A really cool feature of jQuery is the ability to change an image dynamically, like when you move the mouse over a certain area of the screen a picture will change.
How you do it: You reference the image src property and change it via 2 functions. The first function changes the image and the second changes it back. Then in the HTML we add an event to the area which the mouse will trigger the functions (note these triggers could also be added to the JavaScript). Simple as that. See Live Demo
jQuery change image dynamically
//this code sits outside the (document).ready function
function twittereyesopen() {
//alert("open");
var name_element = $('#twitter-image');
name_element.src = "/images/page-images/twitter-eyes-open.jpg";
}
function twittereyesclosed() {
//alert("closed");
var name_element = $('#twitter-image');
name_element.src = "/images/page-images/twitter-eyes-closed.jpg";
}
The following goes into the HTML:
Frequently Asked Questions about jQuery Image Hover Effects
How can I use jQuery to change an image on hover?
To change an image on hover using jQuery, you need to use the hover() method. This method specifies two functions to run when the mouse pointer hovers over the selected elements. The first function is executed when the mouse enters the HTML element, and the second function is executed as the mouse leaves the HTML element. Here is a simple example:$(document).ready(function(){
$("img").hover(function(){
$(this).attr("src", "image2.jpg");
}, function(){
$(this).attr("src", "image1.jpg");
});
});
In this example, when you hover over an image, it changes to ‘image2.jpg’. When you move the mouse away, it changes back to ‘image1.jpg’.
Can I use CSS instead of jQuery to change an image on hover?
Yes, you can use CSS to change an image on hover. This can be done using the :hover pseudo-class. Here is an example:img:hover {
content: url('image2.jpg');
}
In this example, when you hover over an image, it changes to ‘image2.jpg’. However, this method does not provide a way to change the image back when the mouse leaves the image. For that functionality, you would need to use JavaScript or jQuery.
How can I change the image on hover for a different div using jQuery?
To change the image on hover for a different div, you can use the hover() method in combination with the find() method. The find() method returns descendant elements of the selected element. Here is an example:$(document).ready(function(){
$("#div1").hover(function(){
$("#div2").find("img").attr("src", "image2.jpg");
}, function(){
$("#div2").find("img").attr("src", "image1.jpg");
});
});
In this example, when you hover over ‘div1’, the image in ‘div2’ changes to ‘image2.jpg’. When you move the mouse away from ‘div1’, the image in ‘div2’ changes back to ‘image1.jpg’.
How can I change the image on hover for a list item using jQuery?
To change the image on hover for a list item, you can use the hover() method in combination with the children() method. The children() method returns all direct children of the selected element. Here is an example:$(document).ready(function(){
$("li").hover(function(){
$(this).children("img").attr("src", "image2.jpg");
}, function(){
$(this).children("img").attr("src", "image1.jpg");
});
});
In this example, when you hover over a list item, the child image of that list item changes to ‘image2.jpg’. When you move the mouse away from the list item, the child image changes back to ‘image1.jpg’.
How can I add a transition effect when the image changes on hover using jQuery?
To add a transition effect when the image changes on hover, you can use the fadeIn() and fadeOut() methods. These methods gradually change the opacity for selected elements from hidden to visible (fadeIn) and from visible to hidden (fadeOut). Here is an example:$(document).ready(function(){
$("img").hover(function(){
$(this).fadeOut(500, function(){
$(this).attr("src", "image2.jpg").fadeIn(500);
});
}, function(){
$(this).fadeOut(500, function(){
$(this).attr("src", "image1.jpg").fadeIn(500);
});
});
});
In this example, when you hover over an image, it fades out, changes to ‘image2.jpg’, and then fades in. When you move the mouse away, it fades out, changes back to ‘image1.jpg’, and then fades in. The number 500 represents the duration of the effect in milliseconds.
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
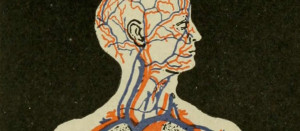
Published in
·Design·Design & UX·Statistics and Analysis·UI Design·Usability·UX·Web·September 1, 2016