src', 'specific.jpg');
});
Is it possible to use a different replacement image for each broken image?
Yes, it’s possible to use a different replacement image for each broken image. You can achieve this by using a function to determine the replacement image based on certain conditions. For example, you can use the alt attribute of the image to determine the replacement image.
How can I use the alt attribute of the image to determine the replacement image?
You can use the alt attribute of the image to determine the replacement image by accessing it inside the error event handler function. Here’s an example:$('img').error(function(){
var alt = $(this).attr('alt');
$(this).attr('src', alt + '.jpg');
});
What if I want to use a default image only if the replacement image also fails to load?
If you want to use a default image only if the replacement image also fails to load, you can bind the error event to the images again inside the error event handler function. Here’s an example:$('img').error(function(){
var alt = $(this).attr('alt');
$(this).attr('src', alt + '.jpg').error(function(){
$(this).attr('src', 'default.jpg');
});
});
Can I use this method to fix broken images in a specific part of the page?
Yes, you can use this method to fix broken images in a specific part of the page. You just need to modify the selector to select the images inside that part. For example, if you want to fix the images inside a div with the id ‘content’, you can do it like this:$('#content img').error(function(){
$(this).attr('src', 'default.jpg');
});
Is there a way to know if an image has been replaced?
Yes, there’s a way to know if an image has been replaced. You can add a class to the image inside the error event handler function. Then, you can use this class to style the replaced images or to select them for further processing. Here’s an example:$('img').error(function(){
$(this).attr('src', 'default.jpg').addClass('replaced');
});
Can I use this method to fix broken images that are added to the page dynamically?
Yes, you can use this method to fix broken images that are added to the page dynamically. You just need to use the on method instead of the error method to bind the error event. This method allows you to bind events to elements that are added to the page after the event handlers are set. Here’s an example:$(document).on('error', 'img', function(){
$(this).attr('src', 'default.jpg');
});
What if I want to do something else when an image fails to load, besides replacing it?
If you want to do something else when an image fails to load, besides replacing it, you can add that code inside the error event handler function. For example, you can log a message to the console like this:$('img').error(function(){
console.log('An image failed to load: ' + $(this).attr('src'));
$(this).attr('src', 'default.jpg');
});
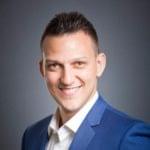
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.