In this article, we’ll investigate the importance of JSON and why we should use it in our applications. We’ll see that jQuery has got us covered with a very nice convenience function.
What is JSON?
JSON stands for JavaScript Object Notation. It’s a language-independent, text-based format, which is commonly used for transmitting data in web applications. In this article, we’ll look at loading JSON data using an HTTP GET request (we can also use other verbs, such as POST).
Why would we choose JSON over say XML? The key advantage of using JSON is efficiency. JSON is less verbose and cluttered, resulting in fewer bytes and a faster parse process. This allows us to process more messages sent as JSON than as XML. Moreover, JSON has a very efficient and natural object representation leading to formats such as BSON, where JSON-like objects are stored in a binary format.
Now, let’s see how jQuery can help us load JSON-encoded data from a remote source. For the impatient among you, there’s a demo towards the end of the article.
JSON jQuery Syntax
The $.getJSON()
method is a handy helper for working with JSON directly if you don’t require much extra configuration. Essentially, it boils down to the more general $.ajax() helper, with the right options being used implicitly. The method signature is:
$.getJSON(url, data, success);
Besides the required URL parameter, we can pass in two optional parameters. One represents the data to send to the server; the other one represents a callback to trigger in case of a successful response.
So the three parameters correspond to:
- the
url
parameter, which is a string containing the URL to which the request is sent - the optional
data
parameter, which is either an object or a string that’s sent to the server with the request - the optional
success(data, textStatus, jqXHR)
parameter, which is a callback function executed only if the request succeeds
In the simplest scenario, we only care about the returned object. In this case, a potential success
callback would look like this:
function success(data) {
// do something with data, which is an object
}
As mentioned, the same request can be triggered with the more verbose $.ajax()
call. Here we would use:
$.ajax({
dataType: 'json',
url: url,
data: data,
success: success
});
Let’s see this in practice using a little demo.
A Sample Application
We’ll start a local server that serves a static JSON file. The object represented by this file will be fetched and processed by our JavaScript code. For the purposes of our demo, we’ll use Node.js to provide the server (although any server will do). This means we’ll need the following three things:
- a working installation of Node.js
- the node package manager (npm)
- a global installation of the live-server package
The first two points are platform-dependent. To install Node, please head to the project’s download page and grab the relevant binaries for your system. Alternatively, you might like to consider using a version manager as described in “Installing Multiple Versions of Node.js Using nvm”.
npm comes bundled with Node, so there’s no need to install anything. However, if you need any help getting up and running, consult our tutorial “A Beginner’s Guide to npm — the Node Package Manager”.
The third point can be achieved by running the following from your terminal:
npm install -g live-server
If you find yourself needing a sudo
prefix (-nix systems) or an elevated command prompt to perform this global installation, you should consider changing the location of global packages.
Once these requirements have been met, we can create the following three files in a new folder:
main.js
, which is the JavaScript file to request the dataexample.json
, which is the example JSON fileindex.html
, which is the HTML page to call the JavaScript and display the data
From the command prompt we can simply invoke live-server
within the new folder. This will open our demo in a new browser tab, running at http://localhost:8080.
The Sample JavaScript
The following code is the complete client-side logic. It waits for the DOMContentLoaded
loaded event to fire, before grabbing a reference to two DOM elements — $showData
, where we’ll display the parsed response, and $raw
, where we’ll display the complete response.
We then attach an event handler to the click
event of the element with the ID get-data
. When this element is clicked, we attempt to load the JSON from the server using $.getJSON()
, before processing the response and displaying it on the screen:
$(document).ready(() => {
const $showData = $('#show-data');
const $raw = $('pre');
$('#get-data').on('click', (e) => {
e.preventDefault();
$showData.text('Loading the JSON file.');
$.getJSON('example.json', (data) => {
const markup = data.items
.map(item => `<li>${item.key}: ${item.value}</li>`)
.join('');
const list = $('<ul />').html(markup);
$showData.html(list);
$raw.text(JSON.stringify(data, undefined, 2));
});
});
});
Besides converting parts of the object to an unordered list, the full object is also stringified and displayed on the screen. The unordered list is added to a <div>
element with the ID show-data
, the JSON string a <pre>
tag, so that it is nicely formatted. Of course, for our example the data is fixed, but in general any kind of response is possible.
Note that we also set some text for the output <div>
. If we insert some (artificial) delay for the JSON retrieval (for example, in your browser’s dev tools), we’ll see that this actually executes before any result of the $.getJSON
request is displayed. The reason is simple: by default, $.getJSON
is non-blocking — that is, async. Therefore, the callback will be executed at some (unknown) later point in time.
Distilling the source to obtain the crucial information yields the following block:
$('#get-data').on('click', () => {
$.getJSON('example.json', (data) => {
console.log(data);
});
});
Here we only wire the link to trigger the start of the $.getJSON
helper before printing the returned object in the debugging console.
