function isValidDate(s) {
var bits = s.split('/');
var d = new Date(bits[2] + '/' + bits[1] + '/' + bits[0]);
return !!(d && (d.getMonth() + 1) == bits[1] && d.getDate() == Number(bits[0]));
}
//Test it!
var currentDate = new Date('31/09/2011');
console.log(isValidDate(currentDate.toString()));
console.log(isValidDate('30/09/2011'));
JavaScript Function to to get the number of days in a month.
function daysInMonth(month, year) {
return new Date(year, month, 0).getDate();
}
//Test it!
daysInMonth('09','2011');
//Output: 30
Also See:
- jQuery Get Later Date / Future Date
- JavaScript Showing Wrong Year For Date
- jQuery DATETIME Functions – Complete Listing
/* check a date is valid, if not revert to last day in month */
validateDateLastDayMonth: function()
{
/* helpers */
function isValidDate(s) {
var bits = s.split('/');
var d = new Date(bits[2] + '/' + bits[1] + '/' + bits[0]);
return !!(d && (d.getMonth() + 1) == bits[1] && d.getDate() == Number(bits[0]));
}
function daysInMonth(month, year) {
return new Date(year, month, 0).getDate();
}
/* init */
var currentDate = new Date(),
currentMonth = currentDate.getMonth() + 1,
lastDayOfMonth = new Date(currentDate.getFullYear(), (currentDate.getMonth() - 1), 0).getDate(),
departureDate = FCL.DATETIME.futureDateDays(14),
depDate = departureDate.split('/'),
departureDateMonth = depDate[1];
if (departureDateMonth != currentMonth) {
departureDate = FCL.DATETIME.leadingZero(lastDayOfMonth) +'/'+ FCL.DATETIME.leadingZero(currentMonth) +'/'+ depDate[2];
}
/* validate date */
if (!isValidDate(departureDate.toString()))
{
var bits = departureDate.split('/');
departureDate = FCL.DATETIME.leadingZero(daysInMonth(bits[1],bits[2])) +'/'+ FCL.DATETIME.leadingZero(currentMonth) +'/'+ depDate[2];
}
$('input[name="depDate"]').val(departureDate);
}
Frequently Asked Questions (FAQs) about jQuery Date Validation
How Can I Validate a Date in a Specific Format Using jQuery?
jQuery provides a method called $.datepicker.parseDate
which can be used to validate a date in a specific format. This method takes two parameters: the format of the date and the date string. If the date string matches the format, the method returns a Date object. If it doesn’t match, it throws an error. Here’s an example:try {
$.datepicker.parseDate('dd/mm/yy', '31/12/2020');
console.log('Date is valid');
} catch (error) {
console.log('Date is invalid');
}
What is the jQuery Validation Plugin and How Can It Be Used for Date Validation?
The jQuery Validation Plugin provides a powerful framework for client-side form validation. It includes built-in validation methods for common needs, including date validation. To use it, you need to include the plugin in your project and call the validate
method on your form. You can specify rules for each form field, including the date field. For example:$('form').validate({
rules: {
dateField: {
date: true
}
}
});
How Can I Validate a Date Range Using jQuery?
To validate a date range, you can use the $.datepicker.parseDate
method to convert the date strings to Date objects, and then compare them. Here’s an example:var startDate = $.datepicker.parseDate('dd/mm/yy', '01/01/2020');
var endDate = $.datepicker.parseDate('dd/mm/yy', '31/12/2020');
if (startDate > endDate) {
console.log('Start date is after end date');
} else {
console.log('Date range is valid');
}
Can I Use jQuery to Validate a Date Without Using a Plugin?
Yes, you can validate a date without using a plugin by using the built-in JavaScript Date object. However, this method is less flexible and more complex than using a plugin or the $.datepicker.parseDate
method. Here’s an example:var dateString = '31/12/2020';
var dateParts = dateString.split('/');
var dateObject = new Date(+dateParts[2], dateParts[1] - 1, +dateParts[0]);
if (dateObject.getDate() == dateParts[0] && dateObject.getMonth() == dateParts[1] - 1 && dateObject.getFullYear() == dateParts[2]) {
console.log('Date is valid');
} else {
console.log('Date is invalid');
}
How Can I Customize the Error Messages in jQuery Validation Plugin?
You can customize the error messages in the jQuery Validation Plugin by specifying the messages
option in the validate
method. Here’s an example:$('form').validate({
rules: {
dateField: {
date: true
}
},
messages: {
dateField: {
date: 'Please enter a valid date'
}
}
});
How Can I Validate a Date in a Different Language Using jQuery?
The jQuery UI Datepicker provides localization support for different languages. You can include the localization file for your language and then use the $.datepicker.regional
option to set the date format and other options for your language. Here’s an example:$.datepicker.setDefaults($.datepicker.regional['fr']);
How Can I Validate a Date in a Leap Year Using jQuery?
The $.datepicker.parseDate
method and the jQuery Validation Plugin automatically handle leap years. If you’re using the JavaScript Date object, you can check for a leap year by using this condition:if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
// It's a leap year
}
How Can I Validate a Date in the Future Using jQuery?
To validate a date in the future, you can compare the date with the current date. Here’s an example:var date = $.datepicker.parseDate('dd/mm/yy', '31/12/2020');
var now = new Date();
if (date > now) {
console.log('Date is in the future');
} else {
console.log('Date is not in the future');
}
How Can I Validate a Date in the Past Using jQuery?
To validate a date in the past, you can compare the date with the current date, similar to validating a date in the future. Here’s an example:var date = $.datepicker.parseDate('dd/mm/yy', '31/12/2020');
var now = new Date();
if (date < now) {
console.log('Date is in the past');
} else {
console.log('Date is not in the past');
}
How Can I Validate a Date in a Specific Month or Year Using jQuery?
To validate a date in a specific month or year, you can use the getMonth
and getFullYear
methods of the Date object. Here’s an example:var date = $.datepicker.parseDate('dd/mm/yy', '31/12/2020');
if (date.getMonth() == 11 && date.getFullYear() == 2020) {
console.log('Date is in December 2020');
} else {
console.log('Date is not in December 2020');
}
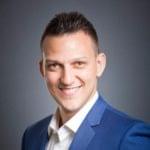
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.