Quick example on how to use JavaScript to sort arrays by index values. To analyse the best method of doing this in terms of performance I looked at a JS perf test for sorting objects.
var data = Array();
data[0] = {"apples":1, "pears":2, "oranges":3};
data[1] = {"apples":3, "pears":3, "oranges":5};
data[2] = {"apples":4, "pears":1, "oranges":6};
console.log(data);
data.sort(function(a, b){
var a1= a.pears, b1= b.pears;
if(a1== b1) return 0;
return a1> b1? 1: -1;
});
console.log(data);
Here you can see that we have sorted by “pears” values. The first line is before and second line is after the sort: pear 1, pear 2, pear 3.
JS Sort on Objects
//objects
var array = [{id:'12', name:'Smith', value:1},{id:'13', name:'Jones', value:2}];
array.sort(function(a, b){
var a1= a.name, b1= b.name;
if(a1== b1) return 0;
return a1> b1? 1: -1;
});
JS Sort on Arrays
//arrays
var array =[ ['12', ,'Smith',1],['13', 'Jones',2]];
array.sort(function(a, b){
var a1= a[1], b1= b[1];
if(a1== b1) return 0;
return a1> b1? 1: -1;
});
Frequently Asked Questions (FAQs) about Sorting Arrays in JavaScript
How can I sort an array in descending order in JavaScript?
In JavaScript, the sort() function sorts an array in ascending order by default. However, you can sort an array in descending order by passing a compare function to the sort() function. Here’s an example:let array = [5, 2, 8, 1, 4];
array.sort(function(a, b) {
return b - a;
});
console.log(array); // Output: [8, 5, 4, 2, 1]
In the compare function, if ‘b – a’ returns a positive value, ‘b’ is sorted before ‘a’. This results in the array being sorted in descending order.
How can I sort an array of objects based on a specific property?
To sort an array of objects based on a specific property, you can pass a compare function to the sort() function. The compare function should return the difference between the specific property of two objects. Here’s an example:let array = [
{ name: 'John', age: 23 },
{ name: 'Jane', age: 21 },
{ name: 'Oliver', age: 25 }
];
array.sort(function(a, b) {
return a.age - b.age;
});
console.log(array);
In this example, the array is sorted based on the ‘age’ property of the objects.
Can I use the sort() function to sort an array of strings?
Yes, you can use the sort() function to sort an array of strings. By default, the sort() function sorts strings based on the string Unicode values, which might not always result in the expected order. For example, uppercase letters have lower Unicode values than lowercase letters. Therefore, ‘Z’ would be sorted before ‘a’. If you want to sort strings in a case-insensitive manner, you can convert the strings to lowercase or uppercase in the compare function. Here’s an example:let array = ['John', 'jane', 'Oliver'];
array.sort(function(a, b) {
return a.toLowerCase().localeCompare(b.toLowerCase());
});
console.log(array); // Output: ['jane', 'John', 'Oliver']
In this example, the array is sorted in a case-insensitive manner.
How can I sort an array in numerical order instead of lexicographical order?
By default, the sort() function sorts numbers as strings, which results in a lexicographical order. To sort an array in numerical order, you can pass a compare function to the sort() function. The compare function should return the difference between two numbers. Here’s an example:let array = [10, 2, 30, 4];
array.sort(function(a, b) {
return a - b;
});
console.log(array); // Output: [2, 4, 10, 30]
In this example, the array is sorted in numerical order.
Can I use the sort() function to sort an array of dates?
Yes, you can use the sort() function to sort an array of Date objects. The sort() function can compare Date objects because they are converted to milliseconds since the Unix Epoch when compared. Here’s an example:let array = [
new Date(2021, 11, 1),
new Date(2021, 10, 1),
new Date(2021, 9, 1)
];
array.sort(function(a, b) {
return a - b;
});
console.log(array);
In this example, the array is sorted in ascending order of dates.
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
Published in
·CMS & Frameworks·Development Environment·Miscellaneous·Patterns & Practices·PHP·September 29, 2014
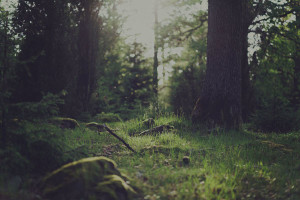
Published in
·CSS·Design·Design & UX·HTML & CSS·Resources·Typography·UI Design·Web Fonts·June 9, 2016