The Sample JSON
The sample JSON file is much larger than the subset we care about. Nevertheless, the sample has been constructed in such a way as to show most of the JSON grammar. The file reads:
{
"items": [
{
"key": "First",
"value": 100
},
{
"key": "Second",
"value": false
},
{
"key": "Last",
"value": "Mixed"
}
],
"obj": {
"number": 1.2345e-6,
"enabled": true
},
"message": "Strings have to be in double-quotes."
}
In the sample JavaScript, we’re only manipulating the array associated with the items
key. In contrast to ordinary JavaScript, JSON requires us to place keys in double quotes. Additionally, we can’t use trailing commas for specifying objects or arrays. However, as with ordinary JavaScript arrays, we are allowed to insert objects of different types.
The Sample Web Page
We’ve already looked at the script and the sample JSON file. All that’s left is the web page, which provides the parts being used by the JavaScript file to trigger and display the JSON file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Request JSON Test</title>
</head>
<body>
<a href="#" id="get-data">Get JSON data</a>
<div id="show-data"></div>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="main.js"></script>
</body>
</html>
There’s not much to say here. We use the minified version of jQuery from the official web page. Then we include our script, which is responsible for injecting the logic.
Note: as we’re including our JavaScript files in the correct place (just before the closing </body>
tag), it no longer becomes necessary to use a $(document).ready()
callback, because at this point, the document will be ready by definition.
Demo
And this is what we end up with.
The More General Method
As previously mentioned, the $.ajax
method is the real deal for any (not only JSON-related) web request. This method allows us to explicitly set all the options we care about. We can adjust async
to true
if we want this to call to run concurrently — that is, run potentially at the same time as other code. Setting it to false
will prevent other code from running while the download is in progress:
$.ajax({
type: 'GET',
url: filename,
data: data,
async: false,
beforeSend: (xhr) => {
if (xhr && xhr.overrideMimeType) {
xhr.overrideMimeType('application/json;charset=utf-8');
}
},
dataType: 'json',
success: (data) => {
//Do stuff with the JSON data
}
});
The overrideMimeType
method (which overrides the MIME type returned by the server) is only called for demonstration purposes. Generally, jQuery is smart enough to adjust the MIME-type according to the used data type.
Before we go on to introduce the concept of JSON validation, let’s have short look at a more realistic example. Usually, we won’t request a static JSON file, but will load JSON, which is generated dynamically (for example, as the result of calling an API). The JSON generation is dependent on some parameter(s), which have to be supplied beforehand:
const url = 'https://api.github.com/v1/...';
const data = {
q: 'search',
text: 'my text'
};
$.getJSON(url, data, (data, status) => {
if (status === 200) {
//Do stuff with the JSON data
}
});
Here we check the status to ensure that the result is indeed the object returned from a successful request and not some object containing an error message. The exact status code is API-dependent, but for most GET requests, a status code of 200 is usual.
The data is supplied in the form of an object, which leaves the task of creating the query string (or transmitting the request body) up to jQuery. This is the best and most reliable option.
JSON Validation
We shouldn’t forget to validate our JSON data! There’s an online JSON Validator tool called JSONLint that can be used to validate JSON files. Unlike JavaScript, JSON is very strict and doesn’t have tolerances — for example, for the aforementioned trailing commas or multiple ways of writing keys (with /
, without quotes).
So, let’s discuss some of the most common errors when dealing with JSON.
Common $.getJSON errors
- Silent failures on
$.getJSON
calls. This might happen if, for example,jsoncallback=json1234
has been used, while the functionjson1234()
doesn’t exist. In such cases, the$.getJSON
will silently error. We should therefore always usejsoncallback=?
to let jQuery automatically handle the initial callback. - In the best case, real JSON (instead of JSONP) is used (either by talking with our own server or via CORS). This eliminates a set of errors potentially introduced by using JSONP. The crucial question is: Does the server / API support JSONP? Are there any restrictions on using JSONP? You can find out more about working with JSONP here.
Uncaught syntax error: Unexpected token
(in Chrome) orinvalid label
(in Firefox). The latter can be fixed by passing the JSON data to the JavaScript callback. In general, however, this is a strong indicator that the JSON is malformed. Consider using JSONLint, as advised above.
The big question now is: How do we detect if the error actually lies in the transported JSON?
How to fix JSON errors
There are three essential points that should be covered before starting any JSON-related debugging:
- We have to make sure that the JSON returned by the server is in the correct format with the correct MIME-type being used.
- We can try to use $.get instead of
$.getJSON
, as it might be that our server returns invalid JSON. Also, ifJSON.parse()
fails on the returned text, we immediately know that the JSON is to blame. - We can check the data that’s being returned by logging it to the console. This should then be the input for further investigations.
