Building a Twitter Hashtag Contest – Registering the App
Key Takeaways
- Hosting a contest on Twitter can significantly enhance user interaction and promote a brand or application. This can be achieved by creating a hashtag contest that generates a winner based on user effort and performance, rather than luck.
- To start building a Twitter hashtag contest, you need to register an application on Twitter with write access. Use the OAuth standard technique to authenticate access to the Twitter API. A library called tmhOAuth can be used to automate this process.
- The contest process involves users authenticating their Twitter account, creating tweets with specific hashtags through the application, and selecting winners based on the number of retweets. The winner will be picked based on the total number of retweets for the tweets the users create.
- After setting up the initial details of the TwitterHashTagContest class and initializing the tmhOAuth library, move into the Twitter authorization process. Generate request tokens to initialize user authorization, then generate user-specific request credentials (access tokens) by validating the request tokens.
Introduction
Social networking sites play a vital role in increasing the popularity of a website or application. The vast majority of web sites provide the ability to share their content on popular social media sites with the click of a button. Organizing a contest on these sites is another excellent way of promoting your application.
We are going to build a contest on Twitter to enhance user interaction with a web application. This will be a two part series where the first part focuses on introducing you to Twitter contests and configuring a Twitter application, while the second part will focus on the implementation of the contest. Let’s get started.
Introduction to Contests in Twitter
Twitter is a social networking and micro blogging service utilizing instant messaging, SMS or a web interface. Twitter is considered to be the SMS of web, so contests on Twitter will usually be different from contests on other popular social networking sites.
The main goal of a Twitter contest is to get people to create as many tweets as possible about a certain topic to improve awareness. Let’s take a look at some of the most popular types of contests using Twitter.
Sweepstakes – This is the simplest type of Twitter contests. Users can enter the contest by creating a tweet with a given #tag and their username. The winner will be picked through a lucky draw.
Creative Answer – In this contest, organizers ask a question by creating a tweet or publishing the question on their website. User have to create tweets with a given #tag to answer the question. The most creative answer will be chosen manually by the organizers.
Retweet to win – Organizers create a tweet and users have to retweet it. The winner will be picked randomly from all the retweeters.
Follow to win – Organizers provide a company profile or the profile they want to promote. Users have to be a follower of given profile. Picking the winners is usually done randomly at the end of the contest.
Instead of relying entirely on luck, we’ll create a hashtag contest that generates a winner based on the effort and performance of the users.
Planning a Twitter Hashtag Contest
Here are the steps of the contest:
Users need to authenticate their Twitter account – Usually, this is done by providing a Twitter login button where users can authorize access to the application through their Twitter account.
Create Tweets with Hashtags through our application – We are going to use two hashtags with each tweet. The first tag is used to promote the brand. If our brand is SitePoint, we will have a hashtag called
#SitePoint
to promote our brand. The second tag is used to identify the application amongst the other tweets with the first hashtag.Select the winners – There are many ways of picking winners automatically in such contests. In this case, we are going to evaluate winners based on the number of retweets. The winner will be picked based on the total number of retweets for the tweets the users create.
Building
To begin, you need to register an application on Twitter. Visit the Twitter Developer site and create an application with write access. Once you have a Twitter application with consumer_key
and consumer_secret
, follow the instructions in the step by step guide to complete the implementation.
Step 1 – Configuring the OAuth Library
OAuth is the standard technique used by Twitter to authenticate access to their API. There are many existing libraries for automating the authentication process with Twitter. We are going to use a library called tmhOAuth. Download and extract the zip file from Github, or clone it.
Create the application folder with an index.php
file inside it and copy the contents of tmhOAuth library into a folder called twitter
. Then include the tmhOAuth.php
file inside the index.php
file (see below).
Step 2 – Authenticating Users with Twitter
First, users have to authorize the app using their Twitter account. We need a button or link that redirects the user to the authorization process. We are going to use a separate class for managing the logic of this application. Create a file called twitter_hashtag_contest.php
inside the application folder. Now let’s take a look at the updated index.php
file.
<?php
require 'twitter/tmhOAuth.php';
require 'twitter_hashtag_contest.php';
session_start();
$contest = new TwitterHashTagContest();
if(isset($_GET['action']) && $_GET['action'] == 'twitter'){
$contest->getRequestToken();
} else {
echo "<a href='?action=twitter'>LogIn with Twitter</a>";
}
We can display the login link by default. Once the login link is clicked, we have to redirect the user to Twitter for app authorization and retrieving the request tokens. We have to set up the initial details of TwitterHashTagContest
class, before we move into the implementation of getRequestToken
function.
