How to Conduct Cold Email Outreach like a Pro
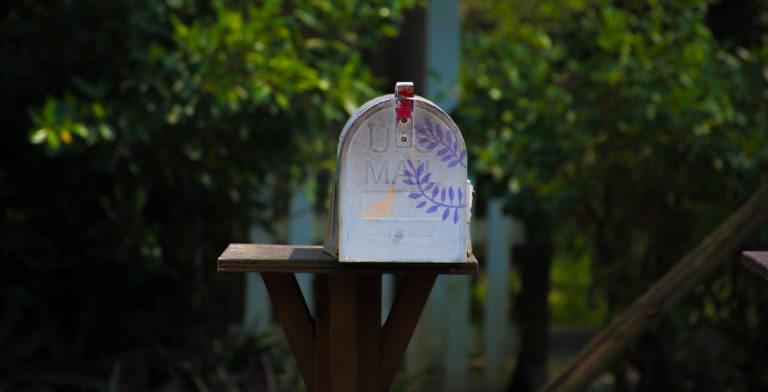
Key Takeaways
- Cold emailing, when done correctly, can be an effective and cost-efficient marketing channel. It’s important to research and connect with the recipient on a personal level, offering value to their business.
- To find leads, define your target industry, job profile, and country. Use tools like Google search, LinkedIn, and email finder services like Hunter, Lusha, and Voila Norbert. Avoid general email addresses or contact forms.
- When writing the first email, start with an informal conversation and highlight ways your skills can add value to their business. Always end the email with a call to action to increase response rates.
- Persistence is key in cold emailing. Follow a 3-7-7 formula for follow-ups, sending the first follow up email 3 days after the initial email, then two more reminders spaced 7 days apart. Even if a prospect declines, keep them in your network for potential future opportunities.
In the grand scheme of marketing things, email is hard not to think of as an antiquated mode. But consider this — over 300 million emails are sent every minute of every day. And according to Marketing Sherpa, 91% of study respondents reported that they like to receive promotional mails from the companies they do business with. That is proof enough that email is not only alive, but kicking as well and by all indications, it will continue to do so in the foreseeable future.
That being said, there’s a big difference between traditional email marketing, where you collect someone’s email through a form, and cold emailing, where you send a person an email without an opt-in.
When it’s done correctly, a cold mail is not spam.
A well thought-out, targeted cold mail to the right person can not only get results, but it beats the heck out of most marketing channels as far as cost-effectiveness goes. I have been working full-time as a freelance marketing consultant and content writer for the past two years, and I part-timed as one for over four years prior when I was employed. I have relied exclusively on cold emailing to get clients, and I can tell you without a shadow of doubt that it just plain works! Here’s how I would do it…
The Right Mindset
Predominantly, people are given to two distinct frames of mind when it comes to cold mailing (or even cold calling). Either they cringe at the thought of contacting someone they don’t know and shun the idea. Or, they shamelessly spam everyone without any regard for the recipient’s privacy or interest. Both are the products of misconceptions.
Firstly, there is nothing wrong with contacting someone if your services can add value to their business. In fact, your prospects will appreciate you for saving them the trouble of finding a solution themselves. However, pestering your prospects over and over again will earn your mail a spot in their spam folder real quick.
To make cold mailing work, you will want to get to know the person you are sending the email to. Take the time to look at their work. Have they written an article you enjoyed reading? Did they just get promoted? Was a recent product from their company a success? Did they leave an interesting quote or post on social media? Instead of just telling them all that’s great about you, find a reason to connect with them and start a conversation. Then take it up from there.
Finding Leads
Firstly, you will need to decide who is it that you wish to reach out to. Start by making a list of the industry, job profile and country you want to target. As a developer with experience and interest in real estate, for instance, you might create a profile such as this…
Industry: Real Estate
Job profile(s): chief technology officer, webmaster
Country: USA/Australia/UK
Now that we know who to target, let’s go out and find the right people!
Google search is a great place to start. Simply type in “Real estate companies in [name of place]” and note the name of the companies along with their websites. If you are targeting companies in other countries, Google will typically show local results rather than those of the place you want. You can use a proxy service like proxysite.com or a VPN such as HMA to help you out here.
