jQuery code snippet to get the relative position of the mouse pointer. The function takes in the element id as a parameter and the current x and y co-ordinates of the mouse pointer. It then returns the relative distances between the mouse’s cursors current position and the specified element.
function rPosition(elementID, mouseX, mouseY) {
var offset = $('#'+elementID).offset();
var x = mouseX - offset.left;
var y = mouseY - offset.top;
return {'x': x, 'y': y};
}
Example Usage
jQuery(document).ready(function($) {
//get the current x and y of the mouse pointer
var X = $('body').offset().left;
var Y = $('body').offset().top;
mouseX = ev.pageX - X;
mouseY = ev.pageY - Y;
//get the relative position to the #eid element on the page
alert(rPosition('eid',x,y));
});
Frequently Asked Questions (FAQs) about jQuery Relative Mouse Position
How can I get the mouse position in jQuery without using mouse events?
In jQuery, mouse events are typically used to get the mouse position. However, if you want to get the mouse position without using mouse events, you can use the .offset()
method. This method returns the offset coordinates of the first element in the set of matched elements, relative to the document. Here’s a simple example:var position = $("#element").offset(); // returns an object containing the properties top and left
console.log("The image is located at: " + position.left + "," + position.top);
How can I get the mouse position within an element using jQuery?
To get the mouse position within an element, you can use the .offset()
method in combination with the pageX and pageY properties of the event object. Here’s an example:$("#element").mousemove(function(event) {
var parentOffset = $(this).offset();
var relX = event.pageX - parentOffset.left;
var relY = event.pageY - parentOffset.top;
console.log("X Coordinate: " + relX + " Y Coordinate: " + relY);
});
What is the use of the mousemove()
method in jQuery?
The mousemove()
method in jQuery is used to attach an event handler function for the mousemove
event, or trigger that event on an element. This event is sent to an element when the mouse pointer moves inside the element. It can be used to track the movement of the mouse and perform actions based on the mouse’s position.
How can I get the position of the mouse pointer in jQuery?
You can get the position of the mouse pointer in jQuery using the event.pageX
and event.pageY
properties. These properties provide the position of the mouse pointer, relative to the left edge and top edge of the document, respectively.
How can I find the mouse position relative to an element using jQuery?
To find the mouse position relative to an element, you can subtract the position of the element from the position of the mouse pointer. Here’s an example:$("#element").mousemove(function(event) {
var x = event.pageX - $(this).offset().left;
var y = event.pageY - $(this).offset().top;
console.log("X Coordinate: " + x + " Y Coordinate: " + y);
});
This will give you the position of the mouse pointer relative to the specified element.
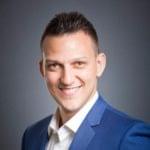
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.