If your working with JavaScript regular expressions a lot (like me) this is worth knowing. For those of you that don’t know the exec() method simply tests for a match in a string and returns the matched text if it finds a match, otherwise it returns null.
/* match just the href of js includes */
var jsSrcRegex = /src="(.+?)"/igm;
/* html for js include */
var html = '<script type="text/javascript" src="/jquery-1.6.1.min.js"></script>';
console.log(jsSrcRegex.exec(html));
console.log(html);
console.log(jsSrcRegex);
console.log(jsSrcRegex.exec(html));

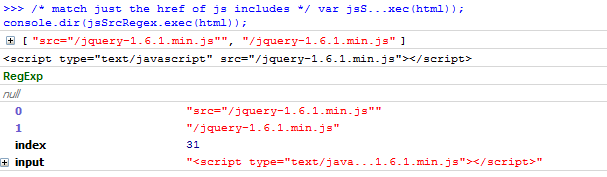
regex.compile() fixes the problem
Now, if you use .compile() on the regex it works!/* match just the href of js includes */
var jsSrcRegex = /src="(.+?)"/igm;
/* html for js include */
var html = '<script type="text/javascript" src="/jquery-1.6.1.min.js"></script>';
console.log(jsSrcRegex.exec(html));
/* recompile the regex */
jsSrcRegex.compile(jsSrcRegex);
console.log(html);
console.log(jsSrcRegex);
console.log(jsSrcRegex.exec(html));
As you can see the array of results is found once we recompiled.

Frequently Asked Questions about jQuery and Regex
What is the purpose of using jQuery exec() and compile() functions with Regex?
The exec() and compile() functions in jQuery are used in conjunction with regular expressions (Regex). The exec() function is a Regex method that returns an array of information or null on a mismatch. It’s used to find matches in a string. On the other hand, the compile() function is used to change the Regex pattern. This can be useful when you need to change the pattern dynamically based on certain conditions in your code.
How can I use the exec() function in jQuery?
The exec() function is a method of the RegExp object. It searches a string for a specified pattern and returns the found text as an object. If no match is found, it returns null. Here’s a basic example:var myRegex = /hello/;
var myString = "hello world";
var result = myRegex.exec(myString);
console.log(result); // Outputs: ["hello"]
How can I use the compile() function in jQuery?
The compile() function is a method of the RegExp object. It’s used to change and recompile the regular expression. However, it’s important to note that this method is deprecated and not recommended for use in new projects. Instead, you can simply create a new RegExp object with the new pattern.
How can I use jQuery to match a Regex pattern in a string?
You can use the match() function in jQuery to search a string for a match against a regular expression, and return the matches, as an Array object. Here’s an example:var myString = "Hello World!";
var result = myString.match(/World/);
console.log(result); // Outputs: ["World"]
What are some common use cases for Regex in jQuery?
Regular expressions in jQuery are commonly used for form validation, search and replace operations, data extraction from strings, and more. They provide a powerful way to manipulate text and data in your web applications.
How can I test my jQuery and Regex code online?
There are several online platforms where you can test your jQuery and Regex code, such as JSFiddle, CodePen, and the online jQuery editor on Tutorialspoint. These platforms provide a live coding environment where you can write, test, and debug your code.
What are some common mistakes to avoid when using Regex in jQuery?
Some common mistakes include not properly escaping special characters, using the wrong flags, and not considering all possible input scenarios. It’s also important to test your regular expressions thoroughly to ensure they behave as expected.
How can I learn more about regular expressions in JavaScript and jQuery?
There are many resources available online to learn about regular expressions in JavaScript and jQuery. Websites like W3Schools, MDN Web Docs, and Stack Overflow have comprehensive guides and tutorials. You can also find many video tutorials on platforms like YouTube.
Can I use Regex with other jQuery methods besides exec() and compile()?
Yes, regular expressions can be used with several jQuery methods, such as test(), match(), replace(), and search(). Each of these methods provides different functionality for manipulating and searching strings.
How can I improve the performance of my Regex in jQuery?
There are several ways to improve the performance of your regular expressions in jQuery. These include avoiding greedy quantifiers, using non-capturing groups when you don’t need the matched data, and using character classes instead of alternation where possible.
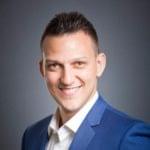
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.