Use jQuery capture the event of when the browser window is resized, then do something. In the example below it logs the window’s new size. Update 16/05/2013: See the debounce method below for smarter window resizing!
//capture window resize
$(window).bind('resize', function(e)
{
var win = $(this),
w = win.width(),
h = win.height();
console.log('window resized to: ' + w + ' by ' + h);
});
//output: window resized to: 1598 by 521
Refresh page on browser resize
A pretty hacky cross browser IE8+ solution.//this is in a timeout so it works in IE8
setTimeout(function()
{
$(window).bind('resize', function(e)
{
if(window.RT) clearTimeout(window.RT);
window.RT = setTimeout(function()
{
this.location.reload(false); /* false to get page from cache */
}, 300);
});
}, 1000);
Example to reposition a nav bar when window is resized
Move navigation menu bar when the window is resized. Slight 300ms delay but this is to stop it from recursively calling reposition when browser is being resized.
(function($,W)
{
//DOM Ready
$(function()
{
//responsive main nav absolute top position relative to window height
function repositionMainNav()
{
var newT = W.innerHeight - 300;
newT = (newT < 165) ? 165 : newT; //min top
newT = (newT > 550) ? 550 : newT; //max top
// console.log(newT);
$('#navbar').css('top', newT);
}
repositionMainNav();
$(W).bind('resize', function(e)
{
if(W.RT) clearTimeout(W.RT);
W.RT = setTimeout(function()
{
//recalculate the vertical position of the main nav
repositionMainNav();
}, 300);
});
});
})(jQuery, window);
Decounced “Smarter” window resize event
Courtesy of the ever outstanding Mr Paul Irish in his debounced post and see the demo in action.(function($,sr){
// debouncing function from John Hann
// http://unscriptable.com/index.php/2009/03/20/debouncing-javascript-methods/
var debounce = function (func, threshold, execAsap) {
var timeout;
return function debounced () {
var obj = this, args = arguments;
function delayed () {
if (!execAsap)
func.apply(obj, args);
timeout = null;
};
if (timeout)
clearTimeout(timeout);
else if (execAsap)
func.apply(obj, args);
timeout = setTimeout(delayed, threshold || 100);
};
}
// smartresize
jQuery.fn[sr] = function(fn){ return fn ? this.bind('resize', debounce(fn)) : this.trigger(sr); };
})(jQuery,'smartresize');
// usage:
$(window).smartresize(function(){
// code that takes it easy...
});
Frequently Asked Questions about jQuery Window Resize
How can I use jQuery to capture window resize events?
To capture window resize events using jQuery, you can use the .resize() method. This method attaches a function to run when a resize event is fired. Here’s a simple example:$(window).resize(function() {
console.log('Window has been resized!');
});
In this code, whenever the window is resized, the message ‘Window has been resized!’ will be logged to the console.
What is the difference between .resize() and .on(‘resize’) in jQuery?
Both .resize() and .on(‘resize’) are used to attach resize event handlers in jQuery. The .resize() method is a shorthand for .on(‘resize’). The main difference is that .on() method provides a way to attach event handlers to elements that may not yet exist. It’s generally recommended to use .on() for its flexibility.
How can I trigger the resize event manually in jQuery?
You can manually trigger the resize event using the .trigger() method in jQuery. Here’s how you can do it:$(window).trigger('resize');
This code will trigger the resize event on the window.
How can I remove a resize event handler in jQuery?
You can remove a resize event handler using the .off() method in jQuery. Here’s an example:$(window).off('resize');
This code will remove all resize event handlers from the window.
How can I get the new window size after a resize event in jQuery?
You can get the new window size using the .width() and .height() methods in jQuery. Here’s how you can do it:$(window).resize(function() {
var newWidth = $(window).width();
var newHeight = $(window).height();
console.log('New width: ' + newWidth + ', New height: ' + newHeight);
});
This code will log the new window size to the console whenever the window is resized.
Can I use jQuery to capture resize events on elements other than the window?
Yes, you can use jQuery to capture resize events on any element, not just the window. However, keep in mind that the resize event is only fired on the window by default in most browsers. To capture resize events on other elements, you may need to use a plugin or custom code.
How can I debounce the resize event in jQuery to improve performance?
Debouncing the resize event can help improve performance by limiting the number of times the event handler is called during continuous resizing. Here’s how you can do it using the _.debounce function from the Underscore.js library:$(window).resize(_.debounce(function() {
console.log('Window has been resized!');
}, 250));
This code will delay the execution of the event handler until 250 milliseconds have passed without another resize event.
How can I use jQuery to capture resize events in a cross-browser compatible way?
The .resize() method in jQuery is cross-browser compatible and should work in all modern browsers. However, keep in mind that the resize event is only fired on the window by default in most browsers. To capture resize events on other elements in a cross-browser compatible way, you may need to use a plugin or custom code.
Can I use jQuery to capture resize events on mobile devices?
Yes, you can use jQuery to capture resize events on mobile devices. However, keep in mind that the behavior of the resize event can vary between different mobile browsers. For example, some mobile browsers may fire the resize event when the address bar hides or shows.
How can I use jQuery to capture both resize and orientation change events?
You can use jQuery to capture both resize and orientation change events by attaching event handlers to both events. Here’s how you can do it:$(window).on('resize orientationchange', function() {
console.log('Window has been resized or orientation has been changed!');
});
This code will log a message to the console whenever the window is resized or the orientation is changed.
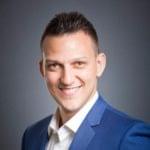
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.