Simple jQuery code snippet to get label text associated with checkbox.
console.log($('label[for="carHireChk"]').html());
$("label[for='comedyclubs']")
$("label[for=' + this.attr("id") + ']").attr('class', 'error');
See doco for more info: http://api.jquery.com/next-adjacent-Selector/
Frequently Asked Questions (FAQs) about jQuery Label Text Checkbox
How can I get the text of a selected checkbox using jQuery?
To get the text of a selected checkbox using jQuery, you can use the .text() method. This method returns the text content of the selected elements. Here’s an example:$('input[type="checkbox"]:checked').each(function() {
var checkboxText = $(this).next('label').text();
console.log(checkboxText);
});
In this code, we’re selecting all checked checkboxes and for each of them, we’re getting the text of the next label element.
How can I change the text of a label using jQuery?
To change the text of a label using jQuery, you can use the .text() method. This method sets the text content of the selected elements. Here’s an example:$('label[for="myCheckbox"]').text('New Label Text');
In this code, we’re selecting the label for a checkbox with the id “myCheckbox” and setting its text to “New Label Text”.
How can I select a checkbox by its label text using jQuery?
To select a checkbox by its label text using jQuery, you can use the :contains() selector. This selector selects elements that contain the specified text. Here’s an example:$('label:contains("Label Text")').prev('input[type="checkbox"]').prop('checked', true);
In this code, we’re selecting the label that contains “Label Text” and checking the previous checkbox.
How can I get the value of a selected checkbox using jQuery?
To get the value of a selected checkbox using jQuery, you can use the .val() method. This method returns the value of the selected elements. Here’s an example:$('input[type="checkbox"]:checked').each(function() {
var checkboxValue = $(this).val();
console.log(checkboxValue);
});
In this code, we’re selecting all checked checkboxes and for each of them, we’re getting its value.
How can I check or uncheck a checkbox using jQuery?
To check or uncheck a checkbox using jQuery, you can use the .prop() method. This method sets properties on the selected elements. Here’s an example:// To check
$('input[type="checkbox"]').prop('checked', true);
// To uncheck
$('input[type="checkbox"]').prop('checked', false);
In this code, we’re selecting all checkboxes and checking or unchecking them.
How can I toggle a checkbox using jQuery?
To toggle a checkbox using jQuery, you can use the .click() method. This method simulates a mouse click on the selected elements. Here’s an example:$('input[type="checkbox"]').click();
In this code, we’re selecting all checkboxes and toggling them.
How can I bind an event handler to the change event of a checkbox using jQuery?
To bind an event handler to the change event of a checkbox using jQuery, you can use the .change() method. This method attaches an event handler function to the change event. Here’s an example:$('input[type="checkbox"]').change(function() {
if ($(this).is(':checked')) {
console.log('Checkbox is checked');
} else {
console.log('Checkbox is unchecked');
}
});
In this code, we’re selecting all checkboxes and binding an event handler to their change event.
How can I select all checkboxes using jQuery?
To select all checkboxes using jQuery, you can use the :checkbox selector. This selector selects all checkbox inputs. Here’s an example:$('input:checkbox').prop('checked', true);
In this code, we’re selecting all checkboxes and checking them.
How can I deselect all checkboxes using jQuery?
To deselect all checkboxes using jQuery, you can use the :checkbox selector. This selector selects all checkbox inputs. Here’s an example:$('input:checkbox').prop('checked', false);
In this code, we’re selecting all checkboxes and unchecking them.
How can I get the number of checked checkboxes using jQuery?
To get the number of checked checkboxes using jQuery, you can use the .length property. This property returns the number of elements in the jQuery object. Here’s an example:var checkedCount = $('input[type="checkbox"]:checked').length;
console.log(checkedCount);
In this code, we’re selecting all checked checkboxes and getting their count.
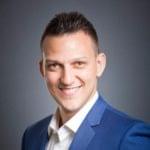
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.