Simple jQuery code snippet to get the highest value in array for specific index value. The Dataset.
var data = Array();
data[0] = {"apples":1, "pears":2, "oranges":3};
data[1] = {"apples":3, "pears":3, "oranges":5};
data[2] = {"apples":4, "pears":1, "oranges":6};
The function.
//get the highest value in array for specific index
//usage: getHighestVal(data,index)
//data = array
//index = index of array to analyse
function getHighestVal(data, index)
{
$.each(data, function (i,v)
{
thisVal = v[index];
max = (max < thisVal) ? thisVal : max;
});
return max;
}
var highest = getHighestVal(data, 'apples');
console.log(highest);
The Example:
So in this example, we want to get the highest value of “apple”.

Frequently Asked Questions (FAQs) about jQuery and Array Indexing
How can I find the maximum value in an array using jQuery?
jQuery does not have a built-in function to find the maximum value in an array. However, you can use JavaScript’s Math.max function in combination with the apply method to achieve this. Here’s how you can do it:var array = [1, 2, 3, 4, 5];
var max = Math.max.apply(null, array);
console.log(max); // Outputs: 5
In this code, Math.max.apply(null, array) is used to find the maximum value in the array. The apply method is used to call the Math.max function with an array of arguments.
How can I find the minimum value in an array using jQuery?
Similar to finding the maximum value, jQuery does not have a built-in function to find the minimum value in an array. However, you can use JavaScript’s Math.min function in combination with the apply method. Here’s an example:var array = [1, 2, 3, 4, 5];
var min = Math.min.apply(null, array);
console.log(min); // Outputs: 1
In this code, Math.min.apply(null, array) is used to find the minimum value in the array.
How can I find the index of the maximum value in an array using jQuery?
jQuery does not provide a built-in function to find the index of the maximum value in an array. However, you can use JavaScript’s reduce function to achieve this. Here’s how:var array = [1, 2, 3, 4, 5];
var maxIndex = array.reduce((maxIndex, value, index, array) => value > array[maxIndex] ? index : maxIndex, 0);
console.log(maxIndex); // Outputs: 4
In this code, the reduce function is used to iterate over the array and find the index of the maximum value.
How can I find the index of the minimum value in an array using jQuery?
Similar to finding the index of the maximum value, jQuery does not provide a built-in function to find the index of the minimum value in an array. However, you can use JavaScript’s reduce function. Here’s an example:var array = [1, 2, 3, 4, 5];
var minIndex = array.reduce((minIndex, value, index, array) => value < array[minIndex] ? index : minIndex, 0);
console.log(minIndex); // Outputs: 0
In this code, the reduce function is used to iterate over the array and find the index of the minimum value.
How can I sort an array in ascending order using jQuery?
jQuery does not provide a built-in function to sort an array in ascending order. However, you can use JavaScript’s sort function. Here’s how:var array = [5, 4, 3, 2, 1];
array.sort((a, b) => a - b);
console.log(array); // Outputs: [1, 2, 3, 4, 5]
In this code, the sort function is used to sort the array in ascending order.
How can I sort an array in descending order using jQuery?
Similar to sorting an array in ascending order, jQuery does not provide a built-in function to sort an array in descending order. However, you can use JavaScript’s sort function. Here’s an example:var array = [1, 2, 3, 4, 5];
array.sort((a, b) => b - a);
console.log(array); // Outputs: [5, 4, 3, 2, 1]
In this code, the sort function is used to sort the array in descending order.
How can I find the sum of all values in an array using jQuery?
jQuery does not provide a built-in function to find the sum of all values in an array. However, you can use JavaScript’s reduce function. Here’s how:var array = [1, 2, 3, 4, 5];
var sum = array.reduce((sum, value) => sum + value, 0);
console.log(sum); // Outputs: 15
In this code, the reduce function is used to iterate over the array and find the sum of all values.
How can I find the average of all values in an array using jQuery?
jQuery does not provide a built-in function to find the average of all values in an array. However, you can use JavaScript’s reduce function in combination with the length property. Here’s an example:var array = [1, 2, 3, 4, 5];
var average = array.reduce((sum, value) => sum + value, 0) / array.length;
console.log(average); // Outputs: 3
In this code, the reduce function is used to find the sum of all values, and then the sum is divided by the length of the array to find the average.
How can I remove duplicates from an array using jQuery?
jQuery provides a unique function that can be used to remove duplicates from an array. Here’s how:var array = [1, 2, 2, 3, 3, 4, 4, 5, 5];
var uniqueArray = $.unique(array);
console.log(uniqueArray); // Outputs: [1, 2, 3, 4, 5]
In this code, the unique function is used to remove duplicates from the array.
How can I check if an array contains a specific value using jQuery?
jQuery provides an inArray function that can be used to check if an array contains a specific value. Here’s an example:var array = [1, 2, 3, 4, 5];
var index = $.inArray(3, array);
console.log(index); // Outputs: 2
In this code, the inArray function is used to find the index of the value 3 in the array. If the value is not found, the function returns -1.
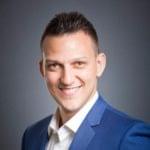
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.