How to Use PHP Namespaces, Part 2: Importing, Aliases, and Name Resolution
Key Takeaways
- PHP namespaces can be imported using the ‘use’ operator, allowing for simplified code referencing. Multiple namespaces can be imported at once, separated by a comma, and PHP will search through these imported namespaces until it finds a match.
- Namespace aliases in PHP provide a way to reference longer namespaces with a shorter name, making the code cleaner and more readable. Aliases can be defined for classes within a namespace, but not for constants or functions.
- PHP resolves identifier names according to certain rules. Fully-qualified functions, classes or constants are resolved at compile-time, while unqualified names are translated according to import rules. Inside a namespace, all qualified names not already translated have the current namespace prepended. Unqualified function calls within a namespace are resolved at run-time.
In part 1, we discussed why PHP namespaces are useful and the
namespace
keyword. In this article, we examine the use
command and the way PHP resolves namespace names.
For the purposes of this example, we will define two almost identical code blocks; the only difference is their namespace:
lib1.php:
<?php
// application library 1
namespace App\Lib1;
const MYCONST = 'App\Lib1\MYCONST';
function MyFunction() {
return __FUNCTION__;
}
class MyClass {
static function WhoAmI() {
return __METHOD__;
}
}
?>
lib2.php:
<?php
// application library 2
namespace App\Lib2;
const MYCONST = 'App\Lib2\MYCONST';
function MyFunction() {
return __FUNCTION__;
}
class MyClass {
static function WhoAmI() {
return __METHOD__;
}
}
?>
There is a little PHP terminology to understand before we begin…
Fully-qualified name
Any PHP code can refer to a fully-qualified name — an identifier starting with the namespace backslash separator, e.g. AppLib1MYCONST, AppLib2MyFunction(), etc.
Fully-qualified names have no ambiguity. The initial backslash operates in a similar way to a file path; it signifies the ‘root’ global space. If we implemented a different MyFunction() in our global space, it could be called from lib1.php or lib2.php using MyFunction()
.
Fully-qualified names are useful for one-off function calls or object initialization. However, they can become impractical when you are making lots of calls. As we will discover below, PHP offers other options to save us from namespace typing cramps.
Qualified name
An identifier with at least one namespace separator, e.g. Lib1MyFunction().
Unqualified name
An identifier without a namespace separator, e.g. MyFunction().
Working Within the Same Namespace
Consider the following code:
myapp1.php:
<?php
namespace App\Lib1;
require_once('lib1.php');
require_once('lib2.php');
header('Content-type: text/plain');
echo MYCONST . "\n";
echo MyFunction() . "\n";
echo MyClass::WhoAmI() . "\n";
?>
Although we include both lib1.php and lib2.php, the identifiers MYCONST, MyFunction, and MyClass will only reference code in lib1.php. This occurs because the myapp1.php code is within the same AppLib1 namespace:
result:
App\Lib1\MYCONST
App\Lib1\MyFunction
App\Lib1\MyClass::WhoAmI
Namespace Importing
Namespaces can be imported with the use
operator, e.g.
myapp2.php:
<?php
use App\Lib2;
require_once('lib1.php');
require_once('lib2.php');
header('Content-type: text/plain');
echo Lib2\MYCONST . "\n";
echo Lib2\MyFunction() . "\n";
echo Lib2\MyClass::WhoAmI() . "\n";
?>
Any number of use
statements can be defined or you can separate individual namespaces with a comma. In this example we have imported the AppLib2 namespace. We still cannot refer directly to MYCONST, MyFunction or MyClass because our code is in the global space and PHP will look for them there. However, if we add a prefix of ‘Lib2’, they become qualified names; PHP will search through the imported namespaces until it finds a match.
result:
App\Lib1\MYCONST
App\Lib2\MyFunction
App\Lib2\MyClass::WhoAmI
Namespace Aliases
Namespace aliases are perhaps the most useful construct. Aliases allow us to reference long namespaces using a shorter name.
myapp3.php:
<?php
use App\Lib1 as L;
use App\Lib2\MyClass as Obj;
header('Content-type: text/plain');
require_once('lib1.php');
require_once('lib2.php');
echo L\MYCONST . "\n";
echo L\MyFunction() . "\n";
echo L\MyClass::WhoAmI() . "\n";
echo Obj::WhoAmI() . "\n";
?>
The first use
statement defines AppLib1 as ‘L’. Any qualified names using ‘L’ will be translated to ‘AppLib1’ at compile-time. We can therefore refer to LMYCONST and LMyFunction rather than the fully-qualified name.
The second use
statement is more interesting. It defines ‘Obj’ as an alias for the class ‘MyClass’ within the AppLib2 namespace. This is only possible for classes — not constants or functions. We can now use new Obj()
or run static methods as shown above.
result:
App\Lib1\MYCONST
App\Lib1\MyFunction
App\Lib1\MyClass::WhoAmI
App\Lib2\MyClass::WhoAmI
PHP Name Resolution Rules
PHP identifier names are resolved using the following namespace rules. Refer to the PHP manual for more information.
