Here is how you might get a a future date using jQuery. The future date is calculated based on the current date for example days later than the current date. If you are unsure, here is how you can get the current date.
Check if is future date using jQuery
function isFutureDate()
{
var date=new Date();
date.setFullYear(2020,1,1);
var _now=new Date();
if(date.getTime()>_now.getTime())
{
// date is future
}
}
Check if is a 4 digit year
This functions checks if the date has 2 digit days and months and 4 digit year.function twodigits(digits) {
return (digits > 9) ? digits : '0' + digits;
}
The full code
var fullDate = new Date();
var twoDigitDays = twodigits(fullDate.getDate());
var twoDigitMonth = twodigits(fullDate.getMonth() + 1);
var departDate = twoDigitDays + "/" + twoDigitMonth + "/" + fullDate.getFullYear();
console.log(departDate);
fullDate.setDate(fullDate.getDate()+7);
var twoDigitDays = twodigits(fullDate.getDate());
var twoDigitMonth = twodigits(fullDate.getMonth() + 1);
var returnDate = twoDigitDays + "/" + twoDigitMonth + "/" + fullDate.getFullYear();
console.log(returnDate);
Alternate Example
var currentDate = new Date(),
currentMonth = currentDate.getMonth() + 1,
lastDayOfMonth = new Date(currentDate.getFullYear(), (currentDate.getMonth() - 1), 0).getDate(),
departureDate = futureDateDays(14),
depDate = departureDate.split('/'),
departureDateMonth = depDate[1];
if (departureDateMonth != currentMonth) {
departureDate = leadingZero(currentMonth) + '/' + leadingZero(lastDayOfMonth) +'/'+ depDate[2];
}
console.log(departureDate);
//quick fix convert back to date using string format mm/dd/yyyy
var validDate = new Date(departureDate);
//then back to string
departureDate = leadingZero(validDate.getDate()) + '/' + leadingZero(validDate.getMonth()+1) +'/'+ validDate.getFullYear();
//Output:
//09/31/2011
//Date {Sat Oct 01 2011 00:00:00 GMT+1000}
//01/10/2011
Frequently Asked Questions (FAQs) about jQuery Date and Future Date
How can I use jQuery to check if a date is in the future?
To check if a date is in the future using jQuery, you can use the JavaScript Date object. First, create a new Date object for the current date and time. Then, create another Date object for the date you want to check. Compare these two dates using the getTime method, which returns the number of milliseconds since the Unix Epoch (January 1, 1970). If the future date’s time is greater than the current date’s time, then the date is in the future.
How can I set a date 10 days in the future using jQuery?
To set a date 10 days in the future, you can use the JavaScript Date object and its setDate method. First, create a new Date object for the current date and time. Then, use the getDate method to get the current day of the month, and add 10 to it. Use the setDate method to set the day of the month to this new value. The Date object will automatically adjust the month and year if necessary.
How can I format a future date to “dd-mm-yyyy” using jQuery?
To format a date to “dd-mm-yyyy” in jQuery, you can use the JavaScript Date object and its methods. First, create a new Date object for the date you want to format. Then, use the getDate, getMonth, and getFullYear methods to get the day, month, and year. Note that getMonth returns a zero-based value (0 for January, 1 for February, etc.), so you need to add 1 to it. Finally, concatenate these values with dashes in between.
How can I validate if a user-entered date is a future date using jQuery?
To validate if a user-entered date is a future date, you can use the JavaScript Date object and jQuery’s val method. First, use the val method to get the value of the input field where the user enters the date. Then, create a new Date object for this value and another Date object for the current date and time. Compare these two dates as described in the answer to the first question.
How can I use jQuery to check if a date is the current date or a future date?
To check if a date is the current date or a future date, you can use the JavaScript Date object and its toDateString method. First, create a new Date object for the current date and time, and another Date object for the date you want to check. Convert these dates to strings using the toDateString method, which returns a string in the format “weekday month day year”. If the strings are equal, then the date is the current date. If the future date’s time is greater than the current date’s time, then the date is in the future.
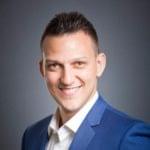
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.