This is how you can generate data for use with AJAX using a “POST” method which contains JSON data and then pass it to a PHP script and then decode ready to use as variables (name value pairs). In this example, I’ve used form input values to generate the data passed as a JSON string, but you can create your own JSON data to pass doesn’t have to be from a form.
jQuery / AJAX
Basic AJAX function to pass the JSON data to the server-side script.$.ajax({
type: "POST",
url: targetURL,
async: false,
data: JSON.stringify($('#form').serializeArray()),
success: function(data){
console.log(data);
return true;
},
complete: function() {},
error: function(xhr, textStatus, errorThrown) {
console.log('ajax loading error...');
return false;
}
});
If we take a look at the generated JSON is has name value pairs.
Generated JSON example:
data=[{"name":"product","value":"riserva shiraz wine glass"},{"name":"supid","value":"81"},{"name":"brandid","value":"60"},{"name":"blid","value":"7"},{"name":"cid","value":"381"}];
PHP Variable Dynamics
// decode JSON string to PHP object, 2nd param sets to associative array
$decoded = json_decode($_GET['data'],true);
output values:
foreach ($decoded as $value) {
echo $value["name"] . "=" . $value["value"];
}
//set values:
foreach ($decoded as $value) {
$$value["name"] = $value["value"];
}
//both:
foreach ($decoded as $value) {
$$value["name"] = $value["value"];
echo $value["name"] . "=" . $$value["name"];
echo "";
}
Sorry, no demo, but feel free to ask questions.
Frequently Asked Questions (FAQs) about jQuery, PHP, AJAX, and JSON
How can I handle errors in jQuery AJAX calls to PHP scripts?
Error handling is crucial in any programming task, including jQuery AJAX calls to PHP scripts. You can handle errors by using the .fail() method in jQuery. This method is called when the request fails. It takes a function as an argument, which will be executed when an error occurs. Inside this function, you can write code to handle the error. For instance, you can display an error message to the user.
How can I send multiple data using jQuery AJAX to a PHP script?
To send multiple data using jQuery AJAX to a PHP script, you can use an object literal in the data option of the $.ajax() method. The object literal contains key-value pairs where the key is the name of the variable and the value is the value of the variable. The PHP script can then access these variables using the $_POST or $_GET array, depending on the type of the AJAX request.
How can I use the JSON data returned from a PHP script in jQuery?
When a PHP script returns JSON data, jQuery can parse it using the $.parseJSON() method. This method takes a JSON string as an argument and returns a JavaScript object. You can then use this object to access the data in the JSON.
How can I make synchronous AJAX requests in jQuery?
By default, AJAX requests in jQuery are asynchronous, meaning that the script continues to run while the request is being processed. However, you can make synchronous AJAX requests by setting the async option to false in the $.ajax() method. Note that synchronous requests can block the browser and are generally not recommended.
How can I send a JSON object from jQuery to a PHP script?
To send a JSON object from jQuery to a PHP script, you can use the JSON.stringify() method to convert the object into a JSON string. You can then send this string as data in the AJAX request. The PHP script can decode the JSON string using the json_decode() function.
How can I use the POST method in jQuery AJAX?
To use the POST method in jQuery AJAX, you can use the $.post() method. This method is a shorthand for the $.ajax() method with the type option set to “POST”. The $.post() method takes three arguments: the URL of the PHP script, the data to send, and a function to handle the response.
How can I use the GET method in jQuery AJAX?
To use the GET method in jQuery AJAX, you can use the $.get() method. This method is a shorthand for the $.ajax() method with the type option set to “GET”. The $.get() method takes three arguments: the URL of the PHP script, the data to send, and a function to handle the response.
How can I set the content type of an AJAX request in jQuery?
To set the content type of an AJAX request in jQuery, you can use the contentType option in the $.ajax() method. This option sets the Content-Type HTTP header of the request. For instance, to send JSON data, you can set the contentType option to “application/json”.
How can I handle the success of an AJAX request in jQuery?
To handle the success of an AJAX request in jQuery, you can use the .done() method. This method is called when the request succeeds. It takes a function as an argument, which will be executed when the request is successful. Inside this function, you can write code to handle the response.
How can I cancel an AJAX request in jQuery?
To cancel an AJAX request in jQuery, you can use the .abort() method. This method cancels the request if it has not yet been sent, or if it has been sent but not yet completed. Note that not all requests can be cancelled, depending on the browser and the type of the request.
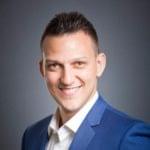
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.