JavaScript code snippet to get the current date in the format dd/mm/yyyy. The month is formatted to prefix with a zero (ie “04”) for single month figures. Also, here is how you can get a future date using jQuery
var fullDate = new Date()
console.log(fullDate);
//Thu May 19 2011 17:25:38 GMT+1000 {}
//convert month to 2 digits
var twoDigitMonth = ((fullDate.getMonth().length+1) === 1)? (fullDate.getMonth()+1) : '0' + (fullDate.getMonth()+1);
var currentDate = fullDate.getDate() + "/" + twoDigitMonth + "/" + fullDate.getFullYear();
console.log(currentDate);
//19/05/2011
Note: the console.log() commands are just for use with firebug.
If the above code doesn’t work try this (thanks pnilesh):
var fullDate = new Date();console.log(fullDate);
var twoDigitMonth = fullDate.getMonth()+"";if(twoDigitMonth.length==1) twoDigitMonth="0" +twoDigitMonth;
var twoDigitDate = fullDate.getDate()+"";if(twoDigitDate.length==1) twoDigitDate="0" +twoDigitDate;
var currentDate = twoDigitDate + "/" + twoDigitMonth + "/" + fullDate.getFullYear();console.log(currentDate);
Frequently Asked Questions (FAQs) about jQuery and Today’s Date
How can I get the current date in jQuery?
jQuery doesn’t have a built-in method to get the current date. However, you can use JavaScript’s built-in Date object to get the current date. Here’s a simple example:var currentDate = new Date();
console.log(currentDate);
This will return the current date and time. If you want to format this date in the dd-mm-yyyy format, you can do so like this:var day = currentDate.getDate();
var month = currentDate.getMonth() + 1;
var year = currentDate.getFullYear();
var formattedDate = day + '-' + month + '-' + year;
console.log(formattedDate);
What is the jQuery.now() method?
The jQuery.now() method is a utility function that returns the number of milliseconds that have passed since the Unix Epoch (January 1, 1970 00:00:00 UTC). It’s essentially a wrapper for the JavaScript Date.now() method. Here’s how you can use it:var milliseconds = jQuery.now();
console.log(milliseconds);
How can I format the current date using jQuery?
As mentioned earlier, jQuery doesn’t have built-in date handling functions. However, you can use JavaScript’s Date object in combination with jQuery to format the current date. Here’s an example:var currentDate = new Date();
var day = ("0" + currentDate.getDate()).slice(-2);
var month = ("0" + (currentDate.getMonth() + 1)).slice(-2);
var year = currentDate.getFullYear();
var formattedDate = day + '-' + month + '-' + year;
console.log(formattedDate);
Can I get the current date and time using jQuery?
Yes, you can get the current date and time using jQuery, but it’s actually JavaScript’s Date object that does the work. Here’s how you can do it:var currentDate = new Date();
console.log(currentDate);
This will return the current date and time in the following format: Wed Sep 22 2021 10:30:15 GMT+0530 (Indian Standard Time)
How can I get the current day, month, and year separately using jQuery?
You can use JavaScript’s Date object to get the current day, month, and year separately. Here’s how you can do it:var currentDate = new Date();
var day = currentDate.getDate();
var month = currentDate.getMonth() + 1;
var year = currentDate.getFullYear();
console.log('Day: ' + day);
console.log('Month: ' + month);
console.log('Year: ' + year);
This will return the current day, month, and year separately.
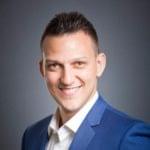
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.