jQuery code snippet to Find and Replace Characters using a loop of every html element in a web page. Change the values in the replace function to suit your needs.
jQuery('html').each(function(i){ jQuery(this).text(jQuery(this).text().replace('replacetext','withthistext'))})
Frequently Asked Questions (FAQs) about jQuery Find and Replace Characters Loop
How can I use jQuery to replace a specific character in a string?
To replace a specific character in a string using jQuery, you can use the replace() method. This method searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced. Here’s a simple example:var str = "Hello World!";
var res = str.replace("World", "jQuery");
In this example, the word “World” is replaced with “jQuery”. The replace() method only replaces the first occurrence of the specified value. To replace all occurrences, you would need to use a regular expression with a “g” flag (global match).
What is the difference between replaceWith() and replaceAll() in jQuery?
The replaceWith() and replaceAll() methods in jQuery are used to replace matched elements, but they work in slightly different ways. The replaceWith() method replaces the selected elements with new content, while the replaceAll() method replaces the elements matched by the specified selector.
For example, if you have two elements with ids “old” and “new”, you can replace “old” with “new” using replaceWith() like this:$("#old").replaceWith($("#new"));
On the other hand, to replace “new” with “old” using replaceAll(), you would do:$("#new").replaceAll("#old");
How can I use jQuery to find and replace HTML text?
To find and replace HTML text using jQuery, you can use the html() method in combination with the replace() method. The html() method sets or returns the content (innerHTML) of selected elements. When used to set content, it overwrites the content of ALL matched elements.
Here’s an example:$("p").html(function(index, oldHtml){
return oldHtml.replace("old text", "new text");
});
In this example, the function takes two arguments: the index of the current element in the list of selected elements, and the old HTML content of the element. The function returns the new HTML content, replacing “old text” with “new text”.
Can I use jQuery to replace multiple different characters in a string?
Yes, you can use jQuery to replace multiple different characters in a string. You can do this by chaining replace() methods, or by using a regular expression. Here’s an example using chained replace() methods:var str = "Hello World!";
var res = str.replace("Hello", "Hi").replace("World", "jQuery");
In this example, the words “Hello” and “World” are replaced with “Hi” and “jQuery”, respectively.
How can I use a loop to find and replace characters in a string using jQuery?
To use a loop to find and replace characters in a string using jQuery, you can use the each() method in combination with the replace() method. The each() method specifies a function to run for each matched element. Here’s an example:var replacements = {"Hello": "Hi", "World": "jQuery"};
$.each(replacements, function(oldStr, newStr) {
str = str.replace(new RegExp(oldStr, 'g'), newStr);
});
In this example, the each() method loops through each key-value pair in the replacements object, and the replace() method replaces each key (oldStr) with its corresponding value (newStr) in the string. The ‘g’ flag in the regular expression ensures that all occurrences of the key are replaced.
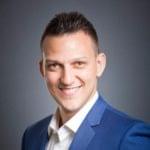
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.