jQuery code snippet to create an event action when a user has scrolled down to a percentage of the web page. We have tested that it’s best to use values between 55 and 100% when catching mouse scrolls.
$(document).ready(function(){
//Example - show a div after 75% of the web page is scrolled.
var webpage = $("body");
var webpage_height = webpage.height();
//alert(webpage_height);
var trigger_height = webpage_height * 0.75;
if ($(window).scrollTop() > (webpage_height-trigger_height)) {
//alert($(window).scrollTop()+" > "+(webpage_height-trigger_height));
$("#divtoshow").show();
}
});
Frequently Asked Questions (FAQs) about jQuery Scroll Events
How can I detect a scroll event in jQuery?
Detecting a scroll event in jQuery is quite straightforward. You can use the .scroll() method, which is a built-in function in jQuery. This method triggers the scroll event, or attaches a function to run when a scroll event occurs. Here is a simple example:$(window).scroll(function(){
console.log("Scroll event detected!");
});
In this code, whenever a scroll event occurs in the window, the message “Scroll event detected!” will be logged to the console.
How can I determine the direction of a jQuery scroll event?
Determining the direction of a scroll event in jQuery requires a bit more work. You need to compare the current scroll position with the previous one. Here is a basic example:var lastScrollTop = 0;
$(window).scroll(function(){
var currentScrollTop = $(this).scrollTop();
if (currentScrollTop > lastScrollTop){
console.log("Scrolled down");
} else if (currentScrollTop < lastScrollTop){
console.log("Scrolled up");
}
lastScrollTop = currentScrollTop;
});
In this code, we store the last scroll position in a variable. Then, on each scroll event, we compare the current scroll position with the last one to determine the direction of the scroll.
What does the .scrollTop() method do in jQuery?
The .scrollTop() method in jQuery gets the current vertical position of the scroll bar for the first element in the set of matched elements or sets the vertical position of the scroll bar for every matched element. When called with no arguments, it returns the scroll position. When called with an argument, it sets the scroll position.
Can I trigger a function when the page is scrolled to a certain point?
Yes, you can trigger a function when the page is scrolled to a certain point. You can do this by checking the current scroll position in the scroll event handler. Here is an example:$(window).scroll(function(){
if ($(this).scrollTop() > 200){
console.log("Scrolled more than 200px");
}
});
In this code, the message “Scrolled more than 200px” will be logged to the console whenever the scroll position exceeds 200 pixels.
How can I animate the scroll event in jQuery?
You can animate the scroll event in jQuery using the .animate() method. This method allows you to create custom animation effects on numbers. Here is an example:$('html, body').animate({
scrollTop: $("#myDiv").offset().top
}, 2000);
In this code, the page will smoothly scroll to the top position of the element with the ID “myDiv” over a period of 2 seconds.
Can I stop or prevent a scroll event in jQuery?
No, you cannot stop or prevent a scroll event in jQuery. The scroll event is a native browser event that cannot be cancelled. However, you can prevent the default action of certain events that may cause a scroll, such as the mousewheel event.
How can I detect a scroll event on a specific element in jQuery?
You can detect a scroll event on a specific element in jQuery by attaching the .scroll() method to that element. Here is an example:$("#myDiv").scroll(function(){
console.log("Scroll event detected on #myDiv!");
});
In this code, a scroll event on the element with the ID “myDiv” will log the message “Scroll event detected on #myDiv!” to the console.
Can I detect horizontal scroll events in jQuery?
Yes, you can detect horizontal scroll events in jQuery using the .scrollLeft() method. This method works similarly to the .scrollTop() method, but for horizontal scrolling.
How can I detect the end of a scroll event in jQuery?
Detecting the end of a scroll event in jQuery can be done by comparing the current scroll position with the total scrollable height. Here is an example:$(window).scroll(function(){
if ($(this).scrollTop() + $(this).innerHeight() >= this.scrollHeight){
console.log("End of scroll detected!");
}
});
In this code, the message “End of scroll detected!” will be logged to the console when the end of the scroll is reached.
Can I detect a scroll event on a mobile device in jQuery?
Yes, you can detect a scroll event on a mobile device in jQuery. The .scroll() method works on mobile devices just like it does on desktop browsers. However, keep in mind that mobile browsers may handle scroll events differently than desktop browsers, so it’s always a good idea to test your code on multiple devices.
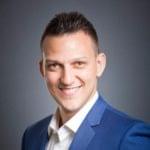
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.