Simple jQuery code snippets to stop input clearing on click by disabling the onclick event associated with a button. This is just down and dirty code sorry there is no proper examples.
$('.del').click(function(){
onclick = $(this).attr('onclick');
$(this).attr('onclick','');
showConfirm(onclick);
return false;
});
$('input').click(function(){
console.log("clicked");
onclick = $(this).attr('onclick');
$(this).attr('onclick','');
showConfirm(onclick);
return false;
});
$('input').each(function () {
this.onclick = undefined;
});
$('.foo').removeAttr('onclick').click(function(){
// Do something
});
var original_onclick = $('.del').attr('onclick');
$('.del').removeAttr('onclick').click(function(){
showConfirm(original_onclick);
});
Frequently Asked Questions (FAQs) about jQuery Disable Onclick Event
How can I disable the onclick event in jQuery?
Disabling the onclick event in jQuery can be achieved using the .off() method. This method removes event handlers that were attached with .on(). Here is a simple example:$("#element").off("click");
In this example, the click event handler for the element with the id “element” is removed. Now, when you click on this element, nothing will happen because the click event handler has been disabled.
Can I disable all events for an element in jQuery?
Yes, you can disable all events for a specific element in jQuery. You can do this by not passing any arguments to the .off() method. Here is an example:$("#element").off();
In this example, all event handlers for the element with the id “element” are removed.
Can I re-enable a disabled onclick event in jQuery?
Yes, you can re-enable a disabled onclick event in jQuery. You can do this by re-attaching the event handler using the .on() method. Here is an example:$("#element").on("click", function() {
// Your code here
});
In this example, a click event handler is attached to the element with the id “element”.
Can I disable the onclick event for a specific function only?
Yes, you can disable the onclick event for a specific function only. You can do this by passing the function as an argument to the .off() method. Here is an example:var myFunction = function() {
// Your code here
};
$("#element").off("click", myFunction);
In this example, the click event handler for the function myFunction is removed from the element with the id “element”.
Can I disable the onclick event for all elements of a certain type?
Yes, you can disable the onclick event for all elements of a certain type. You can do this by using the element type as the selector in the .off() method. Here is an example:$("button").off("click");
In this example, the click event handler is removed from all button elements.
Can I disable multiple events at once in jQuery?
Yes, you can disable multiple events at once in jQuery. You can do this by passing multiple event names, separated by spaces, to the .off() method. Here is an example:$("#element").off("click mouseover");
In this example, the click and mouseover event handlers are removed from the element with the id “element”.
Can I disable the onclick event for dynamically added elements?
Yes, you can disable the onclick event for dynamically added elements. You can do this by using the .on() method to attach an event handler to a static parent element, and specifying the dynamic elements as the selector. Here is an example:$("#parent").on("click", ".dynamic", function(event) {
event.stopImmediatePropagation();
});
In this example, the click event is disabled for all elements with the class “dynamic” that are children of the element with the id “parent”.
Can I disable the onclick event without using jQuery?
Yes, you can disable the onclick event without using jQuery. You can do this by using the removeEventListener method in JavaScript. Here is an example:document.getElementById("element").removeEventListener("click", myFunction);
In this example, the click event handler for the function myFunction is removed from the element with the id “element”.
Can I check if the onclick event is disabled for an element?
Unfortunately, there is no built-in method in jQuery or JavaScript to check if the onclick event is disabled for an element. However, you can keep track of this yourself by using a variable or a data attribute to store the state of the event handler.
Can I disable the onclick event for a certain period of time?
Yes, you can disable the onclick event for a certain period of time. You can do this by using the setTimeout function in JavaScript to re-enable the event handler after a certain period of time. Here is an example:$("#element").off("click");
setTimeout(function() {
$("#element").on("click", myFunction);
}, 5000);
In this example, the click event handler for the function myFunction is removed from the element with the id “element”, and then re-enabled after 5 seconds.
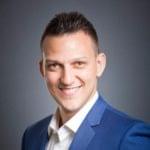
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.