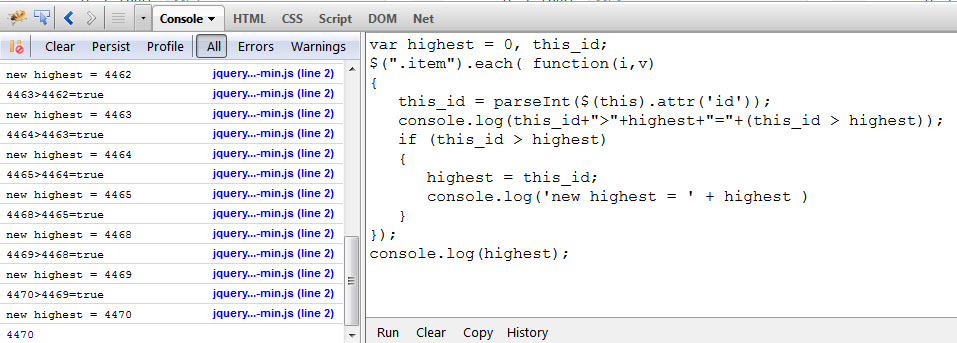
Example DOM Structure
...
...
...
...
The Code
//filtered by class, but you could loop all elements
var highest = 0, this_id;
$(".item").each( function(i,v)
{
this_id = parseInt($(this).attr('id'));
console.log(this_id+">"+highest+"="+(this_id > highest));
if (this_id > highest)
{
highest = this_id;
console.log('new highest = ' + highest )
}
});
console.log(highest);
Frequently Asked Questions (FAQs) about jQuery Highest ID Elements
How can I select an element with the highest ID using jQuery?
To select an element with the highest ID using jQuery, you can use the each()
function to iterate over all elements and compare their IDs. You can store the highest ID found in a variable and update it whenever you find a higher one. Here’s a simple example:var highestId = 0;
$('*[id]').each(function() {
var currentId = parseInt(this.id, 10);
if(currentId > highestId) {
highestId = currentId;
}
});
In this code, $('*[id]')
selects all elements with an ID, and each()
iterates over them. The ID of each element is parsed to an integer and compared to the current highest ID. If it’s higher, highestId
is updated.
What does the $('*[id]')
selector do in jQuery?
The $('*[id]')
selector in jQuery is used to select all elements that have an ID attribute. The *
character is a wildcard that matches any element, and [id]
specifies that the element must have an ID attribute. This selector is useful when you want to perform an operation on all elements that have an ID, such as finding the highest ID.
How can I select an element by its ID in jQuery?
To select an element by its ID in jQuery, you can use the $('#id')
selector, where ‘id’ is the ID of the element. For example, if you have an element with the ID ‘myElement’, you can select it with $('#myElement')
. This will return a jQuery object that you can use to manipulate the element.
Can I use the find()
function to select elements by ID in jQuery?
Yes, you can use the find()
function to select elements by ID in jQuery. However, find()
is typically used to find descendants of a selected element, not to select elements by their ID. To select an element by its ID, it’s more common to use the $('#id')
selector.
How can I select multiple elements by their IDs in jQuery?
To select multiple elements by their IDs in jQuery, you can use the $('#id1, #id2, #id3')
selector, where ‘id1’, ‘id2’, and ‘id3’ are the IDs of the elements. This will return a jQuery object that contains all the selected elements, which you can then manipulate as a group.
How can I get the ID of an element in jQuery?
To get the ID of an element in jQuery, you can use the attr()
function with ‘id’ as the argument. For example, if you have a jQuery object myElement
, you can get its ID with myElement.attr('id')
. This will return the ID of the element as a string.
Can I use jQuery to set the ID of an element?
Yes, you can use jQuery to set the ID of an element. You can use the attr()
function with two arguments: ‘id’ and the new ID. For example, if you have a jQuery object myElement
, you can set its ID with myElement.attr('id', 'newId')
.
How can I remove the ID of an element in jQuery?
To remove the ID of an element in jQuery, you can use the removeAttr()
function with ‘id’ as the argument. For example, if you have a jQuery object myElement
, you can remove its ID with myElement.removeAttr('id')
.
Can I use jQuery to select elements by their ID in a specific part of the page?
Yes, you can use jQuery to select elements by their ID in a specific part of the page. You can first select the part of the page with a selector, and then use the find()
function to find the elements by their ID. For example, if you want to select elements with the ID ‘myElement’ in a div with the class ‘myDiv’, you can use $('.myDiv').find('#myElement')
.
Can I use jQuery to select elements by their ID in a specific form?
Yes, you can use jQuery to select elements by their ID in a specific form. You can first select the form with a selector, and then use the find()
function to find the elements by their ID. For example, if you want to select elements with the ID ‘myElement’ in a form with the ID ‘myForm’, you can use $('#myForm').find('#myElement')
.
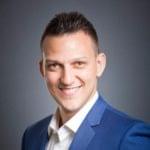
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.