Next, go to each website and look for a person fitting the job description. Typically this can either be found in their About Us or Our Team page. Oftentimes, the contact details of a person won’t be found at their websites. In such cases, you can look them up on LinkedIn. Being a professional network, almost every employed person has a profile on there, as do most companies. If they do have a profile, consider adding them as a connection first. You will get a better response if you personalize the message to the recipient, so try it out.
You can use services such as Hunter, Lusha and Voila Norbert to find email addresses as well. Enter the company website in Hunter search and it will show you all the publicly listed email addresses of that domain.
Lastly, avoid general mail ids such as info@, hello@ etc or contact forms on the website. Mails to these are either rejected, or will get poor response rates. Use them only as a last resort.
Breaking the Ice: Writing Your First Mail
Now that you have a contact list ready, it’s time to approach them. Writing your first mail need not cost you a night’s sleep. Outreach becomes a lot easier if you start off with an informal conversation and become acquainted with the person first.
Follow your prospects on social media and see what they are posting. This takes more time than sending a pitch right off the bat, but it’s worth it. Also, keep a keen eye out for anything on the client side related to your profession. If you are a web developer then note how their website looks and functions. Is there something you can do that will add value to the business?
Let’s say my prospect, John, works as the marketing manager for a mid-sized real estate investment firm and has published an article on the company blog which I liked. I would send a message like this…
Subject: Quick question
Hi John,
I enjoyed reading your article <<article name>> on your company’s blog. Your views on <<special observation>> were particularly interesting as <<why they were interesting>>.
I am a marketing consultant and content writer with a keen interest in real estate. In fact, I had a couple of ideas for your blog. Let me know if you’d like me to send them to you.
I am also a contributor at <<authority sites>> and have worked with some of the biggest names in the field, such as <<business names>>.
Some articles on real estate I wrote that you might find interesting…
<<Sample article links>>
I am skilled at creating blog posts, long form articles, website content, press releases, white papers and e-books specific to real estate. Please let me know if you’d like me to send my rates or more samples.
Are you available for a quick 10 minute call later this week so that I can share some of my ideas with you?
Thanks for your time!
Kind regards,
Parth Misra
Now, understandably, there are people of different persuasions and skillsets, and most of them cannot use this mail. However, you only need to change some of the sentences to suit your background — the structure can remain the same.
For instance, a web developer can point out an interesting feature on the website, instead of an article. Instead of sample articles, add links to projects you have worked on.
Tell them how your skills can add value to their business and how upgrading their website can make it more responsive, user-friendly or functional. If you found an area of their web presence you can improve, point it out in the mail, followed by your solution.
Finally, always make sure you are ending your mail with a call to action. This can dramatically increase your response rates.
Follow Up!
The real reason why cold mailing does not work is not because people get their first mail wrong, but rather because they give up after their first attempt. Too many things can go wrong when you’re cold mailing — your email may have gotten buried beneath other messages, your prospect may forget to reply or maybe your mail got sent to the spam folder. Follow-ups helps serve as a reminder should any of these scenarios present themselves.
Bamidele Onibalusi of Writers in Charge suggests a 3-7-7 formula where you send the first follow up email 3 days after your first mail, then send 2 more reminders spaced 7 days apart. I have been using this formula and can vouch for its effectiveness.
The messages need not be fancy, just ask them if they received your mail or if they have had a chance to read it. Here’s a sample…
Hi <<first name>>,
Just wanted to touch base with you regarding my last mail. I thought of checking with you just to be certain you received it.
Thanks!
Kind regards,
Parth Misra
Most of the responses to my mails have come on the second or third mail, so I can tell you from experience that following up is crucial!
Dealing with Responses
Most responses will fall into one of the following categories…
- Interested, please send rates/let’s talk.
- Not in the office, will be back by <<date>>.
- Not right now, maybe in the future.
- Thanks, but not interested.
While the response for the first case is pretty straight forward (follow up with your rates and setup a call), in the second situation, send a mail on the date mentioned saying you reached out to them while they were away.
Situations c and d are a different story though. It’s easy to think that if someone has declined, you should just call it quits. However, just because your prospect is not interested right now, it does not mean they may never need your services. Also, we cannot expect people to simply remember us in the future because we mailed them a couple of times.
