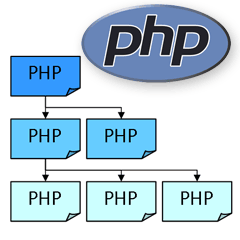
Why Do We Need Namespaces?
As the size of your PHP code library increases, the more likely you will accidentally reuse a function or class name that has been declared before. The problem is exacerbated if you attempt to add third-party components or plugins; what if two or more code sets implement a ‘Database’ class? Until now, the only solution has been long class/function names. For example, WordPress prefixes every name with ‘WP_’. The Zend Framework uses a highly descriptive naming convention that resullts in long-winded class names such as Zend_Search_Lucene_Analysis_Analyzer_Common_Text_CaseInsensitive. Name collision problems can be solved with namespaces. PHP constants, classes, and functions can be grouped into namespaced libraries.How are Namespaces Defined?
By default, all constant, class and function names are placed in a global space — like they were before namespaces were supported. Namespaced code is defined using a singlenamespace
keyword at the top of your PHP file. It must be the first command (with the exception of declare
) and no non-PHP code or white-space can precede the command, e.g.
<?php
// define this code in the MyProject namespace
namespace MyProject;
The code following this line will belong to the MyProject namespace. It is not possible to nest namespaces or define two or more namespaces for the same code block (only the last will be recognized). However, you can define different namespaced code in the same file, e.g.
<?php
namespace MyProject1;
// PHP code for the MyProject1 namespace
namespace MyProject2;
// PHP code for the MyProject2 namespace
// Alternative syntax
namespace MyProject3 {
// PHP code for the MyProject3 namespace
}
?>
However, I would strongly advise defining a single namespace per file.
Sub-namespaces
PHP allows you to define a hierarchy of namespace names so libraries can be sub-divided. Sub-namespaces are separated using a backslash (\) character, e.g.
- MyProject\SubName
- MyProject\Database\MySQL
- CompanyName\MyProject\Common\Widget
Calling Namespaced Code
In a file named lib1.php, we will define a constant, function, and class with the App\Lib1 namespace: lib1.php
<?php
// application library 1
namespace App\Lib1;
const MYCONST = 'App\Lib1\MYCONST';
function MyFunction() {
return __FUNCTION__;
}
class MyClass {
static function WhoAmI() {
return __METHOD__;
}
}
?>
To call this code, we can use PHP code such as:
myapp.php
<?php
header('Content-type: text/plain');
require_once('lib1.php');
echo \App\Lib1\MYCONST . "\n";
echo \App\Lib1\MyFunction() . "\n";
echo \App\Lib1\MyClass::WhoAmI() . "\n";
?>
No namespace is defined for myapp.php so the code exists in the global space. Any direct reference to MYCONST, MyFunction() or MyClass will fail because they exist in the App\Lib1 namespace. We must therefore add a prefix of \App\Lib1 to create a fully-qualified name. The following result is output when we load myapp.php:
App\Lib1\MYCONST
App\Lib1\MyFunction
App\Lib1\MyClass::WhoAmI
Fully-qualified names can obviously become quite long and there are few benefits over defining a class name of App-Lib1-MyClass. However, in the next article, we will discuss aliasing and take a closer look at how PHP resolves namespace names.
See also:
- How to Use PHP Namespaces, Part 2: Importing, Aliases, and Name Resolution
- How to Use PHP Namespaces, Part 3: Keywords and Autoloading
- How to Install PHP 5.3 on Windows
Frequently Asked Questions (FAQs) about PHP 5.3 Namespaces Basics
What is the main purpose of using namespaces in PHP?
Namespaces in PHP serve as a way to encapsulate items. This can be seen as an abstract concept in many places. For example, in any operating system, directories serve to group related files, and PHP namespaces are similar. They are designed to solve two problems that authors of libraries and applications encounter: name collisions between code from different sources and the ability to alias (or shorten) Extra_Long_Names designed to alleviate the first problem.
How do I declare a namespace in PHP?
Declaring a namespace in PHP is quite straightforward. You use the namespace keyword at the top of the PHP file before any other code – except declare statements. The syntax is as follows:namespace MyNamespace;
This code creates a namespace called MyNamespace. You can then add classes, functions, and constants to this namespace.
Can I use multiple namespaces in a single PHP file?
Yes, you can use multiple namespaces in a single PHP file. This is done by using the namespace keyword again. However, it’s important to note that PHP does not support nested namespaces, meaning you cannot declare a namespace inside another namespace.
How do I access elements from another namespace?
To access elements from another namespace, you need to use the fully qualified name of the element. This includes the namespace and the element name, separated by a backslash (). For example, if you have a function named myFunction in a namespace named myNamespace, you would access it like this:\myNamespace\myFunction();
What is the use of the ‘use’ keyword in PHP namespaces?
The ‘use’ keyword in PHP namespaces is used to import a specific class, function, or constant from another namespace, so that it can be accessed with a shorter name. For example, if you have a class named MyClass in a namespace named MyNamespace, you can import it like this:use MyNamespace\MyClass;
After this, you can simply use MyClass instead of the fully qualified name.
Can I alias or shorten namespace names in PHP?
Yes, PHP allows you to alias or shorten namespace names. This is done using the ‘as’ keyword in conjunction with the ‘use’ keyword. For example, if you have a namespace named MyVeryLongNamespaceName, you can alias it like this:use MyVeryLongNamespaceName as ShortName;
After this, you can use ShortName instead of the fully qualified name.
What is the global space in PHP namespaces?
In the context of PHP namespaces, the global space is the space outside of all namespaces. It’s where all the built-in PHP classes, functions, and constants reside. You can access elements in the global space from within a namespace by prefixing the element name with a backslash ().
What is the
NAMESPACE magic constant in PHP?The NAMESPACE magic constant in PHP contains the name of the current namespace. It’s empty in the global space. This can be useful when you need to get the name of the current namespace programmatically.
Can I use namespaces with autoload in PHP?
Yes, you can use namespaces with autoload in PHP. Autoload is a feature that allows PHP to automatically load classes when they are needed, without having to include them manually. When using namespaces, the autoload function can use the namespace name to locate and load the appropriate file.
Are there any naming conventions for PHP namespaces?
While PHP does not enforce any specific naming conventions for namespaces, it’s common practice to use vendor names as the top-level namespace. This helps to avoid name collisions when using code from different vendors. The rest of the namespace is usually structured to reflect the directory structure of the code, using uppercase for directory and class names, and lowercase for function and constant names.
Craig is a freelance UK web consultant who built his first page for IE2.0 in 1995. Since that time he's been advocating standards, accessibility, and best-practice HTML5 techniques. He's created enterprise specifications, websites and online applications for companies and organisations including the UK Parliament, the European Parliament, the Department of Energy & Climate Change, Microsoft, and more. He's written more than 1,000 articles for SitePoint and you can find him @craigbuckler.