Simple jQuery code snippet to replace all occurrences of characters (or strings) within a string. It could be used to find and replace all substrings found inside a string. Don’t forget to leave out the quotes on the first parameter (the g stands for global, you can also use i for an case insensitive search).
//global search in string
str = str.replace(/find/g,”replace”);
//OR global and case insensitive search in string
str = str.replace(/find/gi,”replace”);
global replace with dynamic var
//global replace
var regex = new RegExp('{'+fieldname+'}', "igm");
$itemForm = $itemForm.replace(regex, fieldvalue);
Also see: jQuery Read Text File Via AJAX.
Frequently Asked Questions about jQuery Replace String
What is the basic syntax for replacing a string in jQuery?
The basic syntax for replacing a string in jQuery is quite straightforward. You use the .replace() method, which is a built-in JavaScript method. The syntax is as follows: string.replace(searchValue, newValue). Here, ‘searchValue’ is the string that you want to replace and ‘newValue’ is the string that will replace the ‘searchValue’. It’s important to note that the .replace() method only replaces the first occurrence of the specified value.
How can I replace all occurrences of a string in jQuery?
To replace all occurrences of a string in jQuery, you can use a regular expression with the ‘g’ flag (global match). Here’s an example: var newString = oldString.replace(/oldValue/g, ‘newValue’); This will replace all instances of ‘oldValue’ with ‘newValue’ in the ‘oldString’.
Can I use jQuery to replace a string in HTML content?
Yes, you can use jQuery to replace a string in HTML content. You can use the .html() method in combination with the .replace() method. Here’s an example: $(‘#element’).html($(‘#element’).html().replace(/oldValue/g, ‘newValue’)); This will replace all instances of ‘oldValue’ with ‘newValue’ in the HTML content of the element with the ID ‘element’.
How can I replace a string in a specific part of my HTML document using jQuery?
To replace a string in a specific part of your HTML document, you can use jQuery selectors to target the specific element. Once you’ve targeted the element, you can use the .html() and .replace() methods as described in the previous question.
Can I use jQuery to replace a string in an attribute value?
Yes, you can use jQuery to replace a string in an attribute value. You can use the .attr() method in combination with the .replace() method. Here’s an example: $(‘#element’).attr(‘attribute’, $(‘#element’).attr(‘attribute’).replace(/oldValue/g, ‘newValue’)); This will replace all instances of ‘oldValue’ with ‘newValue’ in the value of the ‘attribute’ of the element with the ID ‘element’.
How can I replace a string in a URL using jQuery?
To replace a string in a URL using jQuery, you can use the .attr() method to get the URL from the href attribute of a link, and then use the .replace() method to replace the string. Here’s an example: $(‘a’).attr(‘href’, $(‘a’).attr(‘href’).replace(/oldValue/g, ‘newValue’)); This will replace all instances of ‘oldValue’ with ‘newValue’ in the URLs of all links.
Can I use jQuery to replace a string in a CSS property value?
Yes, you can use jQuery to replace a string in a CSS property value. You can use the .css() method in combination with the .replace() method. Here’s an example: $(‘#element’).css(‘property’, $(‘#element’).css(‘property’).replace(/oldValue/g, ‘newValue’)); This will replace all instances of ‘oldValue’ with ‘newValue’ in the value of the ‘property’ of the element with the ID ‘element’.
How can I replace a string in a JavaScript variable using jQuery?
To replace a string in a JavaScript variable, you don’t actually need jQuery. You can use the .replace() method of JavaScript. Here’s an example: var newVar = oldVar.replace(/oldValue/g, ‘newValue’); This will replace all instances of ‘oldValue’ with ‘newValue’ in the ‘oldVar’ variable.
Can I use jQuery to replace a string in a form input value?
Yes, you can use jQuery to replace a string in a form input value. You can use the .val() method in combination with the .replace() method. Here’s an example: $(‘#input’).val($(‘#input’).val().replace(/oldValue/g, ‘newValue’)); This will replace all instances of ‘oldValue’ with ‘newValue’ in the value of the input field with the ID ‘input’.
Can I use jQuery to replace a string in a text node?
Yes, you can use jQuery to replace a string in a text node. You can use the .contents() and .filter() methods to get the text nodes, and then use the .replaceWith() method to replace the string. Here’s an example: $(‘#element’).contents().filter(function() { return this.nodeType === 3; }).replaceWith(function() { return this.nodeValue.replace(/oldValue/g, ‘newValue’); }); This will replace all instances of ‘oldValue’ with ‘newValue’ in the text nodes of the element with the ID ‘element’.
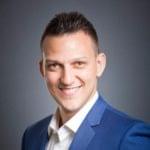
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.