I saw this function and thought I would share it as it could come in handy for dynamic generation of page titles (properly help with SEO if your titles are uniform and capitalized). The function was originally written by John Gruber but was ported over to jQuery by John Resig a couple of years ago.
Usage
var someVar = 'we love jquery';
console.log(upper(someVar));
//output: We love jquery
console.log(lower(someVar));
//output: we love jquery
console.log(titleCaps(someVar));
//output: We Love Jquery
The jQuery titleCaps Function
/**
* Title Caps
*
* Ported to JavaScript By John Resig - http://ejohn.org/ - 21 May 2008
* Original by John Gruber - http://daringfireball.net/ - 10 May 2008
* License: http://www.opensource.org/licenses/mit-license.php
*/
(function($)
{
var small = "(a|an|and|as|at|but|by|en|for|if|in|of|on|or|the|to|v[.]?|via|vs[.]?)";
var punct = "([!"#$%&'()*+,./:;< =>?@[\\\]^_`{|}~-]*)";
/**
* Apply title caps to the supplied string
* @param {String} title
* @returns {String}
*/
titleCaps = function(title)
{
var parts = [], split = /[:.;?!] |(?: |^)["Ã’]/g, index = 0;
while (true)
{
var m = split.exec(title);
parts.push( title.substring(index, m ? m.index : title.length)
.replace(/b([A-Za-z][a-z.'Õ]*)b/g, function(all)
{
return /[A-Za-z].[A-Za-z]/.test(all) ? all : upper(all);
})
.replace(RegExp("\b" + small + "\b", "ig"), lower)
.replace(RegExp("^" + punct + small + "\b", "ig"), function(all, punct, word)
{
return punct + upper(word);
})
.replace(RegExp("\b" + small + punct + "$", "ig"), upper));
index = split.lastIndex;
if(m) parts.push(m[0]);
else break;
}
return parts.join("").replace(/ V(s?). /ig, " v$1. ")
.replace(/(['Õ])Sb/ig, "$1s")
.replace(/b(AT&T|Q&A)b/ig, function(all){
return all.toUpperCase();
});
};
function lower(word)
{
return word.toLowerCase();
}
function upper(word)
{
return word.substr(0,1).toUpperCase() + word.substr(1);
}
//Examples
var someVar = 'we love jquery';
console.log(upper(someVar));
//output: We love jquery
console.log(lower(someVar));
//output: we love jquery
console.log(titleCaps(someVar));
//output: We Love Jquery
})(jQuery);
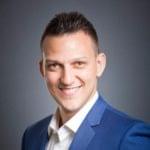
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.
jQuery
Share this article