Useful JavaScript Math Functions and How to Use Them
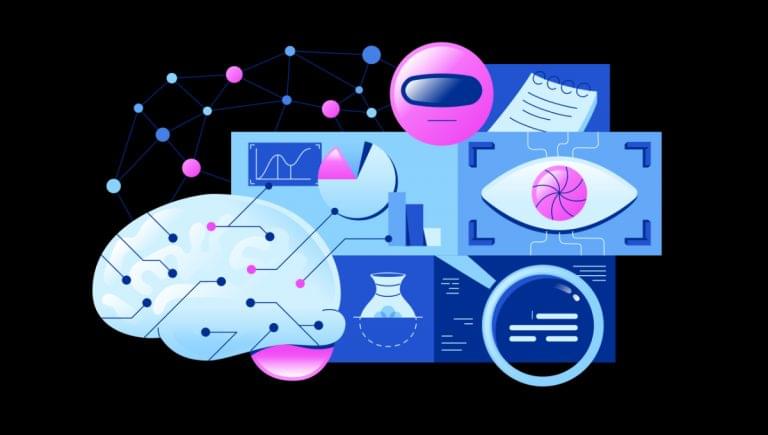
The built-in JavaScript Math object includes a number of useful functions for performing a variety of mathematical operations. Let’s dive in and take a look at how they work and what you might use them for.
Key Takeaways
Math.max and Math.min
These functions pretty much do what you’d expect: they return the maximum or minimum of the list of arguments supplied:
Math.max(1,2,3,4,5)
<< 5
Math.min(4,71,-7,2,1,0)
<< -7
The arguments all have to be of the Number
data type. Otherwise, NaN
will be returned:
Math.max('a','b','c')
<< NaN
Math.min(5,"hello",6)
<< NaN
Watch out, though. JavaScript will attempt to coerce values into a number:
Math.min(5,true,6)
<< 1
In this example, the Boolean value true
is coerced into the number 1
, which is why this is returned as the minimum value. If you’re not familiar with type coercion, it happens when the operands of an operator are of different types. In this case, JavaScript will attempt to convert one operand to an equivalent value of the other operand’s type. You can read more about type coercion in JavaScript: Novice to Ninja, 2nd Edition, in Chapter 2.
A list of numbers needs to be supplied as the argument, not an array, but you can use the spread operator (...
) to unpack an array of numbers:
Math.max(...[8,4,2,1])
<< 8
The Math.max
function is useful for finding the high score from a list of scores saved in an array:
const scores = [23,12,52,6,25,38,19,37,76,54,24]
const highScore = Math.max(...scores)
<< 76
The Math.min
function is useful for finding the best price on a price-comparison website:
const prices = [19.99, 20.25, 18.57, 19,75, 25, 22.50]
const bestPrice = Math.min(...prices)
<< 18.57
Absolute Values
An absolute value is simply the size of the number, no matter what its size. This means that positive numbers stay the same and negative numbers lose their minus sign. The Math.abs
function will calculate the absolute value of its argument:
Math.abs(5)
<< 5
Math.abs(-42)
<< 42
Math.abs(-3.14159)
<< 3.14159
Why would you want to do this? Well, sometimes you want to calculate the difference between two values, which you work out by subtracting the smallest from the largest, but often you won’t know which is the smallest of the two values in advance. To get around, this you can just subtract the numbers in any order and take the absolute value:
const x = 5
const y = 8
const difference = Math.abs(x - y)
<< 3
A practical example might be on a money-saving website, where you want to know how much you could save by calculating the difference between two deals, since you’d be dealing with live price data and wouldn’t know in advance which deal was the cheapest:
const dealA = 150
const dealB = 167
const saving = Math.abs(dealA - dealB)
<< 17
Math.pow
Math.pow
performs power calculations, like these:
3⁴ = 81
In the example above, 3 is known as the base number and 4 is the exponent. We would read it as “3 to the power of 4 is 81”.
The function accepts two values — the base and the exponent — and returns the result of raising the base to the power of the exponent:
Math.pow(2,3)
<< 8
Math.pow(8,0)
<< 1
Math.pow(-1,-1)
<< -1
Math.pow
has pretty much been replaced by the infix exponentiation operator (**
) — introduced in ES2016 — which does exactly the same operation:
2 ** 3
<< 8
8 ** 0
<< 1
(-1) ** (-1)
<< -1
Calculating Roots
Roots are the inverse operation to powers. For example, since 3 squared is 9, the square root of 9 is 3.
