Key Takeaways
- The article discusses the problem of inserting a script into the DOM head tag dynamically when the page is being loaded using JavaScript/jQuery. The author provides solutions for issues such as IE8 causing Error Messages due to an encrypted page/website, loading a second script that references variables created by the first script leading to undefined error messages, and document.write causing page to refresh.
- The author provides a final script that works in all browsers, including IE8. This script checks if the page is not encrypted before loading the OpenX script and checks for the existence of the variable before using it, thus avoiding errors.
- The FAQs section emphasizes the importance of inserting scripts into secure encrypted pages to maintain the security and integrity of the website. It also provides information about jQuery, tips for ensuring script security, common mistakes when inserting scripts into secure encrypted pages, and ways to debug and optimize jQuery scripts for performance.
- IE8 causing Error Messages due to an encrypted page/website – To fix this we can do a check for an encrypted page and only load the OpenX script for pages that aren’t encrypted.
- Loading a second script that references variables created by the first script leading to undefined error messages – To fix this we just added an if statement to check for the existence of the variable before using it.
- document.write causing page to refresh – To fix do not use document.write after the page has been loaded.
(function() {
if (window.location.protocol !== 'https') {
var openx = document.createElement('script'); openx.type = 'text/javascript'; openx.async = true;
openx.src = '';
//Insert into head
var theHead = document.getElementsByTagName('head')[0];
theHead.appendChild(openx);
console.log('script inserted into head');
}
})();
And if you want to include multi-line js script in the head (ie – not just a .js file) you can do it this way.
(function() {
if (window.location.protocol !== 'https:') {
/*Create dynamic scripts*/
var openX = document.createElement('script');
openX.type = 'text/javascript';
openX.defer = 'defer'; /*defer is only supported by IE*/
openX.async = true; /*async is a html5 proposal*/
openX.src = '';
var multiOpenX = document.createElement('script');
multiOpenX.type = 'text/javascript';
multiOpenX.defer = 'defer'; multiOpenX.async = true;
multiOpenX.innerHTML =
['var OX_4ddf11d681ca9 = OX();',
'OX_4ddf11d681ca9.addPage("2400");',
'OX_4ddf11d681ca9.fetchAds();'
].join('n');
/*Insert into head tag*/
var theHead = document.getElementsByTagName('head')[0];
theHead.appendChild(openX);
theHead.appendChild(multiOpenX);
}
})();
IE8 seems to produce errors when using the innerHTML tag in the head section. I cannot see a solution to this except from an alternative approach which doesn’t use innerHTML. We could revert to jQuery.getScript() and then pass in the second script parameters once the first has loaded like so:
$.getScript('ajax/test.js', function() {
alert('Load was performed.');
});
Or even put it into a function and call it from the body like so:
function LoadMyJs(scriptName) {
var theHead = document.getElementsByTagName("head")[0];
var dynamicScript = document.createElement("script");
dynamicScript.type = "text/javascript";
dynamicScript.src = scriptName;
theHead.appendChild(newScript);
}
You can also write the multi-line script this way (warning: some browsers will insert newlines at the continuance, some will not).
var multiOpenX =
'
var OX_4ddf23d681ca9 = OX();
OX_4ddf231181ca9.addPage("2400");
OX_4ddf231181ca9.fetchAds();
';
Final Script
Final script that worked in ALL browsers including IE8:
if (window.location.protocol !== 'https:') {
/*load the OpenX script*/
document.write(unescape('%3Cscript src="<path to script>" type="text/javascript"%3E%3C/script%3E'));
}
if(typeof OX === 'function')
{
var OX_4ddf23d681119 = OX();
OX_4ddf23d681119.addPage("2400");
OX_4ddf23d681119.fetchAds();
}
Also check here for referncing different checks in the URL: http://www.jquery4u.com/javascript/javascript-location-hostnames-url-examples/
Frequently Asked Questions (FAQs) about Inserting Scripts into Secure Encrypted Pages
What is the importance of inserting scripts into secure encrypted pages?
