Quick jQuery snippet to insert into string at a specific index (or a last string). For example, to find the last position of a table row.
//find last position of
var lastRowIndex = $itemForm.lastIndexOf('');
console.log(lastRowIndex);
You could then insert the string into the index of another string like so:
console.log($itemForm.substring(lastRowIndex, $itemForm.length));
$itemForm = $itemForm.substring(0,lastRowIndex) + "" + data.tableRowTemplate.html() + "" + $itemForm.substring(lastRowIndex, $itemForm.length);
console.log($itemForm);
Frequently Asked Questions (FAQs) about jQuery Find Index of String
How can I use jQuery to find the index of a string in an array?
jQuery doesn’t have a built-in method to find the index of a string in an array. However, you can use the JavaScript method indexOf()
. This method returns the first index at which a given element can be found in the array, or -1 if it is not present. Here’s an example:var array = ["apple", "banana", "cherry"];
var index = array.indexOf("banana");
console.log(index); // Outputs: 1
What is the difference between find()
and lastIndexOf()
in jQuery?
The find()
method in jQuery is used to get the descendants of each element in the current set of matched elements, filtered by a selector, jQuery object, or element. On the other hand, lastIndexOf()
is a JavaScript method that returns the last index at which a certain element can be found in the array, or -1 if the element is not found.
How can I use the lastIndexOf()
method in JavaScript?
The lastIndexOf()
method is used to find the position of the last occurrence of a specified value in a string. Here’s an example:var str = "Hello world, welcome to the universe.";
var n = str.lastIndexOf("e");
console.log(n); // Outputs: 36
Can I use the find()
method in jQuery to find the index of a string?
No, the find()
method in jQuery is used to get the descendants of each element in the current set of matched elements, filtered by a selector, jQuery object, or element. To find the index of a string, you can use the JavaScript method indexOf()
.
How can I find the last index of a string in an array using jQuery?
jQuery doesn’t have a built-in method to find the last index of a string in an array. However, you can use the JavaScript method lastIndexOf()
. This method returns the last index at which a given element can be found in the array, or -1 if it is not present. Here’s an example:var array = ["apple", "banana", "cherry", "banana"];
var index = array.lastIndexOf("banana");
console.log(index); // Outputs: 3
What is the difference between indexOf()
and lastIndexOf()
in JavaScript?
The indexOf()
method returns the first index at which a given element can be found in the array, while lastIndexOf()
returns the last index. Both methods return -1 if the element is not found.
Can I use jQuery to find the index of a string in a string?
No, jQuery doesn’t have a built-in method to find the index of a string in a string. However, you can use the JavaScript method indexOf()
. This method returns the first index at which a given element can be found in the string, or -1 if it is not present.
How can I use the find()
method in jQuery?
The find()
method in jQuery is used to get the descendants of each element in the current set of matched elements, filtered by a selector, jQuery object, or element. Here’s an example:$("div").find("p"); // Returns all <p> elements inside <div> elements
Can I use the lastIndexOf()
method in jQuery?
No, lastIndexOf()
is not a jQuery method. It is a JavaScript method that can be used to find the last index at which a given element can be found in the array or string.
How can I find the index of a string in a string using JavaScript?
You can use the indexOf()
method in JavaScript to find the index of a string in a string. Here’s an example:var str = "Hello world, welcome to the universe.";
var n = str.indexOf("welcome");
console.log(n); // Outputs: 13
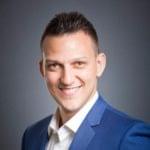
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.