jQuery code snippet to store data in a div for reference later. The data is not shown in the div but is stored against the element. Note that if the jQuery collection references multiple elements, the value returned refers to the first element. Here is how you do it.
//set the value to be stored
$("div").data("valuename", "hello");
//get the stored value
var value = $("div").data("valuename");
//outputs "hello"
alert(value);
//you can also change the value
$("div").data("valuename", 101);
//get the new stored value
var value = $("div").data("valuename");
//outputs "101"
alert(value);
You can use removeData(valuename) to remove the data item stored in the div.
Frequently Asked Questions about jQuery and Div Elements
How can I create a div element using jQuery?
Creating a div element using jQuery is quite simple. You can use the jQuery function $('<div></div>')
to create a new div element. This function creates a new jQuery object with the specified HTML content. You can then append this new div element to the body of your HTML document using the append()
function like so: $('body').append($('<div></div>'));
.
How can I add content to a div element using jQuery?
You can add content to a div element using the html()
or text()
functions in jQuery. The html()
function allows you to add HTML content, while the text()
function allows you to add text content. For example, if you have a div element with the id “myDiv”, you can add content to it like so: $('#myDiv').html('<p>This is some content</p>');
.
How can I store data in a div element using jQuery?
jQuery provides the data()
function to store and retrieve data associated with a DOM element. You can store data in a div element like so: $('#myDiv').data('key', 'value');
. You can then retrieve this data using the same data()
function like so: $('#myDiv').data('key');
.
How can I remove a div element using jQuery?
You can remove a div element using the remove()
function in jQuery. This function removes the selected element along with its child elements. For example, if you have a div element with the id “myDiv”, you can remove it like so: $('#myDiv').remove();
.
How can I hide and show a div element using jQuery?
jQuery provides the hide()
and show()
functions to hide and show elements. You can hide a div element like so: $('#myDiv').hide();
. You can then show this div element like so: $('#myDiv').show();
.
How can I change the CSS of a div element using jQuery?
You can change the CSS of a div element using the css()
function in jQuery. This function allows you to set one or more CSS properties for the selected element. For example, if you have a div element with the id “myDiv”, you can change its background color like so: $('#myDiv').css('background-color', 'red');
.
How can I animate a div element using jQuery?
jQuery provides the animate()
function to create custom animations. You can animate a div element like so: $('#myDiv').animate({left: '250px'});
. This will animate the div element to move 250 pixels to the right.
How can I add a class to a div element using jQuery?
You can add a class to a div element using the addClass()
function in jQuery. This function adds the specified class(es) to each element in the set of matched elements. For example, if you have a div element with the id “myDiv”, you can add a class to it like so: $('#myDiv').addClass('myClass');
.
How can I get the width and height of a div element using jQuery?
jQuery provides the width()
and height()
functions to get the current computed width and height of an element. You can get the width and height of a div element like so: $('#myDiv').width();
and $('#myDiv').height();
.
How can I bind an event to a div element using jQuery?
jQuery provides several functions to bind events to elements, such as click()
, dblclick()
, mouseenter()
, mouseleave()
, etc. You can bind an event to a div element like so: $('#myDiv').click(function() { alert('Div was clicked!'); });
. This will display an alert box when the div element is clicked.
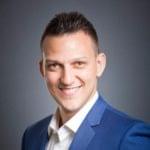
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.