1. Calls to fully-qualified functions, classes or constants are resolved at compile-time.
2. Unqualified and qualified names are translated according to the import rules, e.g. if the namespace ABC is imported as C, a call to CDe()
is translated to ABCDe()
.
3. Inside a namespace, all qualified names not already translated according to import rules have the current namespace prepended, e.g. if a call to CDe()
is performed within namespace AB, it is translated to ABCDe()
.
4. Unqualified class names are translated according to current import rules and the full name is substituted for short imported name, e.g. if class C in namespace AB is imported as X, new X()
is translated to new ABC()
.
5. Unqualified function calls within a namespace are resolved at run-time. For example, if MyFunction() is called within namespace AB, PHP first looks for the function ABMyFunction(). If that is not found, it looks for MyFunction() in the global space.
6. Calls to unqualified or qualified class names are resolved at run-time. For example, if we call new C()
within namespace AB, PHP will look for the class ABC. If that is not found, it will attempt to autoload ABC.
See also:
- How to Use PHP Namespaces, Part 1: The Basics
- How to Use PHP Namespaces, Part 3: Keywords and Autoloading
- How to Install PHP 5.3 on Windows
In tomorrow’s final article we will cover autoloading and other advanced options.
Frequently Asked Questions (FAQs) about PHP Namespaces, Import, and Alias Resolution
What is the main purpose of using namespaces in PHP?
Namespaces in PHP serve as a way to encapsulate items. This can be seen as an abstract concept in many places. For example, in any operating system, directories serve to group related files, and PHP namespaces are similar. They are designed to solve two problems that authors of libraries and applications encounter: name collisions between code from different sources and the ability to alias or shorten long names for convenience.
How do I use the ‘use’ keyword in PHP?
The ‘use’ keyword in PHP is used to import classes, interfaces, functions, and constants from one namespace into another. It’s a way to make your code cleaner and more readable. For example, if you have a class ‘Foo’ in the namespace ‘Bar’, you can use it in another namespace by writing ‘use Bar\Foo;’. Now, you can create an instance of ‘Foo’ without having to write the full namespace.
What is the difference between ‘use’ and ‘require_once’ in PHP?
use’ and ‘require_once’ are both used to include code from other files in PHP, but they work in different ways. ‘use’ is used to import classes, interfaces, functions, and constants from one namespace to another, while ‘require_once’ is used to include a PHP file into another PHP file. If the file specified in ‘require_once’ is not found, it will cause a fatal error and halt the script execution.
How do I import multiple classes from the same namespace in PHP?
You can import multiple classes from the same namespace in PHP by using the ‘use’ keyword followed by the namespace and the classes separated by commas. For example, ‘use MyNamespace{ClassA, ClassB, ClassC};’. This will import ClassA, ClassB, and ClassC from the namespace ‘MyNamespace’.
What is aliasing in PHP namespaces?
Aliasing in PHP namespaces is a way to shorten the name of a class, interface, function, or constant that you’re importing from another namespace. This can make your code cleaner and more readable. For example, if you have a class ‘Foo’ in the namespace ‘Bar’, you can import it with an alias by writing ‘use Bar\Foo as Baz;’. Now, you can create an instance of ‘Foo’ by referring to it as ‘Baz’.
Can I use namespaces in PHP without the ‘use’ keyword?
Yes, you can use namespaces in PHP without the ‘use’ keyword by referring to the fully qualified name of the class, interface, function, or constant. However, this can make your code less readable, especially if you’re dealing with long namespaces. The ‘use’ keyword is recommended for importing items from other namespaces for cleaner and more readable code.
How do I resolve namespace conflicts in PHP?
Namespace conflicts in PHP can be resolved by using aliases. If two classes, interfaces, functions, or constants from different namespaces have the same name, you can import one of them with an alias to avoid a name collision. For example, if you have two ‘Foo’ classes in the ‘Bar’ and ‘Baz’ namespaces, you can import one of them with an alias by writing ‘use Bar\Foo as FooBar;’.
Can I use the same alias for different classes in PHP?
No, you cannot use the same alias for different classes in PHP. Aliases are meant to provide unique, short names for classes, interfaces, functions, or constants that you’re importing from other namespaces. If you try to use the same alias for different classes, PHP will throw a fatal error.
How do I use global namespace aliases in PHP?
You can use global namespace aliases in PHP by prefixing the name of the class, interface, function, or constant with a backslash (”). This tells PHP to look for the item in the global namespace, not in the current namespace. For example, if you’re in the ‘Foo’ namespace and want to use the global ‘Bar’ class, you can write ‘\Bar’.
Can I use namespaces in PHP 5?
Yes, you can use namespaces in PHP 5. The namespace feature was introduced in PHP 5.3.0. However, the syntax and features of namespaces have been improved in later versions of PHP, so it’s recommended to use the latest version of PHP for the best support of namespaces.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.
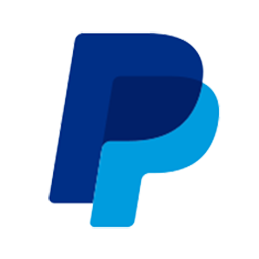
Published in
·APIs·Authentication·Debugging & Deployment·Libraries·Miscellaneous·PHP·Web Services·August 19, 2016