Inserting scripts into secure encrypted pages is crucial for maintaining the security and integrity of your website. Scripts, particularly those written in jQuery, can manipulate HTML content, handle events, create animations, and perform many other functions that enhance the user experience. However, if these scripts are not inserted into secure encrypted pages, they can be exploited by hackers to inject malicious code, steal sensitive data, or disrupt the website’s functionality. Therefore, it’s essential to ensure that your scripts are inserted into secure encrypted pages to protect your website and its users.
How can I ensure that my scripts are secure?
Ensuring the security of your scripts involves several steps. First, always use HTTPS (Hypertext Transfer Protocol Secure) for your website. This encrypts the data transferred between the user’s browser and your website, protecting it from interception. Second, validate all user input to prevent script injection attacks. This involves checking that the input is of the expected type and format before processing it. Third, use Content Security Policy (CSP) headers to restrict which scripts can run on your website. This prevents the execution of unauthorized scripts.
What is jQuery and why is it used?
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, and animation much simpler with an easy-to-use API that works across a multitude of browsers. With a combination of versatility and extensibility, jQuery has changed the way that millions of people write JavaScript.
How can I encrypt my jQuery scripts?
Encrypting your jQuery scripts can be done using various methods. One common method is to use a JavaScript obfuscator, which transforms your code into an equivalent, but harder to understand format. This can deter hackers from trying to reverse-engineer your code. However, it’s important to note that this does not provide absolute security, as determined hackers can still deobfuscate the code with enough effort. Therefore, it’s also crucial to follow other security best practices, such as using HTTPS and validating user input.
How does jQuery work?
jQuery works by providing a simple and consistent interface for interacting with HTML documents. It abstracts many of the complexities of JavaScript, allowing you to write less code to achieve the same results. For example, you can use jQuery to select elements, handle events, create animations, and perform AJAX requests with just a few lines of code. jQuery also handles many of the cross-browser compatibility issues that can arise when writing JavaScript, making your code more robust and reliable.
Can I use jQuery to encrypt and decrypt data?
Yes, you can use jQuery in conjunction with a cryptography library, such as CryptoJS, to encrypt and decrypt data. This can be useful for protecting sensitive data, such as passwords or credit card numbers. However, it’s important to note that client-side encryption should not be the only security measure you use. It should be complemented with server-side encryption and other security practices, such as using HTTPS and validating user input.
How can I learn more about jQuery?
There are many resources available for learning jQuery. The official jQuery website provides comprehensive documentation, tutorials, and examples. There are also many online courses, books, and tutorials available from various sources. Additionally, websites like StackOverflow and the jQuery forum are great places to ask questions and learn from other developers.
What are some common mistakes when inserting scripts into secure encrypted pages?
Some common mistakes when inserting scripts into secure encrypted pages include not using HTTPS, not validating user input, and not using Content Security Policy headers. These mistakes can leave your website vulnerable to script injection attacks, where hackers insert malicious code into your scripts. It’s also a common mistake to rely solely on client-side encryption for security. While client-side encryption can provide an additional layer of protection, it should be complemented with server-side encryption and other security practices.
How can I debug my jQuery scripts?
Debugging your jQuery scripts can be done using the developer tools in your web browser. These tools allow you to inspect the HTML, CSS, and JavaScript of your website, set breakpoints, step through your code, and view any errors or warnings. There are also many jQuery plugins available that can help with debugging, such as jQuery Debugger and FireQuery.
How can I optimize my jQuery scripts for performance?
Optimizing your jQuery scripts for performance can involve several strategies. First, try to minimize the number of DOM manipulations, as these can be expensive in terms of performance. Second, use event delegation to handle events for multiple elements with a single event handler. Third, use the .ready() method to ensure that your scripts only run once the DOM is fully loaded. Finally, consider using a minifier to reduce the size of your scripts, which can improve load times.
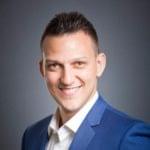
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.