Firstly, setTimeout() and clearTimeout() are pure JavaScript functions but are regularly used in JavaScript animations and the jQuery library. Sometimes when you have timeouts set up you may want to cancel them, in order to do this you need to store the settimeout in a variable.
Looping setTimeouts
Inside this loop the timeout is always going to be the same value: eg: TIMEOUT=1773for(i=0; i<10; i++)
{
newTimeout = setTimeout(function()
{
console.log('TIMEOUT='+newTimeout);
});
}
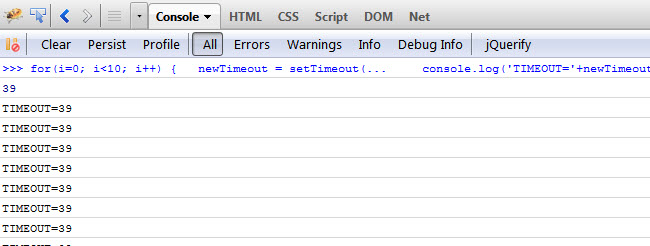
i = 0;
var interval = setInterval(function()
{
newTimeout = setTimeout(function()
{
console.log(this);
console.log('TIMEOUT='+newTimeout);
}, 100);
i++;
if (i > 10)
{
clearInterval(interval);
}
}, 200);
output:
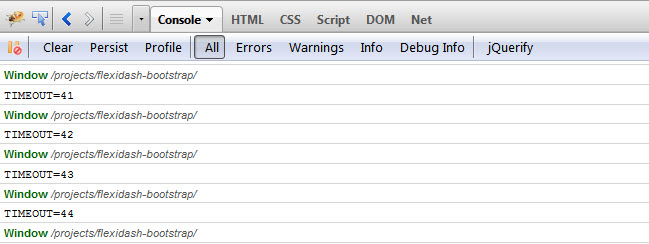
Clear all setTimeouts
for(i=0; i<100; i++)
{
window.clearTimeout(i);
}
Further Reading
I wonder if it is possible to use jQuery to store timeout on object as data??? So then if an animation is queued using a setTimeout it could be stopped if it’s currently in animation or even before it’s started animating? hmm …if (animateWidget !== undefined)
{
//only do 10 windows
for(i=0; i<10; i++)
{
window.clearTimeout(i);
}
//stop all animations on windows
$('.containerPlus').stop();
}
var animateWidget = setTimeout(function()
{
console.log('TIMEOUT: '+animateWidget);
EVI.FLEXIDASH.tileDash();
}, 300);
Frequently Asked Questions about jQuery clearTimeout()
What is the clearTimeout() function in jQuery?
The clearTimeout() function in jQuery is a built-in method that cancels a timeout previously established by calling setTimeout(). This function allows you to stop the execution of the function specified in setTimeout() before the set time period has elapsed. It is particularly useful in scenarios where you want to give the user the ability to stop certain actions from happening after a delay.
How do I use the clearTimeout() function in jQuery?
To use the clearTimeout() function, you first need to assign the setTimeout() function to a variable. This variable is then passed as an argument to the clearTimeout() function. Here’s a basic example:var myTimeout = setTimeout(function(){ alert("Hello"); }, 3000);
clearTimeout(myTimeout);
In this example, the alert box will not show up because the clearTimeout() function stops the setTimeout() function before it can execute.
Can I clear all timeouts at once in jQuery?
There’s no built-in function to clear all timeouts at once in jQuery. However, you can create an array to store all timeout IDs when you set them, and then loop through this array to clear all timeouts. Here’s an example:var timeouts = [];
timeouts.push(setTimeout(function(){ alert("Hello"); }, 3000));
timeouts.push(setTimeout(function(){ alert("World"); }, 5000));
for (var i=0; i<timeouts.length; i++) {
clearTimeout(timeouts[i]);
}
In this example, neither of the alert boxes will show up because all timeouts are cleared before they can execute.
What is the difference between clearTimeout() and clearInterval()?
Both clearTimeout() and clearInterval() are used to cancel timed events set by setTimeout() and setInterval() respectively. The main difference is that setTimeout() executes a function once after a specified delay, while setInterval() continues to execute the function repeatedly at the delay interval, until clearInterval() is called or the window is closed.
Can clearTimeout() stop a function that’s already running?
No, clearTimeout() cannot stop a function that’s already running. It can only prevent a function from starting if it was set to run in the future with setTimeout(). If the specified delay has already passed and the function is running, clearTimeout() will not have any effect.
Does clearTimeout() work with asynchronous functions?
Yes, clearTimeout() works with asynchronous functions. However, keep in mind that if the asynchronous function has already started executing, clearTimeout() will not stop it. It can only prevent the function from starting if it was set to run in the future with setTimeout().
What happens if I call clearTimeout() without an argument?
If you call clearTimeout() without an argument or with an invalid argument, the function will simply do nothing. It won’t throw an error, but it also won’t clear any timeouts.
Can I use clearTimeout() in a loop?
Yes, you can use clearTimeout() in a loop. This is often done when you have multiple timeouts that you want to clear at once. You can store the IDs of the timeouts in an array, and then use a loop to clear each one.
Does clearTimeout() work across different browsers?
Yes, clearTimeout() is a standard JavaScript function and is supported by all major browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer.
Can I use clearTimeout() in Node.js?
Yes, clearTimeout() is a global function in Node.js, just like in browser-based JavaScript. It works the same way, allowing you to cancel a timeout that was previously set with setTimeout().
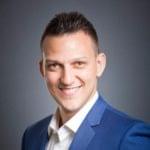
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.