This is a little jQuery function I wrote to add a selected class to an element based on the current date and time (using date timestamp). The idea being to set a current session which is currently showing, like shown in the screenshot below.
$.dateTimeHighlightNow() function
/**
* $.dateTimeHighlightNow()
* Author: Sam Deering
* Adds/removes a selected class on elements based on the current date and time.
* usage: $('.program p').dateTimeHighlightNow();
*/
jQuery.fn.dateTimeHighlightNow = function()
{
return this.each(function()
{
var datetimestamp = Math.round(new Date().getTime() / 1000)
elem = $(this),
start = elem.attr('start'),
finish= elem.attr('finish');
log('datetimestamp = '+datetimestamp);
if (start < datetimestamp && finish > datetimestamp)
{
elem.addClass('selected');
log(elem);
}
else
{
elem.removeClass('selected');
}
});
};
Usage:
$('.program p').dateTimeHighlight();
Your HTML code should look something like this:
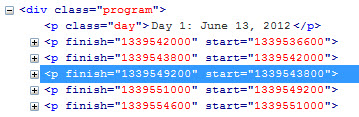
/* monitor for auto change of current active session based on date/time */
setInterval(function()
{
//run every minute
$('.program p').dateTimeHighlight();
}, 60000);
Even Further thoughts
- PHP timestamp is executed on the server side (your servers system clock).
- JavaScript timestamp is executed on the client-side (your pc system clock).
- A futher check to convert the clients Time Zone settings to the servers to highlight current session (this is something I may look into implementing in the near future so stay tuned for that).
Frequently Asked Questions (FAQs) about jQuery Highlight Effect
How can I use the jQuery highlight effect to emphasize a specific element on my webpage?
To use the jQuery highlight effect, you first need to include the jQuery library in your HTML file. After that, you can use the .effect() method with the “highlight” effect. This method takes three parameters: the name of the effect, the options object, and the duration of the effect. For example, to highlight a div with the id “myDiv”, you would use the following code:$("#myDiv").effect("highlight", {}, 3000);
This will highlight the div for 3 seconds.
Can I change the color of the highlight effect in jQuery?
Yes, you can change the color of the highlight effect by using the color option in the options object. For example, to change the highlight color to red, you would use the following code:$("#myDiv").effect("highlight", {color: "#ff0000"}, 3000);
How can I stop the highlight effect before it finishes?
You can stop the highlight effect before it finishes by using the .stop() method. This method takes two parameters: the clearQueue parameter and the jumpToEnd parameter. If clearQueue is set to true, all animations in the queue will be removed. If jumpToEnd is set to true, the current animation will be completed immediately. For example, to stop the highlight effect on a div with the id “myDiv”, you would use the following code:$("#myDiv").stop(true, true);
Can I use the highlight effect on multiple elements at once?
Yes, you can use the highlight effect on multiple elements at once by using a class selector or the .each() method. For example, to highlight all divs with the class “highlight”, you would use the following code:$(".highlight").effect("highlight", {}, 3000);
How can I make the highlight effect repeat?
You can make the highlight effect repeat by using a setInterval function. This function takes two parameters: the function to be executed and the time interval in milliseconds. For example, to make the highlight effect on a div with the id “myDiv” repeat every 5 seconds, you would use the following code:setInterval(function() {
$("#myDiv").effect("highlight", {}, 3000);
}, 5000);
Can I use the highlight effect with other effects?
Yes, you can use the highlight effect with other effects by chaining them together. For example, to use the highlight effect and the bounce effect on a div with the id “myDiv”, you would use the following code:$("#myDiv").effect("highlight", {}, 3000).effect("bounce", {}, 3000);
How can I use the highlight effect on hover?
You can use the highlight effect on hover by using the .hover() method. This method takes two functions as parameters: one for when the mouse enters the element and one for when the mouse leaves the element. For example, to use the highlight effect on a div with the id “myDiv” when the mouse hovers over it, you would use the following code:$("#myDiv").hover(function() {
$(this).effect("highlight", {}, 3000);
});
Can I use the highlight effect on click?
Yes, you can use the highlight effect on click by using the .click() method. This method takes a function as a parameter that will be executed when the element is clicked. For example, to use the highlight effect on a div with the id “myDiv” when it is clicked, you would use the following code:$("#myDiv").click(function() {
$(this).effect("highlight", {}, 3000);
});
How can I use the highlight effect on form input?
You can use the highlight effect on form input by using the .focus() and .blur() methods. The .focus() method is triggered when the input field gets focus, and the .blur() method is triggered when the input field loses focus. For example, to use the highlight effect on an input field with the id “myInput”, you would use the following code:$("#myInput").focus(function() {
$(this).effect("highlight", {}, 3000);
}).blur(function() {
$(this).effect("highlight", {color: "#ff0000"}, 3000);
});
Can I use the highlight effect on page load?
Yes, you can use the highlight effect on page load by using the .ready() method. This method is triggered when the DOM is fully loaded. For example, to use the highlight effect on a div with the id “myDiv” when the page loads, you would use the following code:$(document).ready(function() {
$("#myDiv").effect("highlight", {}, 3000);
});
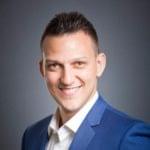
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.