Step 3 – Initializing TwitterHashTagContest Class
We are using TwitterHashTagContest
class to handle all the application specific details, so let’s look at the initialization and configuration for said class.
class TwitterHashTagContest{
private $config;
private $twitter_auth;
private $app_details;
private $result_tweets;
public function __construct(){
$this->config['consumer_key'] = 'consumer_key';
$this->config['consumer_secret'] = 'consumer_secret';
$this->config['OAUTH_CALLBACK']='URL to index.php file';
$this->twitter_auth = new tmhOAuth($this->config);
$this->result_tweets = array();
}
}
We can start the configuration by defining consumer_key
,consumer_secret
and callback URL in an array. Then we can initialize the tmhOAuth
library using the configuration array. Finally, we initialize an array for storing the results at the completion of the contest.
Having completed configuration details, now we can move into the Twitter authorization process.
Step 4 – Generate Request Tokens
First, we have to get request tokens to initialize the user authorization process. Twitter provides the API URL oauth/request_token
for generating request tokens. Let’s take a look at the implementation of the getRequestToken
function defined earlier.
public function getRequestToken() {
$this->twitter_auth->request("POST", $this->twitter_auth->url("oauth/request_token", ""), array(
'oauth_callback' => $this->config['OAUTH_CALLBACK']
));
if ($this->twitter_auth->response["code"] == 200) {
// get and store the request token
$response = $this->twitter_auth->extract_params($this->twitter_auth->response["response"]);
$_SESSION["authtoken"] = $response["oauth_token"];
$_SESSION["authsecret"] = $response["oauth_token_secret"];
// redirect the user to Twitter to authorize
$url = $this->twitter_auth->url("oauth/authenticate", "") . '?oauth_token=' . $response["oauth_token"];
header("Location: " . $url);
exit;
}
return false;
}
We already have an instance of the tmhOAuth
class, initialized within the constructor of TwitterHashTagContest
. We have to use the request method of tmhOAuth
to access Twitter’s API. This function takes 3 arguments where the first one defines the request type (POST or GET) and the next two arguments define the API endpoint URL and parameters respectively.
We are using the oauth/request_token
API URL to generate the tokens. The response will contain the request tokens as well as the status of the request. Code 200 means a successful completion of the request and we proceed by using the extract_params
function to extract all the parameters from the response into an array. Next, we store the oauth_token
and oauth_token_secret
in the current session.
Finally, we redirect the user for authorization using oauth/authenticate
or oauth/authorize
.
We can use either oauth/authenticate
or oauth/authorize
to handle the authorization process. oauth/authorize
requires the user to authorize the application on each request, even if it’s already authorized for the user. oauth/authenticate
differentiates from oauth/authorize
by avoiding authorization on each request. We have to tick the Allow this application to be used to Sign in with Twitter checkbox on the Settings tab of our Twitter application to allow this.
Once redirected, users can log in and authorize the application, then Twitter will automatically redirect the request to the callback URL. Next, we have to generate user specific request credentials (access tokens) by validating the request tokens.
Step 5 – Generate Access Tokens
First, we have to filter the response retrieved after authorizing the app. Let’s look at the updated code.
if(isset($_GET['action']) && $_GET['action'] == 'twitter'){
$contest->getRequestToken();
} else if(isset($_GET['oauth_token'])) {
$oauth_token = isset($_GET['oauth_token']) ? $_GET['oauth_token'] : '';
$oauth_verifier = isset($_GET['oauth_verifier']) ? $_GET['oauth_verifier'] : '';
$response = $contest->getRequestCredentials($oauth_verifier,$oauth_token);
$contest->createUserSession($response);
} else {
echo "<a href='?action=twitter'>LogIn with Twitter</a>";
}
The response from Twitter contains oauth_token
and oauth_verifier
as URL parameters. We can filter the request using these parameters and call the getRequestCredentials
function to generate the access tokens. The following code illustrates the implementation of getRequestCredentials
.
public function getRequestCredentials($oauth_verifier,$oauth_token){
$this->twitter_auth->request("POST", $this->twitter_auth->url("oauth/access_token", ""), array(
// pass a variable to set the callback
'oauth_verifier' => $oauth_verifier,
'oauth_token' => $oauth_token
));
$response = array();
if($this->twitter_auth->response["code"] == 200) {
$response = $this->twitter_auth->extract_params($this->twitter_auth->response["response"]);
}
return $response;
}
As we did earlier, the POST request is created on $this->twitter_auth
object to access oauth/access_token
API. Here, we are passing the oauth_verifier
and oauth_token
retrieved from previous request for generating the access tokens. Finally, we check the response code to match 200 and return the extracted components of the response object.