Math.sqrt
can be used to return the square root of the number provided as an argument:
Math.sqrt(4)
<< 2
Math.sqrt(100)
<< 10
Math.sqrt(2)
<< 1.4142135623730951
This function will return NaN
if a negative number or non-numerical value is provided as an argument:
Math.sqrt(-1)
<< NaN
Math.sqrt("four")
<< NaN
But watch out, because JavaScript will attempt to coerce the type:
Math.sqrt('4')
<< 2
Math.sqrt(true)
<< 1
Math.cbrt
returns the cube root of a number. This accepts all numbers — including negative numbers. It will also attempt to coerce the type if a value that’s not a number is used. If it can’t coerce the value to a number, it will return NaN
:
Math.cbrt(1000)
<< 10
Math.cbrt(-1000)
<< -10
Math.cbrt("10")
<< 2.154434690031884
Math.cbrt(false)
<< 0
It’s possible to calculate other roots using the exponentiation operator and a fractional power. For example, the fourth root of a number can be found by raising it to the power one-quarter (or 0.25). So the following code will return the fourth root of 625:
625 ** 0.25
<< 5
To find the fifth root of a number, you would raise it to the power of one fifth (or 0.2):
32 ** 0.2
<< 2
In general, to find the nth root of a number you would raise it to the power of 1/n
, so to find the sixth root of a million, you would raise it to the power of 1/6:
1000000 ** (1/6)
<< 9.999999999999998
Notice that there’s a rounding error here, as the answer should be exactly 10. This will often happen with fractional powers that can’t be expressed exactly in binary. (You can read more about this rounding issue in “A Guide to Rounding Numbers in JavaScript“.)
Also note that you can’t find the roots of negative numbers if the root is even. This will return NaN
. So you can’t attempt to find the 10th root of -7, for example (because 10 is even):
(-7) ** 0.1 // 0.1 is 1/10
<< NaN
One reason you might want to calculate roots is to work out growth rates. For example, say you want to 10x your profits by the end of the year. How much do your profits need to grow each month? To find this out, you’d need to calculate the 12th root of 10, or 10 to the power of a twelfth:
10 ** (1/12)
<< 1.2115276586285884
This result tells us that the monthly growth factor has to be around 1.21 in order to 10x profits by the end of the year. Or to put it another way, you’d need to increase your profits by 21% every month in order to achieve your goal.
Logs and Exponentials
Logarithms — or logs for short — can be used to find the exponent of a calculation. For example, imagine you wanted to solve the following equation:
2ˣ = 100
In the equation above, x
certainly isn’t an integer, because 100 isn’t a power of 2. This can be solved by using base 2 logarithms:
x = log²(100) = 6.64 (rounded to 2 d.p.)
The Math
object has a log2
method that will perform this calculation:
Math.log2(100)
<< 6.643856189774724
It also has a log10
method that performs the same calculations, but uses 10 as the base number:
Math.log10(100)
<< 2
This result is telling us that, to get 100, you need to raise 10 to the power of 2.
There’s one other log method, which is just Math.log
. This calculates the natural logarithm, which uses Euler’s number — e
(approximately 2.7) — as the base. This might seem to be a strange value to use, but it actually occurs often in nature when exponential growth happens — hence the name “natural logarithms”:
Math.log(10)
<< 4.605170185988092
Math.log(Math.E)
<< 1
The last calculation shows that Euler’s number (e
) — which is stored as the constant Math.E
— needs to be raised to the power of 1 to obtain itself. This makes sense, because any number to the power of 1 is in fact itself. The same results can be obtained if 2 and 10 are provided as arguments to Math.log2
and Math.log10
:
Math.log2(2)
<< 1
Math.log10(10)
<< 1
Why would you use logarithms? It’s common when dealing with data that grows exponentially to use a logarithmic scale so that the growth rate is easier to see. Logarithmic scales were often used to measure the number of daily COVID-19 cases during the pandemic as they were rising so quickly.
If you’re lucky enough to have a website that’s growing rapidly in popularity (say, doubling every day) then you might want to consider using a logarithmic scale before displaying a graph to show how your popularity is growing.
Hypotenuse
You might remember studying Pythagoras’ theorem at school. This states that the length of the longest side of a right-angled triangle (the hypotenuse) can be found using the following formula:
h² = x² + y²
Here, x and y are the lengths of the other two sides.
