How you might use jQuery to filter elements by their data Attribute values. This code snippet grabs all divs that have an id beginning with “proto_” and have a data attribute of “state” with value “open”.
var $el = $('div[id^=proto_]').filter(function()
{
return ($(this).data("state") == "open")
});
console.log($el);
I’ve done a quick demo in jsfiddle to demonstrate. https://jsfiddle.net/qgwnY/
Optimised Version
Thanks Vlad.var $el = $('div[id^=proto_]').filter('div[data-state=open]').css('color','red');
Check this out on jsfiddle.
Frequently Asked Questions (FAQs) about jQuery Filter Objects by Data Attribute
How can I use jQuery to filter objects by data attribute?
jQuery provides a powerful way to manipulate and filter objects based on their data attributes. You can use the .filter()
function in jQuery to achieve this. The .filter()
function allows you to specify a function as its parameter, and this function should return either true or false. If the function returns true, the element will be included in the filtered set; if it returns false, it will not be included. Here’s a basic example:$('div').filter(function() {
return $(this).data('key') === 'value';
});
In this example, we’re filtering all div elements that have a data attribute ‘key’ with the value ‘value’.
What is the difference between .filter()
and .find()
in jQuery?
Both .filter()
and .find()
are jQuery methods used to narrow down the search for elements in a jQuery object. The main difference between them is that .filter()
reduces the set of matched elements to those that match the selector or pass the function’s test, while .find()
gets the descendants of each element in the current set of matched elements, filtered by a selector, jQuery object, or element.
Can I use multiple data attributes in the .filter()
function?
Yes, you can use multiple data attributes in the .filter()
function. You just need to add more conditions in the function. Here’s an example:$('div').filter(function() {
return $(this).data('key1') === 'value1' && $(this).data('key2') === 'value2';
});
In this example, we’re filtering all div elements that have a data attribute ‘key1’ with the value ‘value1’ and a data attribute ‘key2’ with the value ‘value2’.
How can I filter elements based on a data attribute value that contains a certain string?
You can use the attribute contains selector [name*="value"]
to select elements whose attribute value contains a specific string. Here’s an example:$('div[data-key*="value"]').filter(function() {
// Your code here
});
In this example, we’re filtering all div elements that have a data attribute ‘key’ whose value contains the string ‘value’.
Can I use .filter()
with other jQuery methods?
Yes, you can chain .filter()
with other jQuery methods. For example, you can use .filter()
with .css()
to change the style of the filtered elements:$('div').filter(function() {
return $(this).data('key') === 'value';
}).css('color', 'red');
In this example, we’re changing the text color to red for all div elements that have a data attribute ‘key’ with the value ‘value’.
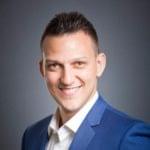
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.