Please keep in mind that we are only implementing the success path considering the scope of this tutorial. In a real implementation, we will have to implement the error handling part to filter other response codes as well.
Afterwards, we can use the access tokens and enable tweeting capabilities for the users. We pass the response to a function called createUserSession
, for adding the details to the browser session and initializing the tweeting screen.
In a real implementation, we need to keep the access tokens and the details of the logged in user in the database to retrieve the tokens. Here, we are using browser sessions instead to simplify the tutorial.
Conclusion
Promoting applications through social media contests is an excellent approach. Twitter is one of the easiest platforms to host a contest on, so we started with the goal of building a hashtag contest on Twitter.
So far, we implemented the initial steps to create a Twitter application and authenticate users into our system. In the next part, we will be completing the implementation of this contest by creating the tweet capabilities for the users and generating the results.
Until then, let us know your thoughts about this part!
Frequently Asked Questions about Building a Twitter Hashtag Contest and Registering a Twitter App
How can I ensure my Twitter hashtag contest complies with Twitter’s rules and policies?
Twitter has specific rules and policies for running contests. These include ensuring that your contest doesn’t encourage spammy behavior, such as multiple entries or creating multiple accounts. You should also clearly state the rules of your contest, including eligibility requirements and how winners will be selected. It’s important to follow these rules to avoid having your contest or your account flagged by Twitter.
Can I register a hashtag for my Twitter contest?
While you can’t officially register a hashtag with Twitter, you can create a unique hashtag for your contest. This helps to track entries and engage with participants. Before choosing a hashtag, do a quick search on Twitter to ensure it’s not already in use.
What are some effective strategies for running a Twitter contest?
Some effective strategies include setting clear and simple contest rules, choosing a unique and relevant hashtag, promoting your contest across multiple platforms, and engaging with participants throughout the contest. It’s also important to choose a prize that’s relevant and appealing to your target audience.
How can I use a Twitter app for my hashtag contest?
A Twitter app can help you manage your contest more effectively. You can use it to track entries, monitor your hashtag, and engage with participants. You can also use it to randomly select a winner, ensuring fairness in your contest.
How can I create an effective hashtag campaign for my contest?
An effective hashtag campaign involves more than just choosing a unique hashtag. It also involves promoting your hashtag across multiple platforms, engaging with participants, and monitoring your hashtag to track its performance. You should also consider the timing of your campaign, as well as the relevance of your hashtag to your target audience.
What are the benefits of running a Twitter hashtag contest?
Running a Twitter hashtag contest can help increase your brand visibility, engage with your audience, and attract new followers. It can also help you gather user-generated content, which can be used in future marketing efforts.
How can I promote my Twitter hashtag contest?
You can promote your contest by tweeting about it regularly, sharing it on other social media platforms, and encouraging your followers to share it with their networks. You can also consider partnering with influencers or other brands to reach a wider audience.
How can I track the performance of my Twitter hashtag contest?
You can track the performance of your contest by monitoring your hashtag, tracking the number of entries, and using Twitter’s analytics tools. This can help you understand how your contest is performing and make adjustments as needed.
How can I ensure fairness in my Twitter hashtag contest?
To ensure fairness, clearly state the rules of your contest and how winners will be selected. You can also use a Twitter app to randomly select a winner. Be transparent about the process to maintain trust with your audience.
What should I do after my Twitter hashtag contest ends?
After your contest ends, announce the winner and thank all participants. You can also share some of the best entries or moments from the contest. Analyze the performance of your contest to understand what worked well and what could be improved for future contests.
Rakhitha Nimesh is a software engineer and writer from Sri Lanka. He likes to develop applications and write on latest technologies. He is available for freelance writing and WordPress development. You can read his latest book on Building Impressive Presentations with Impress.js. He is a regular contributor to 1stWebDesigner, Tuts+ network and SitePoint network. Make sure to follow him on Google+.
Published in
·Miscellaneous·Patterns & Practices·Performance & Scaling·PHP·Programming·Web·February 17, 2014
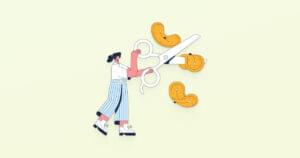
Published in
·automation·CMS & Frameworks·Frameworks·Laravel·Miscellaneous·Patterns & Practices·PHP·October 26, 2015
Published in
·APIs·CMS & Frameworks·Meta·Miscellaneous·Patterns & Practices·PHP·Programming·Web·July 25, 2014