The Math
object has a hypot
method that will calculate the length of the hypotenuse when provided with the other two lengths as arguments. For example, if one side is length 3 and the other is length 4, we can work out the hypotenuse using the following code:
Math.hypot(3,4)
<< 5
But why would this ever be useful? Well, the hypotenuse is a measure of the shortest distance between two points. This means that, if you know the x and y coordinates of two elements on the page, you could use this function to calculate how far apart they are:
const ship = {x: 220, y: 100}
const boat = {x: 340, y: 50}
const distance = Math.hypot(ship.x - boat.x,ship.y - boat.y)
I hope that this short roundup has been useful and helps you utilize the full power of the JavaScript Math object in your projects.
Related reading:
- How to Generate Random Numbers in JavaScript with Math.random()
- A Guide to Rounding Numbers in JavaScript
Frequently Asked Questions (FAQs) about JavaScript Math Functions
What is the purpose of the Math object in JavaScript?
The Math object in JavaScript is a built-in object that has properties and methods for mathematical constants and functions. It’s not a function object, rather, it’s a static object. This means you don’t create a Math object of your own, nor do you call it as a method. Instead, you simply call its properties and methods statically. For example, you can use Math.PI to get the value of pi or Math.sqrt(9) to get the square root of 9.
How can I use the Math.random() function in JavaScript?
The Math.random() function in JavaScript is used to generate a random number between 0 (inclusive) and 1 (exclusive). This function does not need any arguments and it returns a floating-point, pseudo-random number. If you want to generate a random number within a specific range, you can do so by multiplying the result by the range size and then adding the range start. For example, to get a random number between 1 and 10, you can use: Math.floor(Math.random() * 10) + 1.
What is the difference between Math.round(), Math.ceil(), and Math.floor() in JavaScript?
These three methods in JavaScript are used to round numbers. Math.round() rounds a number to the nearest integer. If the fractional part is 0.5 or greater, it rounds up, otherwise, it rounds down. Math.ceil() always rounds a number up to the nearest integer, regardless of the fractional part. Math.floor() always rounds a number down to the nearest integer, again regardless of the fractional part.
How can I use the Math.pow() function in JavaScript?
The Math.pow() function in JavaScript is used to raise a number to a power. It takes two arguments: the base and the exponent. For example, to calculate 2 raised to the power of 3, you can use: Math.pow(2, 3), which will return 8.
What is the use of the Math.sqrt() function in JavaScript?
The Math.sqrt() function in JavaScript is used to calculate the square root of a number. It takes one argument, the number you want to find the square root of. For example, to find the square root of 9, you can use: Math.sqrt(9), which will return 3.
How can I use the Math.abs() function in JavaScript?
The Math.abs() function in JavaScript is used to get the absolute value of a number. It takes one argument, the number you want to find the absolute value of. For example, to find the absolute value of -5, you can use: Math.abs(-5), which will return 5.
What is the purpose of the Math.sin(), Math.cos(), and Math.tan() functions in JavaScript?
These functions in JavaScript are used to perform trigonometric calculations. Math.sin() returns the sine of a number, Math.cos() returns the cosine, and Math.tan() returns the tangent. All these functions take one argument, an angle in radians.
How can I use the Math.log() function in JavaScript?
The Math.log() function in JavaScript is used to get the natural logarithm (base e) of a number. It takes one argument, the number you want to find the logarithm of. For example, to find the natural logarithm of 10, you can use: Math.log(10).
What is the purpose of the Math.max() and Math.min() functions in JavaScript?
These functions in JavaScript are used to find the largest and smallest numbers in a list, respectively. They can take any number of arguments. For example, to find the largest number among 1, 2, and 3, you can use: Math.max(1, 2, 3), which will return 3.
How can I use the Math.exp() function in JavaScript?
The Math.exp() function in JavaScript is used to get e (Euler’s number, approximately 2.718) raised to a power. It takes one argument, the power to raise e to. For example, to calculate e raised to the power of 1, you can use: Math.exp(1), which will return approximately 2.718.
Darren loves building web apps and coding in JavaScript, Haskell and Ruby. He is the author of Learn to Code using JavaScript, JavaScript: Novice to Ninja and Jump Start Sinatra.He is also the creator of Nanny State, a tiny alternative to React. He can be found on Twitter @daz4126.