Tab control usability hover triggers in jQuery
Tab control usability hover triggers in jQuery. Tested and works in Chrome, FF, IE9, IE8.
Load is jsfiddle for TAB demo to work.
jsfiddle
The tabindex Property
- The tabindex attribute is supported in all major browsers, except Safari.
- HTML5 – tabindex attribute can be used on any HTML element
jQuery
$(document).ready(function () {
/* "trigger" hover by adding a CSS class */
$('.transition-container').on('mouseover', function (e) {
$(this).find('.transition').addClass('activeHover');
$(this).on('mouseout', function (e) {
$(this).find('.transition').removeClass('activeHover');
});
});
/* get key code */
function getKeyCode(key) {
//return the key code
return (key == null) ? event.keyCode : key.keyCode;
}
/* for usabiity capture keyboard tabbing */
$(document).on('keyup', function (eventObj) {
//tab keycode = 9
if (getKeyCode(eventObj) == '9') {
var $el = $(document.activeElement);
//remove any current active elements
$('.transition').removeClass('activeHover');
if ($el.hasClass('transition-container')) {
$el.find('.transition').addClass('activeHover');
}
}
});
});
/* Internet Explorer sniffer code to add class to body tag for IE version */
var ie = (function () {
var undef,
v = 3,
div = document.createElement('div'),
all = div.getElementsByTagName('i');
while (
div.innerHTML = '',
all[0]);
if (v > 4) {
$('body').addClass('ie' + v);
}
}());
CSS
/* transition speed */
.transition {
-webkit-transition: -webkit-transform .2s ease-out;
-moz-transition: -moz-transform .2s ease-out;
-o-transition: -o-transform .2s ease-out;
-ms-transition: -ms-transform .2s ease-out;
transition: transform .2s ease-out;
}
/* IE 9 scale */
.ie9 .activeHover {
-ms-transform: scale(1.2, 1.2);
}
/* IE 8 scale */
.ie8 .activeHover {
filter: progid:DXImageTransform.Microsoft.Matrix(M11=1.1, M12=0, M21=0, M22=1.1, SizingMethod='auto expand');
}
/* modern browsers transition */
.activeHover {
-webkit-transform:scale(1.2);
-moz-transform:scale(1.2);
-o-transform:scale(1.2);
transform:scale(1.2);
}
/* IE 7 scale */
.ie7 .activeHover {
filter: none;
transform: none;
}
.transition-container {
border: 1px solid blue;
margin: 15px;
padding:5px;
}
*:active {
border: 1px solid red;
}
}
HTML5
Click to get focus and start pressing TAB to switch between items. Press SHIFT+TAB to go back up.
Frequently Asked Questions (FAQs) about Tab Control Usability and Hover Triggers in jQuery
How can I simulate a tab key press with jQuery?
Simulating a tab key press with jQuery can be achieved by triggering a keydown event and passing the keycode for the tab key (which is 9). Here’s a simple example:$(document).keydown(function(e) {
if (e.which == 9) {
// Your code here
}
});
In this code, the keydown event is triggered whenever a key is pressed. If the key pressed is the tab key (keycode 9), the code within the if statement is executed.
How can I trigger a hover event on an anchor tag with jQuery?
You can trigger a hover event on an anchor tag using the hover() function in jQuery. Here’s an example:$("a").hover(function() {
// Code to execute when mouse enters the element
}, function() {
// Code to execute when mouse leaves the element
});
In this code, the hover() function takes two functions as parameters. The first function is executed when the mouse enters the element, and the second function is executed when the mouse leaves the element.
What does the trigger() function do in jQuery?
The trigger() function in jQuery is used to execute all handlers and behaviors attached to the matched elements for the given event type. This can be useful for simulating user interactions for testing purposes. For example, you can trigger a click event on a button like this:$("button").trigger("click");
In this code, the click event handlers attached to the button element are executed.
How can I use the hover() function in jQuery?
The hover() function in jQuery is used to specify two functions to run when the mouse pointer hovers over the selected elements. This function is a shorthand for the combination of mouseenter() and mouseleave() functions. Here’s an example:$("div").hover(function() {
$(this).addClass("hover");
}, function() {
$(this).removeClass("hover");
});
In this code, the hover() function adds the “hover” class to a div when the mouse enters it, and removes the “hover” class when the mouse leaves it.
How can I simulate a tab key press with jQuery for form navigation?
You can simulate a tab key press for form navigation by triggering a keydown event and using the focus() function to move the focus to the next input element. Here’s an example:$("input").keydown(function(e) {
if (e.which == 9) {
$(this).next("input").focus();
}
});
In this code, when the tab key is pressed in an input field, the focus moves to the next input field in the form.
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
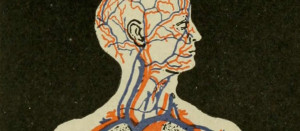
Published in
·Design·Design & UX·Statistics and Analysis·UI Design·Usability·UX·Web·September 1, 2016