So, instead of just forgetting about a prospect if they say no/not right now, send a mail thanking them for their time and ask if you can add them as a connection on LinkedIn, Facebook or Twitter.
By connecting with them on social media, you can stay on top of their minds by posting interesting, relevant content there. When a requirement does crop up, there’s a good chance you will hear from them. Here’s an email I would use for responses c and d…
Hi <<first name>>,
I understand completely, thank you for your time anyway. Please do let me know if you have any content development needs in the future — I will be glad to be of service.
Also, do you mind if I add you as a connection on LinkedIn, if you are on there? I’d love to stay in touch with you!
Kind Regards,
Parth Misra
Final Thoughts
While a strategy can dramatically increase the odds of receiving positive feedback, cold mailing is still a numbers game. To get results, you will have to commit an hour or two every day to cold mailing. Turn it into an exercise and don’t give up if you don’t hear back after your first few attempts. Remember that with cold emailing, we are trying to start a dialogue with a prospect, not close a deal. Keep at it and soon enough, you will have more work than you can handle!
To learn more about running a successful freelance business, check out our book, The Principles of Successful Freelancing.
Frequently Asked Questions about Cold Email Outreach
What is the best way to personalize a cold email outreach?
Personalization is key in cold email outreach. It’s not just about addressing the recipient by their name, but also about understanding their needs, interests, and challenges. Research about the recipient’s company, their role, and their industry. Use this information to tailor your email, making it relevant and valuable to them. Show them that you’ve taken the time to understand their business and that you’re offering a solution to a problem they’re facing.
How can I increase the response rate of my cold emails?
Increasing the response rate of your cold emails requires a combination of effective subject lines, personalized content, and appropriate follow-ups. Your subject line should be compelling and intriguing to prompt the recipient to open the email. The content should be concise, relevant, and provide value to the recipient. Lastly, don’t forget to follow up. Sometimes, emails get lost in the inbox clutter, and a gentle reminder can prompt a response.
What are some common mistakes to avoid in cold email outreach?
Some common mistakes in cold email outreach include sending generic emails, not personalizing your message, and not following up. Avoid sending the same email to all your prospects. Instead, tailor your message to each recipient. Also, ensure that your email provides value to the recipient. Lastly, don’t forget to follow up. Persistence often pays off in cold email outreach.
How can I ensure my cold emails don’t end up in the spam folder?
To avoid your cold emails ending up in the spam folder, ensure that you’re not using spam trigger words in your subject line or email body. Also, use a reputable email service provider, maintain a clean email list, and always include a clear and easy way for recipients to opt-out of your emails.
How can I measure the success of my cold email outreach?
The success of your cold email outreach can be measured using various metrics such as open rate, response rate, and conversion rate. The open rate shows how many recipients opened your email, the response rate shows how many recipients replied to your email, and the conversion rate shows how many recipients took the desired action after reading your email.
How often should I follow up on my cold emails?
The frequency of follow-ups depends on the nature of your email and the recipient’s response. However, a good rule of thumb is to follow up 3-7 days after your initial email if you haven’t received a response. Remember, persistence is key in cold email outreach.
What should I include in my cold email outreach?
Your cold email should include a compelling subject line, a personalized introduction, a clear and concise body that provides value to the recipient, and a clear call-to-action. Also, don’t forget to include your contact information.
How can I make my cold emails stand out?
To make your cold emails stand out, use a compelling subject line, personalize your message, provide value to the recipient, and use a professional and friendly tone. Also, ensure that your email is well-formatted and free of grammatical errors.
What is the best time to send cold emails?
The best time to send cold emails depends on your target audience. However, studies have shown that emails sent on Tuesday, Wednesday, and Thursday have the highest open rates. Also, emails sent in the early morning or late afternoon tend to have better response rates.
How can I improve my cold email outreach strategy?
To improve your cold email outreach strategy, continuously test and optimize your emails. Experiment with different subject lines, email content, and sending times. Also, always measure your results and use this data to inform your strategy.
Parth is a freelance writer and certified content marketing specialist. He loves writing on technology, renewable energy, fitness and marketing. A value driven marketing evangelist, he has a knack for finding unique and unconventional ways to connect brands with their audiences using entertaining, informative content. Find out more about him at his website - Quill Canvas.