Using ajaxStart and ajaxStop methods
The example below we have used it to display a loading image and disable a form submit button once it has been clicked while the ajax request is being processed. And then when it returns the image is hidden and the submit button becomes available again.var $form = $('#form');
$form.find('.loading')
.hide()
.ajaxStart(function()
{
$(this).show();
$form.find('.submit').attr('disabled', 'disabled');
})
.ajaxStop(function()
{
$(this).hide();
$form.find('.submit').removeAttr('disabled');
});
Using inserted code before ajax
Another method might be to add the image before the ajax call.//show loading image, disable submit button
$form.find('.msg').remove();
$form.find('.loading').show();
$form.find('.submit').attr('disabled', 'disabled');
And then add a complete handler to the ajax function.
//hide loading image, enable submit button again
complete: function()
{
$form.find('.loading').hide();
$form.find('.submit').removeAttr('disabled');
}
Using ajaxSetup()
A further method is to use jQuery.ajaxSetup so that the image is hidden and form enabled when all ajax requests are returned “completed”.$.ajaxSetup( {
complete:function() {
$form.find('.loading').hide();
$form.find('.submit').removeAttr('disabled');
}
});
Frequently Asked Questions about jQuery AJAX Loading
What is the purpose of jQuery’s ajaxStart() method?
The ajaxStart() method in jQuery is a built-in function that specifies a function to be executed whenever an AJAX request begins and there are no other AJAX requests currently running. This method is often used to trigger a loading animation or message to inform the user that a process is running in the background, enhancing the user experience.
How can I use ajaxStart() to show a loading spinner?
To use ajaxStart() to show a loading spinner, you first need to create a spinner element in your HTML. Then, in your jQuery script, you can use the ajaxStart() method to display the spinner when an AJAX request starts. Here’s a simple example:$(document).ajaxStart(function(){
$("#spinner").show();
});
In this example, “#spinner” is the id of your spinner element. The spinner will be displayed whenever an AJAX request starts.
How can I hide the loading spinner when the AJAX request is complete?
You can use the ajaxStop() method to hide the spinner when the AJAX request is complete. Here’s how you can do it:$(document).ajaxStop(function(){
$("#spinner").hide();
});
In this example, “#spinner” is the id of your spinner element. The spinner will be hidden when the AJAX request is complete.
Can I use ajaxStart() with specific AJAX requests only?
Yes, you can use the ajaxStart() method with specific AJAX requests only. To do this, you need to bind the ajaxStart() method to the element that triggers the AJAX request instead of the document. Here’s an example:$("#myButton").ajaxStart(function(){
$("#spinner").show();
});
In this example, the spinner will be displayed only when an AJAX request triggered by the “#myButton” element starts.
What is the difference between ajaxStart() and ajaxSend()?
Both ajaxStart() and ajaxSend() are jQuery methods that are triggered at the start of an AJAX request. However, ajaxStart() is only triggered if there are no other AJAX requests currently running, while ajaxSend() is triggered for every AJAX request.
Can I use ajaxStart() with AJAX requests that use the GET method?
Yes, the ajaxStart() method can be used with AJAX requests that use any HTTP method, including GET, POST, PUT, DELETE, etc.
How can I handle errors in AJAX requests with ajaxStart()?
The ajaxStart() method itself does not provide a way to handle errors in AJAX requests. However, you can use the ajaxError() method in combination with ajaxStart() to handle errors. Here’s an example:$(document).ajaxStart(function(){
$("#spinner").show();
}).ajaxError(function(event, jqxhr, settings, thrownError){
$("#spinner").hide();
alert("An error occurred: " + thrownError);
});
In this example, the spinner is hidden and an alert is displayed whenever an error occurs in an AJAX request.
Can I use ajaxStart() in combination with other jQuery AJAX methods?
Yes, the ajaxStart() method can be used in combination with any other jQuery AJAX methods, such as ajaxStop(), ajaxComplete(), ajaxError(), ajaxSuccess(), etc.
How can I use ajaxStart() to improve the user experience on my website?
The ajaxStart() method can be used to improve the user experience on your website by providing feedback to the user when an AJAX request is running. For example, you can use ajaxStart() to display a loading spinner or message, which informs the user that a process is running in the background and that they need to wait.
Can I use ajaxStart() with jQuery’s shorthand AJAX methods?
Yes, the ajaxStart() method can be used with jQuery’s shorthand AJAX methods, such as $.get(), $.post(), $.load(), etc. The ajaxStart() method will be triggered whenever an AJAX request starts, regardless of the method used to initiate the request.
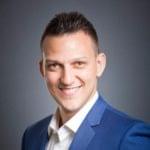
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.