Debugging should then commence with the previously mentioned JSONLint tool.
Conclusion
JSON is the de-facto standard format for exchanging text data. jQuery’s $.getJSON()
method gives us a nice little helper to deal with almost any scenario involving a request for JSON formatted data. In this article, we’ve investigated some methods and possibilities that come with this handy helper.
If you need help implementing JSON fetching in your code (using $.getJSON()
or anything else), come and visit us in the SitePoint forums.
FAQs About Ajax/jQuery.getJSON
What is the use of getJSON?
The getJSON
method, though not a built-in JavaScript function, plays a crucial role in web development by facilitating the retrieval of JSON data from remote servers or APIs. It is commonly offered by JavaScript libraries and frameworks like jQuery and Axios.
In jQuery, $.getJSON()
serves as a straightforward way to perform asynchronous GET requests, making it easier to interact with APIs. You specify the URL to the resource you want to retrieve JSON data from and define a callback function to handle the received data once the request is successful. This approach is widely used for dynamically loading content into web applications, enabling real-time updates without requiring a full page reload.
On the other hand, Axios, a versatile JavaScript HTTP client, provides the axios.get()
method for making GET requests. It retrieves data in a Promise-based fashion, simplifying the handling of responses. Axios automatically parses JSON data, allowing developers to work with the content seamlessly. This flexibility makes Axios a popular choice for more complex applications, offering greater control and customization over HTTP requests and responses.
In summary, the getJSON
method, or its equivalents in various JavaScript libraries, empowers web developers to fetch JSON data from servers or APIs, making it a fundamental tool for creating dynamic and interactive web applications that rely on external data sources. Whether you opt for jQuery’s simplicity or the comprehensive capabilities of Axios, these methods simplify the process of integrating remote data into your web applications, enhancing user experiences and functionality.
What is the difference between Ajax and getJSON?
Ajax and getJSON
serve distinct purposes in web development, both involving asynchronous HTTP requests but with differences in scope and specialization.
Ajax, short for Asynchronous JavaScript and XML, is a comprehensive approach to making asynchronous requests from a web page to a server. It encompasses various techniques and is not tied to any specific data format. Ajax can handle a wide range of data types, including HTML, XML, JSON, or plain text. It provides developers with granular control over request setup, response processing, and error handling. While this flexibility is valuable for handling diverse scenarios, it often involves more extensive code and configuration.
In contrast, getJSON
is a specialized method primarily offered by JavaScript libraries like jQuery for making HTTP GET requests specifically designed for JSON data. It assumes that the response from the server will be in JSON format, automatically parsing the JSON into a JavaScript object. This simplifies data handling, as developers don’t need to manually parse JSON responses. getJSON
offers a streamlined and concise approach when you know your data is in JSON format, making it a convenient choice for scenarios where JSON is the expected and sole data type. However, it lacks the versatility of Ajax in dealing with different data formats.
The choice between Ajax and getJSON
depends on your specific use case. If you require flexibility to handle various data types and more control over request handling, Ajax is a suitable choice. On the other hand, if you are certain that you are working with JSON data and prefer a simplified and specialized approach, getJSON
can be a more straightforward option for fetching and processing JSON data in your web application.
How to get JSON data from a URL in JavaScript?
To fetch JSON data from a URL in JavaScript, the Fetch API provides a streamlined and versatile method. Here’s how it works:
First, you define the URL of the resource you want to retrieve JSON data from. This URL can be an endpoint on your server or a publicly available API.
Then, you use the fetch(url)
function, passing in the URL as its argument, to initiate an HTTP GET request to that URL. The fetch
function returns a Promise, which allows you to work with the asynchronous response.
You can chain the then
method to the fetch Promise to handle the response. Within this method, you typically check if the response status is OK (HTTP status code 200), as an error could occur if the network connection is unavailable or if the server returns an error status code. If the response is OK, you proceed to parse the response body as JSON using response.json()
. This method also returns a Promise that resolves to the parsed JSON data. Finally, another then
block processes the JSON data, enabling you to manipulate and use it in your JavaScript application. Any errors that occur during the fetch operation are handled in the catch
block, where you can address network issues or invalid JSON responses.
This approach is widely adopted due to its simplicity and asynchronous nature, ensuring that your application remains responsive while fetching data from external sources.

Florian Rappl is an independent IT consultant working in the areas of client / server programming, High Performance Computing and web development. He is an expert in C/C++, C# and JavaScript. Florian regularly gives talks at conferences or user groups. You can find his blog at florian-rappl.de.
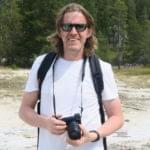
Network admin, freelance web developer and editor at SitePoint.