jQuery code snippet to update images with a domain name, turning it from a relative path into an absolute path. Sometimes useful when testing across domains with the same static content or pulling images from an external domain.
(function ($) {
$(document).ready(function () {
$('img').each(function (i, v) {
var $el = $(this),
s = $el.attr('src'),
sRx = /[/res/images/.*]+/igm;
console.log(s);
console.log(s.test(sRx));
if (s.test(sRx)) {
console.log('match');
s = 'http://splash.abc.net.au' + s;
$el.attr('src', s);
}
});
});
})(jQuery);
jsfiddle.net/BK9Zp/
JSfiddly lolz.
There is an awesome project by Ben Alman which has all the bells and whistles in this area check it out:
https://raw.github.com/cowboy/jquery-urlinternal/master/jquery.ba-urlinternal.js
Frequently Asked Questions (FAQs) about Relative and Absolute Paths
What is the difference between relative and absolute paths?
Relative and absolute paths are two different ways to specify the location of a file or directory in a computer’s file system. An absolute path specifies the exact location of a file or directory from the root directory, while a relative path specifies the location of a file or directory relative to the current directory. For example, if you are in the directory /home/user/documents, the relative path to a file named file.txt in the same directory would be simply file.txt, while the absolute path would be /home/user/documents/file.txt.
How do I get the value of the src attribute in jQuery?
In jQuery, you can get the value of the src attribute of an image by using the attr() method. Here’s an example:$('img').attr('src');
This will return the src attribute of the first image on the page. If you want to get the src attribute of all images on a page, you can use the each() method like this:$('img').each(function() {
console.log($(this).attr('src'));
});
This will log the src attribute of each image to the console.
What is the difference between a relative path and an absolute path in HTML?
In HTML, a relative path refers to a location that is relative to the current page without needing to specify the full URL. For example, if you have an image in the same directory as your HTML file, you can use a relative path to link to it like this: <img src="image.jpg">
. An absolute path, on the other hand, refers to the exact location of a file or directory, and it always includes the full URL. For example: <img src="http://www.example.com/image.jpg">
.
How do I solve the src absolute path problem?
If you’re having trouble with the src attribute not finding the file you’re trying to link to, it’s likely that the path you’re providing is incorrect. Make sure that the path is correct and that the file exists at that location. If you’re using an absolute path, make sure that the URL is correct and accessible. If you’re using a relative path, make sure that the path is correct relative to the current file.
How do I get the src attribute of all images on a page with jQuery?
You can get the src attribute of all images on a page with jQuery by using the each() method. Here’s an example:$('img').each(function() {
console.log($(this).attr('src'));
});
This will log the src attribute of each image to the console. If you want to store these in an array, you can do so like this:var srcs = [];
$('img').each(function() {
srcs.push($(this).attr('src'));
});
Now the srcs array contains the src attribute of all images on the page.
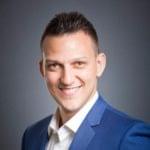
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.